给出一个调用folly库实现多线程的示例
时间: 2023-07-13 07:17:44 浏览: 250
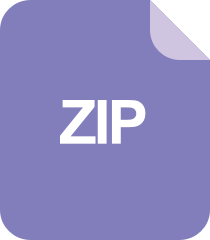
一个创建多线程的例子
以下是使用folly库实现多线程的示例代码:
```c++
#include <iostream>
#include <vector>
#include <folly/executors/GlobalExecutor.h>
#include <folly/futures/Future.h>
using namespace std;
using namespace folly;
int main() {
// 创建一个folly的线程池,其中包含4个线程
auto executor = std::make_shared<folly::CPUThreadPoolExecutor>(4);
// 使用folly::Future实现多线程
std::vector<Future<int>> futures;
for (int i = 0; i < 10; i++) {
futures.push_back(folly::via(executor.get(), [i]() {
cout << "Thread " << std::this_thread::get_id() << " is running task " << i << endl;
return i * i;
}));
}
// 等待所有futures完成并输出结果
folly::collectAll(futures.begin(), futures.end())
.then([](const std::vector<folly::Try<int>>& results) {
cout << "All tasks are completed!" << endl;
for (const auto& result : results) {
if (result.hasValue()) {
cout << "Result: " << result.value() << endl;
} else {
cout << "Error: " << result.exception().what() << endl;
}
}
})
.wait();
return 0;
}
```
在上面的示例中,我们使用了folly::CPUThreadPoolExecutor创建了一个包含4个线程的线程池。通过folly::via函数和lambda表达式,我们将10个任务分别提交到线程池中,并使用folly::Future保存了每个任务的执行结果。最后,我们使用folly::collectAll函数等待所有任务执行完成,并输出结果。由于使用了线程池和异步执行,这些任务可以并发执行,提高了程序的执行效率。
阅读全文
相关推荐

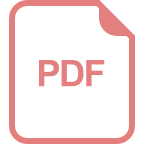
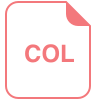
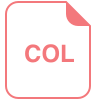
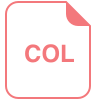
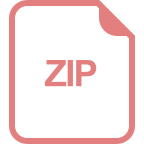
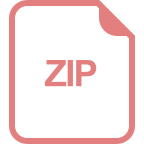
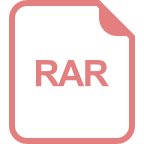
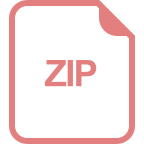
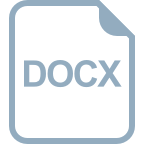