android studio拨号打电话
时间: 2023-04-29 21:01:15 浏览: 253
要在Android Studio中拨打电话,您需要使用以下代码:
1. 在AndroidManifest.xml文件中添加以下权限:
```
<uses-permission android:name="android.permission.CALL_PHONE" />
```
2. 在您的活动中添加以下代码:
```
String phoneNumber = "123456789"; // 替换为您要拨打的电话号码
Intent intent = new Intent(Intent.ACTION_CALL);
intent.setData(Uri.parse("tel:" + phoneNumber));
startActivity(intent);
```
请注意,使用ACTION_CALL意图需要CALL_PHONE权限。如果您的应用程序在Android 6.或更高版本上运行,则还需要在运行时请求权限。
相关问题
android studio拨号
Android Studio可以实现简单拨号功能。首先,你需要在点击事件中调用拨号功能的代码。你可以使用Intent来启动拨号界面,并传递电话号码作为参数。在点击事件中,你可以使用以下代码来实现拨号功能:
```java
@Override
public void onClick(View v) {
Intent intent = new Intent(Intent.ACTION_DIAL);
intent.setData(Uri.parse("tel:电话号码"));
startActivity(intent);
}
```
其中,"电话号码"是你要拨打的电话号码。通过调用`startActivity(intent)`来启动拨号界面。这样,当用户点击相关按钮时,就会打开拨号界面并自动填充电话号码。
请注意,为了使用拨号功能,你需要在AndroidManifest.xml文件中添加以下权限:
```xml
<uses-permission android:name="android.permission.CALL_PHONE" />
```
这样,你就可以在Android Studio中实现简单的拨号功能了。
#### 引用[.reference_title]
- *1* *2* *3* [Android Studio实现简单拨号,短信发送,照相机调用,地图打开的功能(Android Studio学习笔记3)](https://blog.csdn.net/weixin_46628481/article/details/120405642)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
android studio实现打电话
要在Android Studio中实现打电话,可以使用以下代码:
1. 在AndroidManifest.xml文件中添加以下权限:
<uses-permission android:name="android.permission.CALL_PHONE" />
2. 在Activity中添加以下代码:
String phoneNumber = "123456789"; // 替换为要拨打的电话号码
Intent intent = new Intent(Intent.ACTION_CALL);
intent.setData(Uri.parse("tel:" + phoneNumber));
startActivity(intent);
注意:在Android 6.及以上版本中,需要在运行时请求CALL_PHONE权限。可以使用以下代码:
if (ContextCompat.checkSelfPermission(this, Manifest.permission.CALL_PHONE) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.CALL_PHONE}, REQUEST_CALL_PHONE);
} else {
// 已经授权,执行打电话操作
}
其中REQUEST_CALL_PHONE是自定义的请求码,用于处理权限请求结果。在onRequestPermissionsResult方法中处理权限请求结果:
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
if (requestCode == REQUEST_CALL_PHONE) {
if (grantResults.length > && grantResults[] == PackageManager.PERMISSION_GRANTED) {
// 授权成功,执行打电话操作
} else {
// 授权失败,提示用户
}
}
}
相关推荐
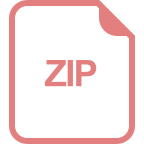
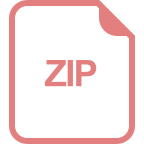
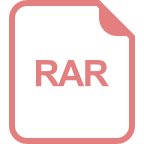
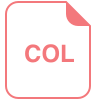
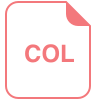
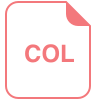
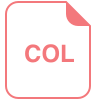
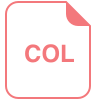







