9 48 C:\Users\DELL\Desktop\未命名1.cpp [Error] in C++98 'teams' must be initialized by constructor, not by '{...}'
时间: 2023-12-12 19:51:28 浏览: 46
这个错误提示是因为你在定义一个类时,其中一个成员变量 `teams` 是一个类类型,但是你却使用了花括号 `{}` 来进行初始化。这种方式只适用于内置类型或者数组类型的初始化,对于类类型的成员变量初始化,需要使用构造函数进行初始化。
因此,你需要在类的定义中添加一个构造函数来对 `teams` 进行初始化,例如:
```
class MyClass {
private:
Team teams;
public:
MyClass() : teams(Team()) {}
};
```
这里的 `Team()` 表示使用 `Team` 类的默认构造函数来进行初始化。你也可以使用其他的构造函数来进行初始化,具体可以根据你的实际需求来选择。
相关问题
"timestamp":1713759117044, "status":404, "error":"Not Found", "message":"No message available", "path":"/teams/add"
根据提供的信息,这是一个API请求的响应结果。具体解释如下:
- "timestamp":1713759117044 表示响应的时间戳,以毫秒为单位。
- "status":404 表示请求的资源未找到,即请求的路径或接口不存在。
- "error":"Not Found" 表示错误类型为“未找到”,即请求的资源不存在。
- "message":"No message available" 表示错误信息不可用,可能是因为请求的路径或接口不存在,没有提供详细的错误信息。
- "path":"/teams/add" 表示请求的路径是"/teams/add",即请求添加团队的接口。
specification by example: how successful teams deliver the right software
“规范化示例”的概念起源于敏捷软件开发方法论,它是一种基于实例和集体理解的软件规范方法。成功的团队通过规范化示例交付正确的软件。
规范化示例的核心思想是通过一系列实际案例来定义软件的功能需求和行为。这些案例是从真实业务场景中提取的,描述了用户和系统之间的交互,以及期望的结果。团队成员通过与利益相关者一起讨论、验证和澄清这些示例,共同理解和定义软件的要求。
规范化示例的成功之处在于有效地传递信息。与传统的需求文档不同,规范化示例提供了直观、具体和可测量的例子,帮助团队和利益相关者更好地理解软件所需的功能和行为。这种可视化的形式有助于避免语义歧义和“对理解的理解”,减少沟通误差,提高团队的协作效率。
另外,规范化示例还加强了团队的自组织能力。通过参与规范化示例的制定和验证,团队成员之间的合作与沟通得到加强,每个人都可以更好地理解软件的需求和目标。这种集体的参与和讨论有助于提高团队的专业素养,减少个人行为对软件交付的负面影响,并增强团队对软件需求变化的适应能力。
另外,规范化示例还有助于提高软件的质量。通过与规范化示例进行对比和验证,团队可以快速发现和解决潜在的问题和漏洞,确保软件的功能和行为符合用户的期望。这种迭代式的开发和测试方法可以有效降低软件交付过程中的风险,提高软件的稳定性和可靠性。
总之,规范化示例是一种成功团队交付正确软件的有效方法。它提供了直观、具体和可测量的需求和行为定义,促进团队协作和自组织能力,增强软件质量和交付效率。
相关推荐
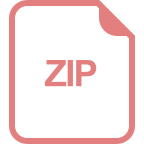
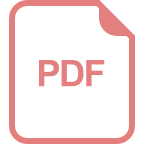












