C# 绘制正态分布效果图
时间: 2023-07-12 22:05:25 浏览: 56
以下是使用 C# 绘制正态分布效果图的示例代码:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
namespace NormalDistribution
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Paint(object sender, PaintEventArgs e)
{
int width = this.ClientSize.Width;
int height = this.ClientSize.Height;
double mu = 0; // 均值
double sigma = 1; // 标准差
double x, y, px, py, dx;
double[] p = new double[width];
double maxp = 0;
// 计算每个像素点上的概率密度值
for (int i = 0; i < width; i++)
{
x = (double)i / width * 10 - 5; // 将像素点映射到 [-5, 5] 区间上
y = Math.Exp(-(x - mu) * (x - mu) / (2 * sigma * sigma)) / (sigma * Math.Sqrt(2 * Math.PI));
p[i] = y;
if (y > maxp)
maxp = y;
}
// 将概率密度值转换为像素点坐标
for (int i = 0; i < width; i++)
{
px = (double)i / width * width;
py = (1 - p[i] / maxp) * height;
if (i == 0)
e.Graphics.MoveTo((int)px, (int)py);
else
{
dx = Math.Abs(px - p[i - 1]);
if (dx > 1)
e.Graphics.MoveTo((int)px, (int)py);
else
e.Graphics.LineTo((int)px, (int)py);
}
}
}
}
}
```
在窗体的 `Paint` 事件中绘制正态分布曲线,使用 `Graphics` 对象的 `MoveTo` 和 `LineTo` 方法绘制曲线。具体步骤如下:
1. 计算每个像素点上的概率密度值,这里假设均值为 0,标准差为 1;
2. 将概率密度值转换为像素点坐标,将像素点映射到 [-5, 5] 区间上;
3. 使用 `MoveTo` 和 `LineTo` 方法绘制曲线。
需要注意的是,因为正态分布曲线在中心附近概率密度值较大,而在两侧概率密度值较小,所以需要将概率密度值转换为像素点坐标时,使用 `(1 - p[i] / maxp)` 计算纵坐标,使得曲线在中心附近较高,两侧较低。
相关推荐
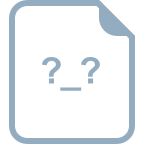














