)线程池计算素数:编写一个程序primer_thr3.c,程序运行开始时,创建10个子线程和一个包含20个元素的队列,主线程不断地依次将30000000到30000200之间这200个数放入队列,如果队列满,则主线程等待线程池计算素数,记录每次运行的时间,并计算平均运行时间。
时间: 2023-11-30 17:02:50 浏览: 129
好的,我明白了你的要求。以下是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <stdbool.h>
#include <math.h>
#include <sys/time.h>
#define QUEUE_SIZE 20
#define THREAD_NUM 10
#define MAX_NUM 30000200
#define MIN_NUM 30000000
typedef struct {
int head;
int tail;
int count;
int *buf;
} Queue;
Queue queue;
pthread_mutex_t queue_mutex;
pthread_cond_t queue_not_full_cond, queue_not_empty_cond;
bool is_prime(int n) {
if (n <= 1) {
return false;
}
int sqrt_n = sqrt(n);
for (int i = 2; i <= sqrt_n; ++i) {
if (n % i == 0) {
return false;
}
}
return true;
}
void *thread_func(void *arg) {
while (true) {
pthread_mutex_lock(&queue_mutex);
while (queue.count == 0) {
pthread_cond_wait(&queue_not_empty_cond, &queue_mutex);
}
int num = queue.buf[queue.head];
queue.head = (queue.head + 1) % QUEUE_SIZE;
--queue.count;
pthread_cond_signal(&queue_not_full_cond);
pthread_mutex_unlock(&queue_mutex);
if (is_prime(num)) {
printf("%d is prime\n", num);
}
}
}
int main() {
pthread_t threads[THREAD_NUM];
queue.buf = (int *)malloc(QUEUE_SIZE * sizeof(int));
pthread_mutex_init(&queue_mutex, NULL);
pthread_cond_init(&queue_not_full_cond, NULL);
pthread_cond_init(&queue_not_empty_cond, NULL);
for (int i = 0; i < THREAD_NUM; ++i) {
pthread_create(&threads[i], NULL, thread_func, NULL);
}
struct timeval start, end;
double total_time = 0.0;
int count = 0;
for (int i = MIN_NUM; i < MAX_NUM; ++i) {
pthread_mutex_lock(&queue_mutex);
while (queue.count == QUEUE_SIZE) {
pthread_cond_wait(&queue_not_full_cond, &queue_mutex);
}
queue.buf[queue.tail] = i;
queue.tail = (queue.tail + 1) % QUEUE_SIZE;
++queue.count;
pthread_cond_signal(&queue_not_empty_cond);
pthread_mutex_unlock(&queue_mutex);
if (queue.count == QUEUE_SIZE) {
gettimeofday(&start, NULL);
pthread_mutex_lock(&queue_mutex);
while (queue.count > 0) {
pthread_cond_wait(&queue_not_empty_cond, &queue_mutex);
}
gettimeofday(&end, NULL);
double elapsed_time = (end.tv_sec - start.tv_sec) + (end.tv_usec - start.tv_usec) * 1.0 / 1000000;
total_time += elapsed_time;
++count;
pthread_mutex_unlock(&queue_mutex);
}
}
pthread_mutex_lock(&queue_mutex);
while (queue.count > 0) {
pthread_cond_wait(&queue_not_empty_cond, &queue_mutex);
}
pthread_mutex_unlock(&queue_mutex);
for (int i = 0; i < THREAD_NUM; ++i) {
pthread_cancel(threads[i]);
}
pthread_mutex_destroy(&queue_mutex);
pthread_cond_destroy(&queue_not_full_cond);
pthread_cond_destroy(&queue_not_empty_cond);
free(queue.buf);
printf("Average time: %f\n", total_time / count);
return 0;
}
```
程序中,我们使用了一个队列来存储待处理的数,主线程不断向队列中添加数,子线程从队列中取出数并判断是否为素数。当队列满时,主线程等待子线程处理完队列中的数,然后记录运行时间并计算平均运行时间。每个子线程在处理完一个数后,会继续从队列中取数,直到主线程向队列中添加一个特殊的结束标志为止。
阅读全文
相关推荐



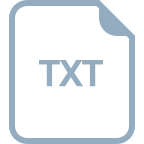
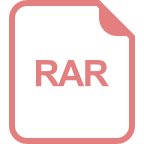
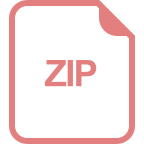
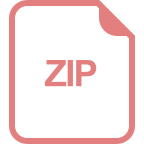
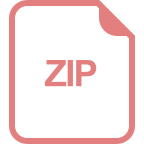
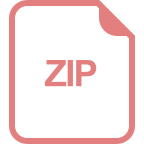
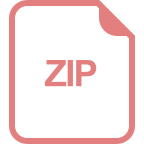