编写两段nodejs代码使得两个nodejs可以通过http协议发送字符串并接收
时间: 2023-08-10 16:10:38 浏览: 104
对于第一个nodejs程序,我们可以使用Node.js内置的http模块来创建一个简单的web服务器来接收和处理HTTP请求:
```javascript
const http = require('http');
// 创建一个HTTP Server
const server = http.createServer((req, res) => {
if (req.method === 'POST' && req.url === '/') {
// 将请求Body解析为字符串
let body = '';
req.on('data', (chunk) => {
body += chunk.toString();
});
req.on('end', () => {
// 在控制台输出收到的请求内容
console.log(`Received request body: ${body}`);
// 将请求内容作为响应返回
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end(body);
});
} else {
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end('Page not found!');
}
});
// 启动服务,监听3000端口
server.listen(3000, () => {
console.log('Server started on port 3000');
});
```
对于第二个nodejs程序,我们可以使用Node.js内置的http模块来向上面的服务器发送POST请求:
```javascript
const http = require('http');
// 定义请求Body
const postData = 'Hello, World!';
// 定义请求参数
const options = {
hostname: 'localhost',
port: 3000,
path: '/',
method: 'POST',
headers: {
'Content-Type': 'text/plain',
'Content-Length': Buffer.byteLength(postData)
}
};
// 发送HTTP请求
const req = http.request(options, (res) => {
console.log(`Received response status code: ${res.statusCode}`);
let body = '';
res.on('data', (chunk) => {
body += chunk.toString();
});
res.on('end', () => {
console.log(`Received response body: ${body}`);
});
});
// 发送请求Body
req.write(postData);
// 结束请求
req.end();
```
这样,我们就可以在控制台上看到第二个nodejs程序发送的POST请求被第一个nodejs程序接收并返回了相同的字符串。
阅读全文
相关推荐
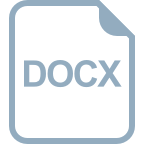
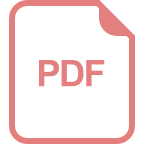
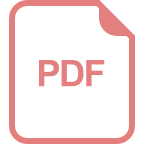
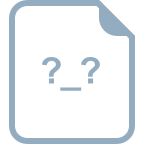
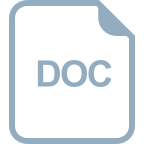
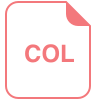
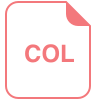
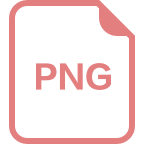
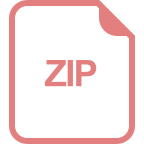
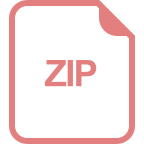
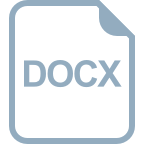