编写c++程序,使用SIFT算法在两幅输入图像中找到特征点,并将这些特征点进行匹配。然后,使用RANSAC算法从匹配的特征点中估计两幅图像之间的单应性矩阵,并筛选出较好的匹配结果。 要求: 1.使用C++语言和OpenCV库来实现程序SIFT算法及RANSAC算法。 2.用SIFT算法检测和提取图像中的特征点。 3.使用一种合适的特征匹配算法(例如,基于最近邻点的匹配)来匹配两幅图像的特征点。 4.使用RANSAC算法从匹配的特征点中估计两幅图像之间的单应性矩阵。 5.筛选出具有较高置信度的匹配结果,并绘制出这些匹配结果。
时间: 2024-03-16 10:44:20 浏览: 72
好的,这是一个计算机视觉相关的问题,需要使用C++语言和OpenCV库来实现。下面是一个大致的实现步骤:
1. 加载并显示两幅输入图像。
2. 使用SIFT算法检测并提取两幅图像的特征点和特征描述符。
3. 使用一种特征点匹配算法(例如,基于最近邻点的匹配)来匹配两幅图像的特征点。
4. 使用RANSAC算法从匹配的特征点中估计两幅图像之间的单应性矩阵。
5. 使用估计的单应性矩阵来筛选出具有较高置信度的匹配结果。
6. 绘制出这些匹配结果,并显示在输出图像中。
下面是一个简单的实现代码:
```c++
#include <opencv2/opencv.hpp>
#include <opencv2/xfeatures2d.hpp>
using namespace cv;
using namespace cv::xfeatures2d;
int main()
{
// 加载并显示两幅输入图像
Mat img1 = imread("image1.jpg", IMREAD_GRAYSCALE);
Mat img2 = imread("image2.jpg", IMREAD_GRAYSCALE);
imshow("img1", img1);
imshow("img2", img2);
// 使用SIFT算法检测并提取两幅图像的特征点和特征描述符
Ptr<SIFT> sift = SIFT::create();
std::vector<KeyPoint> keypoints1, keypoints2;
Mat descriptors1, descriptors2;
sift->detectAndCompute(img1, noArray(), keypoints1, descriptors1);
sift->detectAndCompute(img2, noArray(), keypoints2, descriptors2);
// 使用一种特征点匹配算法(例如,基于最近邻点的匹配)来匹配两幅图像的特征点
BFMatcher matcher(NORM_L2);
std::vector<DMatch> matches;
matcher.match(descriptors1, descriptors2, matches);
// 使用RANSAC算法从匹配的特征点中估计两幅图像之间的单应性矩阵
std::vector<Point2f> points1, points2;
for (auto& match : matches) {
points1.push_back(keypoints1[match.queryIdx].pt);
points2.push_back(keypoints2[match.trainIdx].pt);
}
Mat H = findHomography(points1, points2, RANSAC);
// 使用估计的单应性矩阵来筛选出具有较高置信度的匹配结果
std::vector<Point2f> goodPoints1, goodPoints2;
std::vector<DMatch> goodMatches;
for (int i = 0; i < matches.size(); i++) {
Point2f pt1 = keypoints1[matches[i].queryIdx].pt;
Point2f pt2 = keypoints2[matches[i].trainIdx].pt;
Mat pt1Mat = (Mat_<float>(3,1) << pt1.x, pt1.y, 1);
Mat pt2Mat = (Mat_<float>(3,1) << pt2.x, pt2.y, 1);
Mat pt2Estimate = H * pt1Mat;
pt2Estimate /= pt2Estimate.at<float>(2,0);
float error = norm(pt2Mat - pt2Estimate);
if (error < 5.0) {
goodPoints1.push_back(pt1);
goodPoints2.push_back(pt2);
goodMatches.push_back(matches[i]);
}
}
// 绘制出这些匹配结果,并显示在输出图像中
Mat imgMatches;
drawMatches(img1, keypoints1, img2, keypoints2, goodMatches, imgMatches);
imshow("matches", imgMatches);
waitKey(0);
return 0;
}
```
请注意,此代码仅为示例代码,可能需要根据实际需求进行更改和优化。
阅读全文
相关推荐
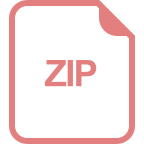
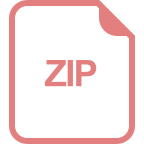

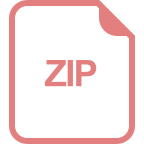
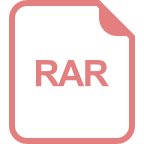
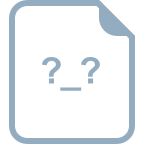
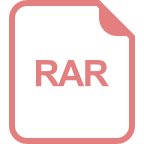
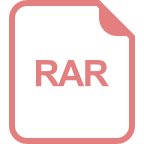
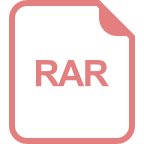
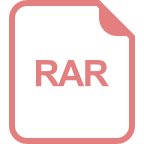
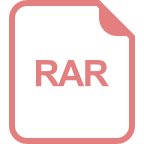
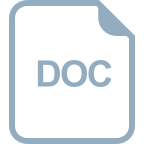
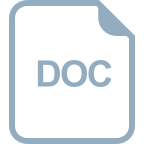




