sqlite数据库和mysql数据库可以通用吗
时间: 2023-09-11 22:06:14 浏览: 292
虽然SQLite和MySQL都是关系型数据库,但它们的实现和功能有所不同,因此不能直接通用。SQLite是一种嵌入式数据库,适合于轻量级、单用户、本地应用程序。而MySQL则是一种完整的客户端/服务器数据库系统,适合于大型多用户应用程序。如果要在两个系统之间共享数据,需要将数据从一个数据库转移到另一个数据库,可以使用一些工具或脚本来实现。
相关问题
python用csv模块和pymysql模块完成.设有数据文件student.csv,内容如下图所示,请设计程序将此文件中的数据导入SQLite数据库或MySQL数据库stu.db的表data中,并对其中的数据进行插入、删除、更改、查询操作。将以上操作封装成函数。
好的,我可以为您提供相应的代码。首先需要安装pandas、PyMySQL和sqlite3模块,您可以使用以下命令安装:
```
pip install pandas
pip install PyMySQL
```
如果您需要使用SQLite数据库,则无需安装PyMySQL模块,只需安装sqlite3模块即可。
以下是将student.csv导入MySQL数据库的代码示例:
```python
import pandas as pd
import pymysql
# 读取csv文件
data = pd.read_csv('student.csv')
# 连接MySQL数据库
conn = pymysql.connect(
host='localhost',
port=3306,
user='root',
password='root',
db='stu',
charset='utf8mb4'
)
# 将数据写入MySQL数据库
data.to_sql(name='data', con=conn, if_exists='replace', index=False)
# 关闭数据库连接
conn.close()
```
以下是将student.csv导入SQLite数据库的代码示例:
```python
import pandas as pd
import sqlite3
# 读取csv文件
data = pd.read_csv('student.csv')
# 连接SQLite数据库
conn = sqlite3.connect('stu.db')
# 将数据写入SQLite数据库
data.to_sql(name='data', con=conn, if_exists='replace', index=False)
# 关闭数据库连接
conn.close()
```
以下是封装了插入、删除、更改、查询操作的函数示例:
```python
import pandas as pd
import pymysql
import sqlite3
def import_data(file_path, db_type, db_name, db_host='localhost', db_port=3306, db_user='root', db_password='root'):
# 读取csv文件
data = pd.read_csv(file_path)
# 连接数据库
if db_type == 'mysql':
conn = pymysql.connect(
host=db_host,
port=db_port,
user=db_user,
password=db_password,
db=db_name,
charset='utf8mb4'
)
elif db_type == 'sqlite':
conn = sqlite3.connect(db_name)
# 将数据写入数据库
data.to_sql(name='data', con=conn, if_exists='replace', index=False)
# 关闭数据库连接
conn.close()
def insert_data(db_type, db_name, db_host='localhost', db_port=3306, db_user='root', db_password='root', **kwargs):
# 连接数据库
if db_type == 'mysql':
conn = pymysql.connect(
host=db_host,
port=db_port,
user=db_user,
password=db_password,
db=db_name,
charset='utf8mb4'
)
elif db_type == 'sqlite':
conn = sqlite3.connect(db_name)
# 插入数据
cursor = conn.cursor()
sql = 'INSERT INTO data VALUES (%s, %s, %s, %s, %s)'
cursor.execute(sql, (kwargs['id'], kwargs['name'], kwargs['gender'], kwargs['age'], kwargs['score']))
conn.commit()
# 关闭数据库连接
conn.close()
def delete_data(db_type, db_name, db_host='localhost', db_port=3306, db_user='root', db_password='root', id):
# 连接数据库
if db_type == 'mysql':
conn = pymysql.connect(
host=db_host,
port=db_port,
user=db_user,
password=db_password,
db=db_name,
charset='utf8mb4'
)
elif db_type == 'sqlite':
conn = sqlite3.connect(db_name)
# 删除数据
cursor = conn.cursor()
sql = 'DELETE FROM data WHERE id = %s'
cursor.execute(sql, id)
conn.commit()
# 关闭数据库连接
conn.close()
def update_data(db_type, db_name, db_host='localhost', db_port=3306, db_user='root', db_password='root', id, **kwargs):
# 连接数据库
if db_type == 'mysql':
conn = pymysql.connect(
host=db_host,
port=db_port,
user=db_user,
password=db_password,
db=db_name,
charset='utf8mb4'
)
elif db_type == 'sqlite':
conn = sqlite3.connect(db_name)
# 更改数据
cursor = conn.cursor()
sql = 'UPDATE data SET name = %s, gender = %s, age = %s, score = %s WHERE id = %s'
cursor.execute(sql, (kwargs['name'], kwargs['gender'], kwargs['age'], kwargs['score'], id))
conn.commit()
# 关闭数据库连接
conn.close()
def select_data(db_type, db_name, db_host='localhost', db_port=3306, db_user='root', db_password='root', id=None):
# 连接数据库
if db_type == 'mysql':
conn = pymysql.connect(
host=db_host,
port=db_port,
user=db_user,
password=db_password,
db=db_name,
charset='utf8mb4'
)
elif db_type == 'sqlite':
conn = sqlite3.connect(db_name)
# 查询数据
cursor = conn.cursor()
if id is None:
sql = 'SELECT * FROM data'
cursor.execute(sql)
else:
sql = 'SELECT * FROM data WHERE id = %s'
cursor.execute(sql, id)
results = cursor.fetchall()
# 关闭数据库连接
conn.close()
return results
```
您可以使用以下代码调用函数示例:
```python
# 将数据导入MySQL数据库
import_data('student.csv', 'mysql', 'stu')
# 插入一条记录
insert_data('mysql', 'stu', id=1001, name='Tom', gender='M', age=18, score=90)
# 删除一条记录
delete_data('mysql', 'stu', id=1001)
# 更改一条记录
update_data('mysql', 'stu', id=1002, name='Lucy', gender='F', age=19, score=95)
# 查询所有记录
results = select_data('mysql', 'stu')
for row in results:
print(row)
# 查询一条记录
result = select_data('mysql', 'stu', id=1002)
print(result)
```
1)将SQLite数据库练习里,学生管理数据库系统中的表全部新建到MySQL里,并将数据导入到数据库中。用Python实现
首先,我们需要在MySQL中新建数据库和表。假设我们新建了一个名为`student`的数据库,并在其中创建了一个名为`student_info`的表,表结构如下:
```mysql
CREATE TABLE student_info (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(50) NOT NULL,
age INT(11) NOT NULL,
gender VARCHAR(10) NOT NULL,
PRIMARY KEY (id)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
接下来,我们需要将SQLite中的数据导入到MySQL中。我们可以使用Python的`sqlite3`和`pymysql`模块来实现这个过程。具体步骤如下:
1. 连接SQLite数据库并查询数据。
```python
import sqlite3
# 连接SQLite数据库
conn_sqlite = sqlite3.connect('student.db')
# 查询数据
cursor_sqlite = conn_sqlite.cursor()
cursor_sqlite.execute('SELECT * FROM student')
students = cursor_sqlite.fetchall()
# 关闭连接
cursor_sqlite.close()
conn_sqlite.close()
```
2. 连接MySQL数据库并插入数据。
```python
import pymysql
# 连接MySQL数据库
conn_mysql = pymysql.connect(host='localhost', port=3306, user='root', password='123456', db='student', charset='utf8')
cursor_mysql = conn_mysql.cursor()
# 插入数据
for student in students:
sql = "INSERT INTO student_info (name, age, gender) VALUES (%s, %s, %s)"
cursor_mysql.execute(sql, (student[1], student[2], student[3]))
# 提交事务并关闭连接
conn_mysql.commit()
cursor_mysql.close()
conn_mysql.close()
```
完整代码如下:
```python
import sqlite3
import pymysql
# 连接SQLite数据库
conn_sqlite = sqlite3.connect('student.db')
# 查询数据
cursor_sqlite = conn_sqlite.cursor()
cursor_sqlite.execute('SELECT * FROM student')
students = cursor_sqlite.fetchall()
# 关闭连接
cursor_sqlite.close()
conn_sqlite.close()
# 连接MySQL数据库
conn_mysql = pymysql.connect(host='localhost', port=3306, user='root', password='123456', db='student', charset='utf8')
cursor_mysql = conn_mysql.cursor()
# 插入数据
for student in students:
sql = "INSERT INTO student_info (name, age, gender) VALUES (%s, %s, %s)"
cursor_mysql.execute(sql, (student[1], student[2], student[3]))
# 提交事务并关闭连接
conn_mysql.commit()
cursor_mysql.close()
conn_mysql.close()
```
运行代码后,MySQL数据库中的`student_info`表中就会有和SQLite数据库中相同的数据。
阅读全文
相关推荐
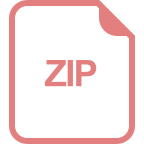
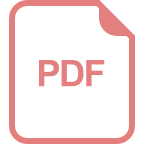
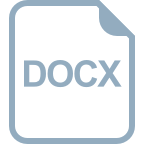
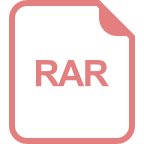
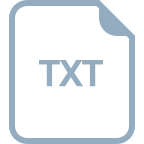
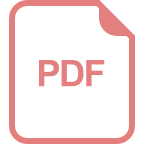
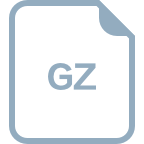
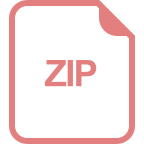
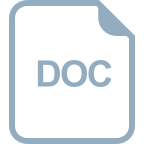
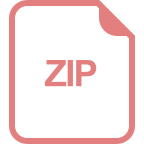
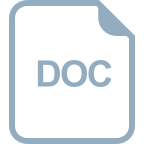
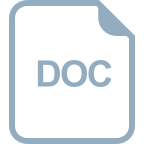
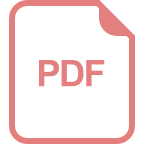
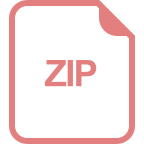
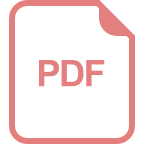
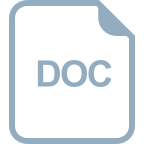