考试界面从题库中随机抽取一题 题库: 题目:1+1等于?,选项:1,2,3,4 题目:3>2是?,选项:正确的,错误的 题目:2*3等于?,选项:2,4,6,8 点击提交按钮弹出确定提交对话框,如果点击确定,则弹出考试结果对话框(需要判断选项是否正确),如果再点击确定则关闭所有窗体。
时间: 2023-06-24 12:04:43 浏览: 59
好的,这个需求可以用简单的 HTML、CSS 和 JavaScript 实现。以下是一个简单的实现:
HTML:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>考试</title>
</head>
<body>
<h1>考试</h1>
<div id="question"></div>
<div id="options"></div>
<button id="submit">提交</button>
<script src="exam.js"></script>
</body>
</html>
```
CSS:
```css
body {
font-family: Arial, sans-serif;
}
h1 {
font-size: 2em;
margin-bottom: 0.5em;
}
#question {
font-size: 1.5em;
margin-bottom: 1em;
}
#options {
margin-bottom: 1em;
}
#options label {
display: block;
margin-bottom: 0.5em;
}
#submit {
font-size: 1.5em;
padding: 0.5em 1em;
}
```
JavaScript:
```javascript
// 题库
const questions = [
{ question: '1+1等于?', options: ['1', '2', '3', '4'], answer: '2' },
{ question: '3>2是?', options: ['正确的', '错误的'], answer: '正确的' },
{ question: '2*3等于?', options: ['2', '4', '6', '8'], answer: '6' }
];
// 当前题目的索引
let currentQuestionIndex = -1;
// 选项元素
const optionsElement = document.getElementById('options');
// 显示下一道题目
function showNextQuestion() {
currentQuestionIndex++;
if (currentQuestionIndex >= questions.length) {
// 已经回答完所有题目,显示考试结果对话框
showResultDialog();
return;
}
const question = questions[currentQuestionIndex];
// 显示题目
document.getElementById('question').textContent = question.question;
// 显示选项
optionsElement.innerHTML = '';
question.options.forEach(option => {
const label = document.createElement('label');
const radio = document.createElement('input');
radio.type = 'radio';
radio.name = 'answer';
radio.value = option;
label.appendChild(radio);
label.appendChild(document.createTextNode(option));
optionsElement.appendChild(label);
});
}
// 显示考试结果对话框
function showResultDialog() {
const correctCount = questions.filter(q => q.answer === getSelectedAnswer(q)).length;
const totalCount = questions.length;
alert(`你答对了${correctCount}题,总共${totalCount}题。`);
}
// 获取当前题目的选择答案
function getSelectedAnswer(question) {
const radios = [...optionsElement.querySelectorAll('input[type=radio]')];
const selectedRadio = radios.find(radio => radio.checked);
return selectedRadio ? selectedRadio.value : null;
}
// 绑定提交按钮的点击事件
document.getElementById('submit').addEventListener('click', () => {
const question = questions[currentQuestionIndex];
const selectedAnswer = getSelectedAnswer(question);
if (selectedAnswer === null) {
alert('请选择一个答案。');
return;
}
if (selectedAnswer === question.answer) {
alert('回答正确!');
} else {
alert('回答错误!');
}
showNextQuestion();
});
// 显示第一道题目
showNextQuestion();
```
这个实现只是一个简单的示例,没有考虑太多的异常情况和用户体验优化。如果要投入实际使用,还需要根据实际需求进行更加完善的开发和测试。
相关推荐
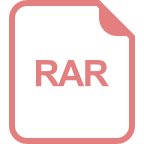
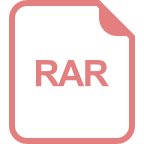














