python从MySQL插入数据
时间: 2024-05-07 12:20:35 浏览: 10
在Python中使用MySQL数据库插入数据,可以使用MySQL官方提供的Python驱动程序MySQL Connector/Python或第三方库PyMySQL。
以下是使用MySQL Connector/Python的示例代码:
```python
import mysql.connector
# 连接数据库
mydb = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="database_name"
)
# 创建游标对象
mycursor = mydb.cursor()
# 插入数据
sql = "INSERT INTO customers (name, address) VALUES (%s, %s)"
val = ("John", "Highway 21")
mycursor.execute(sql, val)
# 提交更改
mydb.commit()
# 输出插入的数据行的ID
print("1 行数据插入成功,ID:", mycursor.lastrowid)
```
以下是使用PyMySQL的示例代码:
```python
import pymysql
# 连接数据库
mydb = pymysql.connect(
host="localhost",
user="username",
password="password",
database="database_name"
)
# 创建游标对象
mycursor = mydb.cursor()
# 插入数据
sql = "INSERT INTO customers (name, address) VALUES (%s, %s)"
val = ("John", "Highway 21")
mycursor.execute(sql, val)
# 提交更改
mydb.commit()
# 输出插入的数据行的ID
print("1 行数据插入成功,ID:", mycursor.lastrowid)
```
以上代码中,我们连接到MySQL数据库,创建游标对象,然后使用 `mycursor.execute()` 方法执行SQL语句插入数据,并使用 `mydb.commit()` 方法提交更改。最后,使用 `mycursor.lastrowid` 属性获取插入的数据行的ID。
相关推荐
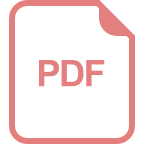
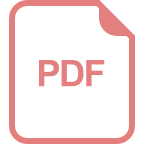
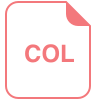
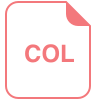
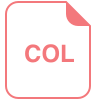
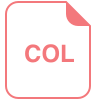
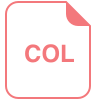









