python中判断字符串中是否出现某子串
时间: 2023-11-01 09:06:45 浏览: 153
您可以使用 Python 中的 `in` 运算符来判断一个字符串是否包含某个子串,示例如下:
```python
string = "Hello, world!"
if "world" in string:
print("子串存在于字符串中")
else:
print("子串不存在于字符串中")
```
输出结果为:
```
子串存在于字符串中
```
您还可以使用字符串的 `find()` 函数来查找子串在字符串中的位置,若不存在则返回 -1,示例如下:
```python
string = "Hello, world!"
index = string.find("world")
if index != -1:
print("子串存在于字符串中,位置为", index)
else:
print("子串不存在于字符串中")
```
输出结果为:
```
子串存在于字符串中,位置为 7
```
相关问题
python中如何在字符串中判断是否有某子串
在 Python 中,可以使用 `in` 关键字来判断一个字符串中是否包含某个子串。示例代码如下:
```python
s = "hello world"
if "world" in s:
print("s 中包含子串 'world'")
else:
print("s 中不包含子串 'world'")
```
输出结果为:
```
s 中包含子串 'world'
```
如果你要判断一个字符串中不包含某个子串,可以使用 `not in` 关键字,示例代码如下:
```python
s = "hello world"
if "python" not in s:
print("s 中不包含子串 'python'")
else:
print("s 中包含子串 'python'")
```
输出结果为:
```
s 中不包含子串 'python'
```
python 如何判断一个字符串是否为另一个字符串的子串
在Python中,你可以使用`in`关键字来判断一个字符串是否为另一个字符串的子串。下面是一个示例代码:
```python
string1 = "Hello, World!"
string2 = "Hello"
if string2 in string1:
print("string2是string1的子串")
else:
print("string2不是string1的子串")
```
在这个例子中,我们使用`in`关键字来判断`string2`是否是`string1`的子串。如果是,则输出"string2是string1的子串";如果不是,则输出"string2不是string1的子串"。
需要注意的是,Python中的字符串判断是区分大小写的。如果你希望忽略大小写进行判断,可以使用字符串的`lower()`或`upper()`方法将两个字符串转换为统一的大小写再进行比较。例如:
```python
string1 = "Hello, World!"
string2 = "hello"
if string2.lower() in string1.lower():
print("string2是string1的子串")
else:
print("string2不是string1的子串")
```
这样就可以忽略大小写进行判断了。
阅读全文
相关推荐
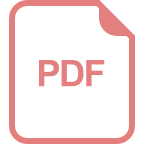
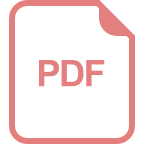
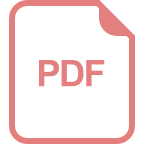










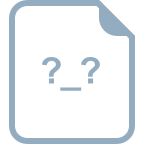