你现在是一位高级的python工程师,现在要求你写一个比较全面的脚本,该脚本功能用于读取各种类型的文件的数据,并将读出来的数据已列表的形式存在
时间: 2024-02-24 16:57:50 浏览: 22
好的,以下是一个可以读取各种类型文件的数据并将其存储为列表的Python脚本,包括文本文件、CSV文件、JSON文件、XML文件和Excel文件:
```python
import csv
import json
import xml.etree.ElementTree as ET
import openpyxl
def read_text_file(file_path):
with open(file_path, 'r') as f:
lines = f.readlines()
return [line.strip() for line in lines]
def read_csv_file(file_path):
with open(file_path, 'r') as f:
reader = csv.reader(f)
data = [row for row in reader]
return data
def read_json_file(file_path):
with open(file_path, 'r') as f:
data = json.load(f)
return data
def read_xml_file(file_path):
tree = ET.parse(file_path)
root = tree.getroot()
data = []
for child in root:
record = {}
for sub_child in child:
record[sub_child.tag] = sub_child.text
data.append(record)
return data
def read_excel_file(file_path, sheet_name):
workbook = openpyxl.load_workbook(file_path)
sheet = workbook[sheet_name]
data = []
for row in sheet.iter_rows(values_only=True):
data.append(list(row))
return data
if __name__ == '__main__':
file_path = 'example.txt'
data = read_text_file(file_path)
print(data)
file_path = 'example.csv'
data = read_csv_file(file_path)
print(data)
file_path = 'example.json'
data = read_json_file(file_path)
print(data)
file_path = 'example.xml'
data = read_xml_file(file_path)
print(data)
file_path = 'example.xlsx'
sheet_name = 'Sheet1'
data = read_excel_file(file_path, sheet_name)
print(data)
```
您可以根据需要调用适当的函数来读取您想要的文件类型,并将数据存储在列表中。注意,在读取Excel文件时,需要使用openpyxl库。
相关推荐
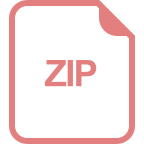
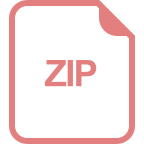














