用Python写一个有界面的五子棋游戏
时间: 2024-05-03 08:16:41 浏览: 73
好的,以下是一个简单的五子棋游戏的 Python 代码,使用了 tkinter 绘制界面:
```python
import tkinter as tk
class Gobang:
def __init__(self, master):
self.master = master
self.master.title("五子棋")
self.master.geometry("600x600")
self.master.resizable(False, False)
self.canvas = tk.Canvas(self.master, bg="#F5DEB3", width=600, height=600)
self.canvas.pack()
self.draw_board()
self.canvas.bind("<Button-1>", self.click)
self.player = "black"
self.chessboard = [[0 for i in range(15)] for j in range(15)]
def draw_board(self):
for i in range(15):
self.canvas.create_line(50 + i * 40, 50, 50 + i * 40, 570, width=2)
for i in range(15):
self.canvas.create_line(50, 50 + i * 40, 570, 50 + i * 40, width=2)
self.canvas.create_oval(245, 245, 255, 255, fill="black")
self.canvas.create_oval(335, 245, 345, 255, fill="black")
self.canvas.create_oval(245, 335, 255, 345, fill="black")
self.canvas.create_oval(335, 335, 345, 345, fill="black")
def click(self, event):
x = (event.x - 35) // 40
y = (event.y - 35) // 40
if self.chessboard[x][y] == 0:
if self.player == "black":
fill_color = "black"
self.chessboard[x][y] = 1
self.player = "white"
else:
fill_color = "white"
self.chessboard[x][y] = 2
self.player = "black"
self.canvas.create_oval(55 + x * 40, 55 + y * 40, 85 + x * 40, 85 + y * 40, fill=fill_color)
self.check_win(x, y)
def check_win(self, x, y):
color = self.chessboard[x][y]
count = 1
i = 1
while x + i < 15 and self.chessboard[x + i][y] == color:
count += 1
i += 1
i = 1
while x - i >= 0 and self.chessboard[x - i][y] == color:
count += 1
i += 1
if count >= 5:
self.game_over(color)
return
count = 1
i = 1
while y + i < 15 and self.chessboard[x][y + i] == color:
count += 1
i += 1
i = 1
while y - i >= 0 and self.chessboard[x][y - i] == color:
count += 1
i += 1
if count >= 5:
self.game_over(color)
return
count = 1
i = 1
while x + i < 15 and y + i < 15 and self.chessboard[x + i][y + i] == color:
count += 1
i += 1
i = 1
while x - i >= 0 and y - i >= 0 and self.chessboard[x - i][y - i] == color:
count += 1
i += 1
if count >= 5:
self.game_over(color)
return
count = 1
i = 1
while x + i < 15 and y - i >= 0 and self.chessboard[x + i][y - i] == color:
count += 1
i += 1
i = 1
while x - i >= 0 and y + i < 15 and self.chessboard[x - i][y + i] == color:
count += 1
i += 1
if count >= 5:
self.game_over(color)
return
def game_over(self, color):
if color == 1:
winner = "黑方"
else:
winner = "白方"
tk.messagebox.showinfo(title="游戏结束", message=winner + "胜利!")
self.canvas.unbind("<Button-1>")
self.master.destroy()
if __name__ == "__main__":
root = tk.Tk()
Gobang(root)
root.mainloop()
```
运行代码后,将会弹出一个五子棋游戏的窗口,您可以点击黑色或白色棋子进行下棋,当有一方获胜时,会弹出胜利提示框。
阅读全文
相关推荐
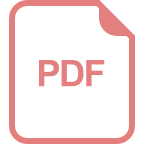
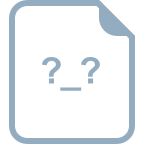
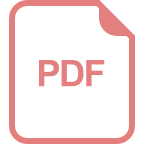












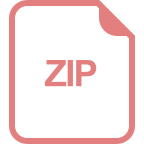
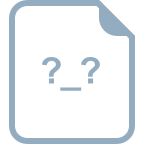
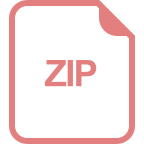
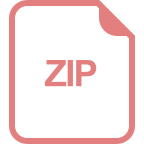