帮我写一个前端页面实现弹幕的效果
时间: 2024-06-12 15:04:38 浏览: 10
以下是一个简单的前端页面实现弹幕效果的示例:
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>弹幕效果</title>
<meta charset="UTF-8">
<style>
#danmu {
position: relative;
height: 300px;
width: 500px;
background-color: #EEE;
overflow: hidden;
}
#danmu div {
position: absolute;
font-size: 14px;
color: #FFF;
white-space: nowrap;
user-select: none;
-webkit-user-select: none;
transform: translateX(-100%);
animation: danmu 5s linear;
animation-fill-mode: forwards;
}
@keyframes danmu {
to {
transform: translateX(100%);
}
}
</style>
</head>
<body>
<div id="danmu"></div>
<form onsubmit="return false;">
<input type="text" id="message">
<button onclick="send()">发送</button>
</form>
<script>
var danmu = document.getElementById('danmu');
var message = document.getElementById('message');
function send() {
var text = message.value;
if (text.length > 0) {
var div = document.createElement('div');
div.innerText = text;
div.style.top = Math.floor(Math.random() * (danmu.offsetHeight - 20)) + 'px';
danmu.appendChild(div);
message.value = '';
}
}
</script>
</body>
</html>
```
CSS代码:
```css
#danmu {
position: relative;
height: 300px;
width: 500px;
background-color: #EEE;
overflow: hidden;
}
#danmu div {
position: absolute;
font-size: 14px;
color: #FFF;
white-space: nowrap;
user-select: none;
-webkit-user-select: none;
transform: translateX(-100%);
animation: danmu 5s linear;
animation-fill-mode: forwards;
}
@keyframes danmu {
to {
transform: translateX(100%);
}
}
```
JavaScript代码:
```javascript
var danmu = document.getElementById('danmu');
var message = document.getElementById('message');
function send() {
var text = message.value;
if (text.length > 0) {
var div = document.createElement('div');
div.innerText = text;
div.style.top = Math.floor(Math.random() * (danmu.offsetHeight - 20)) + 'px';
danmu.appendChild(div);
message.value = '';
}
}
```
解释:
1. HTML部分包含一个div元素和一个表单元素。div元素用于显示弹幕,表单元素用于输入弹幕内容。
2. CSS部分定义了弹幕容器的样式和弹幕元素的样式,其中弹幕元素使用了CSS动画来实现从左到右的滚动效果。
3. JavaScript部分定义了发送弹幕的函数,该函数会创建一个新的弹幕元素并添加到弹幕容器中。弹幕的位置使用了随机数来实现。
4. 在表单元素中使用onsubmit属性,并返回false,可以防止页面刷新。在发送按钮中调用send函数即可发送弹幕。
相关推荐
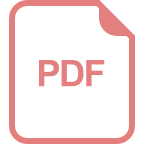
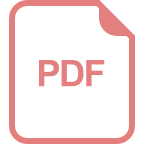
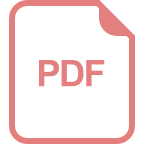














