vue购物车数量增加减少
时间: 2023-06-29 19:12:55 浏览: 53
要实现购物车数量的增加和减少,可以通过以下步骤实现:
1. 在 Vue 的 data 中添加一个数量的变量,比如叫做 `count`,并给它一个初始值为 1。
2. 在模板中显示这个数量变量,比如可以这样写:`<div>数量:{{ count }}</div>`
3. 添加两个按钮,一个用来增加数量,一个用来减少数量,比如可以这样写:
```
<button @click="count++">增加数量</button>
<button @click="count--">减少数量</button>
```
4. 如果希望数量不能小于 1,可以在减少数量的点击事件中添加一个判断,比如这样:
```
<button @click="if (count > 1) count--">减少数量</button>
```
这样就可以实现简单的购物车数量增加和减少功能了。如果需要更复杂的功能,比如限制库存数量、展示总价等,可以根据具体需求进行扩展。
相关问题
vue购物车添加商品数量
如下是一个简单的示例,演示如何实现Vue购物车添加商品数量:
HTML代码:
```
<div id="app">
<h1>购物车</h1>
<ul>
<li v-for="product in products">
{{ product.name }} - ¥{{ product.price }}
<button @click="addToCart(product)">添加到购物车</button>
<span v-if="cart.includes(product.id)">
数量:<button @click="decreaseQuantity(product)">-</button>{{ cartQuantity[product.id] }}
<button @click="increaseQuantity(product)">+</button>
</span>
</li>
</ul>
</div>
```
Vue代码:
```
new Vue({
el: '#app',
data: {
products: [
{ id: 1, name: '商品1', price: 100 },
{ id: 2, name: '商品2', price: 200 },
{ id: 3, name: '商品3', price: 300 }
],
cart: [], // 购物车中商品ID的列表
cartQuantity: {} // 购物车中每个商品的数量
},
methods: {
addToCart(product) {
if (!this.cart.includes(product.id)) {
this.cart.push(product.id);
this.cartQuantity[product.id] = 1;
} else {
this.cartQuantity[product.id]++;
}
},
increaseQuantity(product) {
this.cartQuantity[product.id]++;
},
decreaseQuantity(product) {
if (this.cartQuantity[product.id] > 1) {
this.cartQuantity[product.id]--;
} else {
this.cart.splice(this.cart.indexOf(product.id), 1);
delete this.cartQuantity[product.id];
}
}
}
})
```
在这个示例中,我们创建了一个Vue实例,其中包含了一个`products`数组,以及两个空数组`cart`和`cartQuantity`。然后我们定义了三个方法:`addToCart`、`increaseQuantity`和`decreaseQuantity`。
当用户点击“添加到购物车”按钮时,`addToCart`方法会将商品ID添加到`cart`列表中,并将该商品的数量设为1。如果用户再次添加同样的商品,则只是数量加1。
在购物车列表中,我们检查该商品的ID是否包含在`cart`数组中,如果包含,则显示该商品的数量,并提供增加和减少数量的按钮。`increaseQuantity`和`decreaseQuantity`方法用于增加或减少商品数量,并且在数量减少到0时会将该商品从购物车中移除。
springboot+vue购物车
基于Spring Boot和Vue的购物车实现可以分为以下几个步骤:
1. 创建商品列表页面,展示所有商品信息,包括商品名称、价格、图片等,并在每个商品后面添加“加入购物车”按钮。
2. 点击“加入购物车”按钮后,将该商品信息添加到购物车中。可以使用VueX来实现购物车的状态管理。
3. 创建购物车页面,展示当前购物车中的所有商品信息,包括商品名称、价格、数量、小计等,并在每个商品后面添加“删除”按钮和“增加/减少数量”按钮。
4. 点击“删除”按钮后,将该商品从购物车中删除。
5. 点击“增加/减少数量”按钮后,可以增加或减少该商品的数量,并更新小计金额。
6. 在购物车页面中添加“结算”按钮,点击后跳转到订单确认页面。
下面是一个简单的示例代码,仅供参考:
1. 商品列表页面
```vue
<template>
<div>
<div v-for="item in productList" :key="item.id">
<img :src="item.image" alt="">
<p>{{ item.name }}</p>
<p>{{ item.price }}</p>
<button @click="addToCart(item)">加入购物车</button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
productList: [
{
id: 1,
name: '商品1',
price: 100,
image: 'https://xxx.com/1.jpg'
},
{
id: 2,
name: '商品2',
price: 200,
image: 'https://xxx.com/2.jpg'
},
// 其他商品信息
]
}
},
methods: {
addToCart(item) {
this.$store.commit('addToCart', item)
}
}
}
</script>
```
2. 购物车页面
```vue
<template>
<div>
<div v-for="item in cartList" :key="item.id">
<img :src="item.image" alt="">
<p>{{ item.name }}</p>
<p>{{ item.price }}</p>
<button @click="decreaseQuantity(item)">-</button>
<span>{{ item.quantity }}</span>
<button @click="increaseQuantity(item)">+</button>
<p>{{ item.subtotal }}</p>
<button @click="removeFromCart(item)">删除</button>
</div>
<p>总计:{{ total }}</p>
<button @click="checkout">结算</button>
</div>
</template>
<script>
export default {
computed: {
cartList() {
return this.$store.state.cartList
},
total() {
let sum = 0
this.cartList.forEach(item => {
sum += item.subtotal
})
return sum
}
},
methods: {
removeFromCart(item) {
this.$store.commit('removeFromCart', item)
},
increaseQuantity(item) {
this.$store.commit('increaseQuantity', item)
},
decreaseQuantity(item) {
this.$store.commit('decreaseQuantity', item)
},
checkout() {
// 跳转到订单确认页面
}
}
}
</script>
```
3. VueX状态管理
```javascript
const store = new Vuex.Store({
state: {
cartList: []
},
mutations: {
addToCart(state, item) {
const index = state.cartList.findIndex(i => i.id === item.id)
if (index === -1) {
state.cartList.push({
...item,
quantity: 1,
subtotal: item.price
})
} else {
state.cartList[index].quantity++
state.cartList[index].subtotal += item.price
}
},
removeFromCart(state, item) {
const index = state.cartList.findIndex(i => i.id === item.id)
state.cartList.splice(index, 1)
},
increaseQuantity(state, item) {
const index = state.cartList.findIndex(i => i.id === item.id)
state.cartList[index].quantity++
state.cartList[index].subtotal += item.price
},
decreaseQuantity(state, item) {
const index = state.cartList.findIndex(i => i.id === item.id)
if (state.cartList[index].quantity > 1) {
state.cartList[index].quantity--
state.cartList[index].subtotal -= item.price
}
}
}
})
```
相关推荐
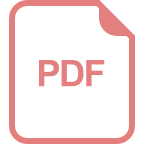
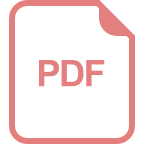












