public class PerfectSquare { public static void main(String[] args) { for (int i = 1; ; i++) { int x = i + 100; // 加上 100 后的数 int y = x + 168; // 再加上 168 后的数 // 判断 x 是否为完全平方数,y 是否为完全平方数 if (isPerfectSquare(x) && isPerfectSquare(y)) { System.out.println("符合条件的整数为:" + i); break; } } } // 判断一个数是否为完全平方数 public static boolean isPerfectSquare(int n) { int sqrt = (int) Math.sqrt(n); return sqrt * sqrt == n; } }解释一下这段代码
时间: 2024-02-14 10:19:58 浏览: 34
这段代码的作用是寻找符合一定条件的整数。具体来说,这个程序会从1开始不断枚举整数 i,然后判断 i + 100 和 i + 100 + 168 是否都为完全平方数。如果是的话,就输出符合条件的整数 i,并停止程序。
其中,isPerfectSquare(int n) 方法是用来判断一个数是否为完全平方数的。它通过计算这个数的平方根,然后判断这个平方根的平方是否等于原数,从而得出结论。
相关问题
Java(Algebra: perfect square) Write a program that prompts the user to enter an integer m and find the smallest integer n such that m * n is a perfect square. (Hint: Store all smallest factors of m into an array list. n is the product of the factors that appear an odd number of times in the array list. For example, consider m = 90, store the factors 2, 3, 3, and 5 in an array list. 2 and 5 appear an odd number of times in the array list. Thus, n is 10.) Here are some sample runs: Enter an integer m: 1500 The smallest number n for m * n to be a perfect square is 15 m * n is 22500
Here's the Java program to solve the problem:
```java
import java.util.ArrayList;
import java.util.Scanner;
public class PerfectSquare {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter an integer m: ");
int m = input.nextInt();
// Find all smallest factors of m and store them in an array list
ArrayList<Integer> factors = new ArrayList<Integer>();
int factor = 2;
while (factor <= m) {
if (m % factor == 0) {
factors.add(factor);
m /= factor;
} else {
factor++;
}
}
// Find the product of the factors that appear an odd number of times
int n = 1;
for (int i = 0; i < factors.size(); i++) {
int count = 0;
for (int j = 0; j < factors.size(); j++) {
if (factors.get(i) == factors.get(j)) {
count++;
}
}
if (count % 2 != 0) {
n *= factors.get(i);
}
}
// Compute m * n and output the result
int product = m * n;
System.out.println("The smallest number n for m * n to be a perfect square is " + n);
System.out.println("m * n is " + product);
}
}
```
Explanation:
- We first prompt the user to enter an integer `m`.
- We then find all smallest factors of `m` and store them in an array list `factors`. We use a loop with a variable `factor` that starts from 2 and goes up to `m`. If `m` is divisible by `factor`, we add `factor` to the array list and divide `m` by `factor`. Otherwise, we increment `factor`.
- After we have all the factors in the array list, we need to find the product of the factors that appear an odd number of times. We use a nested loop to count the occurrences of each factor in the array list. If the count is odd, we multiply the factor to `n`.
- Finally, we compute `m * n` and output the result.
Java (Algebra: perfect square) Write a program that prompts the user to enter an integer m and find the smallest integer n such that m * n is a perfect square. (Hint: Store all smallest factors of m into an array list. n is the product of the factors that appear an odd number of times in the array list. For example, consider m = 90, store the factors 2, 3, 3, and 5 in an array list. 2 and 5 appear an odd number of times in the array list. Thus, n is 10.)
Here's the Java code to solve the problem:
```
import java.util.ArrayList;
import java.util.Scanner;
public class PerfectSquare {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter an integer m: ");
int m = input.nextInt();
ArrayList<Integer> factors = new ArrayList<Integer>();
int factor = 2;
while (factor <= m) {
if (m % factor == 0) {
factors.add(factor);
m /= factor;
}
else {
factor++;
}
}
int n = 1;
for (int i = 0; i < factors.size(); i++) {
int count = 1;
for (int j = i + 1; j < factors.size(); j++) {
if (factors.get(i) == factors.get(j)) {
count++;
}
}
if (count % 2 != 0) {
n *= factors.get(i);
}
}
System.out.println("The smallest integer n such that m * n is a perfect square is: " + n);
}
}
```
Explanation:
1. We first prompt the user to enter an integer `m` using the `Scanner` class.
2. We then create an `ArrayList` called `factors` to store all the smallest factors of `m`.
3. We use a `while` loop to find all the smallest factors of `m`. If `m` is divisible by `factor`, we add it to the `factors` list and divide `m` by `factor`. Otherwise, we increment `factor` by 1.
4. We then use two nested `for` loops to check how many times each factor appears in the `factors` list. If a factor appears an odd number of times, we multiply it with `n`.
5. Finally, we print out the value of `n`.
阅读全文
相关推荐
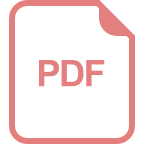
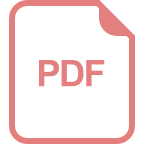
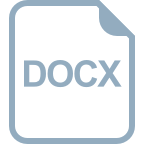



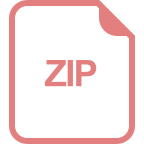
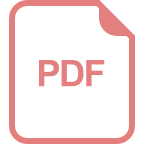
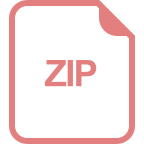
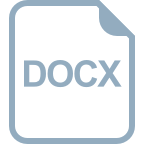
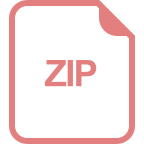
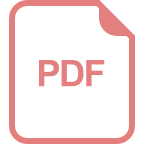