python的Json库
时间: 2024-05-10 15:18:54 浏览: 52
Python的标准库中自带了一个操作JSON数据的模块,叫做`json`。
使用这个模块,可以将Python对象(例如字典、列表等)转化为JSON格式的字符串,也可以将JSON格式的字符串转化为Python对象。
下面是一些常用的操作:
1. 将Python对象转化为JSON格式的字符串:
```python
import json
data = {
"name": "Jack",
"age": 25,
"city": "New York"
}
json_data = json.dumps(data)
print(json_data)
```
输出结果:
```json
{"name": "Jack", "age": 25, "city": "New York"}
```
2. 将JSON格式的字符串转化为Python对象:
```python
json_string = '{"name": "Jack", "age": 25, "city": "New York"}'
data = json.loads(json_string)
print(data)
```
输出结果:
```python
{'name': 'Jack', 'age': 25, 'city': 'New York'}
```
3. 将Python对象写入JSON文件:
```python
data = {
"name": "Jack",
"age": 25,
"city": "New York"
}
with open("data.json", "w") as f:
json.dump(data, f)
```
4. 从JSON文件中读取Python对象:
```python
with open("data.json", "r") as f:
data = json.load(f)
print(data)
```
输出结果:
```python
{'name': 'Jack', 'age': 25, 'city': 'New York'}
```
阅读全文
相关推荐
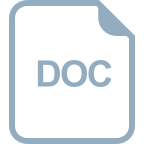
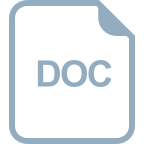
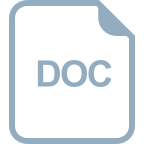
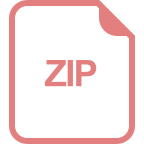
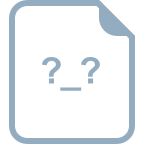
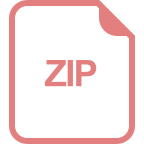
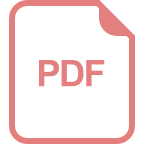
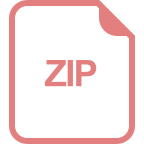
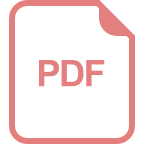
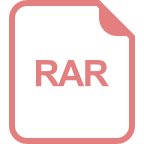
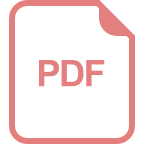
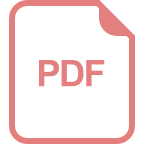
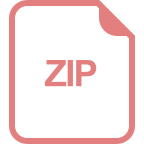
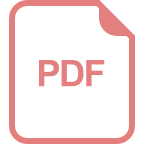
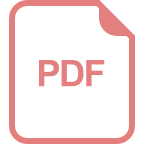
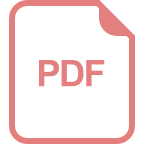
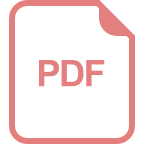
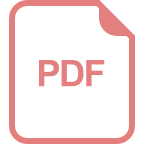
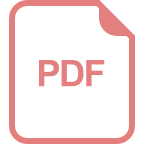