public class BERCodec { public static byte[] encodeInteger(int value) { ByteBuffer buffer = ByteBuffer.allocate(4); buffer.putInt(value); byte[] bytes = buffer.array(); int i = 0; while(i < bytes.length && bytes[i]==0){ i++; } byte[] result = new byte[bytes.length-i+2]; result[0] = 0x02; result[1] = (byte)(bytes.length-i); System.arraycopy(bytes, i, result,2 ,bytes.length-i); return result; } public static int decodeInteger(byte[] data) { int i = data[1]; byte[] s = new byte[4]; System.arraycopy(data,2,s,4-i,i); ByteBuffer wp = ByteBuffer.wrap(s); return wp.getInt(); } public static byte[] encodeOctetString(String value) { byte[] bt = value.getBytes(); byte[] bt1 = new byte[bt.length+2]; bt1[0] = 0x04; bt1[1] = (byte) bt.length; System.arraycopy(bt,0,bt1,2,bt.length); return bt1; } public static String decodeOctetString(byte[] data) { int length = decodeLength(data[1]); return new String(data, 2, length, StandardCharsets.UTF_8); } private static int decodeLength(byte lengthByte) { if ((lengthByte & 0x80) == 0) { return lengthByte; } else { int numBytes = lengthByte & 0x7F; int length = 0; for (int i = 1; i <= numBytes; i++) { length <<= 8; length |= lengthByte & 0xFF; } return length; } } }给出详细注释
时间: 2023-12-19 14:07:03 浏览: 61
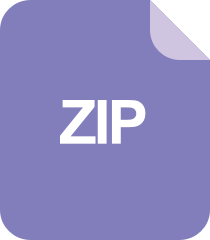
netty-buffer-4.1.68.Final-API文档-中英对照版.zip
下面是对该代码的详细注释:
```java
public class BERCodec {
// 编码整数为字节数组
public static byte[] encodeInteger(int value) {
// 分配一个 4 字节的缓冲区
ByteBuffer buffer = ByteBuffer.allocate(4);
// 将整数值放入缓冲区
buffer.putInt(value);
// 获取缓冲区的字节数组
byte[] bytes = buffer.array();
int i = 0;
// 找到第一个非零字节的位置
while (i < bytes.length && bytes[i] == 0) {
i++;
}
// 创建结果字节数组,长度为缓冲区字节数组的长度减去非零字节的数量加上 2
byte[] result = new byte[bytes.length - i + 2];
// 第一个字节为 0x02,表示整数类型
result[0] = 0x02;
// 第二个字节为长度,即缓冲区字节数组的长度减去非零字节的数量
result[1] = (byte) (bytes.length - i);
// 将缓冲区字节数组中非零字节后面的字节复制到结果字节数组中
System.arraycopy(bytes, i, result, 2, bytes.length - i);
// 返回结果字节数组
return result;
}
// 将字节数组解码为整数
public static int decodeInteger(byte[] data) {
// 第二个字节表示整数的长度
int i = data[1];
// 创建一个 4 字节的字节数组
byte[] s = new byte[4];
// 将字节数组中整数部分复制到 s 数组中
System.arraycopy(data, 2, s, 4 - i, i);
// 创建一个 ByteBuffer 对象,将 s 数组包装起来
ByteBuffer wp = ByteBuffer.wrap(s);
// 从 ByteBuffer 对象中获取整数值
return wp.getInt();
}
// 编码字符串为字节数组
public static byte[] encodeOctetString(String value) {
// 将字符串转换为字节数组
byte[] bt = value.getBytes();
// 创建一个新的字节数组,长度为原始字节数组的长度加上 2
byte[] bt1 = new byte[bt.length + 2];
// 第一个字节为 0x04,表示字符串类型
bt1[0] = 0x04;
// 第二个字节为字符串的长度
bt1[1] = (byte) bt.length;
// 将原始字节数组复制到新的字节数组中
System.arraycopy(bt, 0, bt1, 2, bt.length);
// 返回新的字节数组
return bt1;
}
// 将字节数组解码为字符串
public static String decodeOctetString(byte[] data) {
// 第二个字节表示字符串的长度
int length = decodeLength(data[1]);
// 将字节数组转换为字符串,使用 UTF-8 编码
return new String(data, 2, length, StandardCharsets.UTF_8);
}
// 解码字节长度
private static int decodeLength(byte lengthByte) {
if ((lengthByte & 0x80) == 0) {
// 如果第 8 位为 0,表示长度小于 128,直接返回该字节
return lengthByte;
} else {
// 否则,第 8 位为 1,表示长度大于 128,需要解码
int numBytes = lengthByte & 0x7F; // 获取剩余的字节长度
int length = 0;
for (int i = 1; i <= numBytes; i++) {
// 依次读取后面的字节,并将其左移 8 位,然后与前面的字节相或
length <<= 8;
length |= lengthByte & 0xFF;
}
// 返回解码后的长度
return length;
}
}
}
```
阅读全文
相关推荐
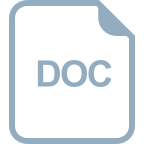
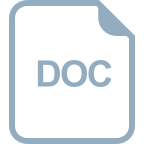














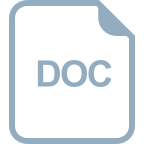
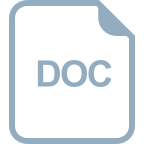