public class Calculator extends JFrame implements ActionListener什么意思
时间: 2023-06-15 22:04:30 浏览: 116
这段代码定义了一个名为 Calculator 的类,该类继承自 JFrame 类,并实现了 ActionListener 接口。继承自 JFrame 类意味着该类具有 JFrame 类的所有属性和方法,可以用于创建一个窗口界面。实现 ActionListener 接口意味着该类必须实现 actionPerformed() 方法,以响应用户界面中的操作事件。因此,这个类可能会包含一些与计算器相关的操作,例如加、减、乘、除等,以及一些用于响应这些操作的方法。
相关问题
Calculator extends JFrame implements ActionListener什么意思
这段代码定义了一个名为 Calculator 的类,它继承了 JFrame 类并实现了 ActionListener 接口。继承 JFrame 类意味着 Calculator 类拥有了 JFrame 类的所有属性和方法,可以用来创建一个窗口界面。实现 ActionListener 接口意味着 Calculator 类必须实现 ActionListener 接口中的方法,以响应用户在界面上的操作事件。因此,这段代码定义了一个可以接收用户输入并进行计算的窗口应用程序。
use Java to achieve:Create a simple calculator Layout The calculator has: ● a display label at the top which will be used to show the calculation results ● a text field for the user to input an integer/double in the middle ● buttons for “clear”, “+”, “-”, “*” and “/” in the bottom Desired final look: Functionality ● Create an accumulator state variable that keeps track of the current overall value of the calculation. ● Clicking the “clear” button, set the accumulator value to 0 ● Clicking the “+” button, add the text field value to the accumulator value ● Clicking the “-” button, subtract the text field value from the accumulator value ● Clicking the “*” button, multiply the accumulator value by the text field value ● Clicking the “/” button, divide the accumulator value by the text field value ● After a button has been pressed, update the display label with the new accumulator value and empty the text field. (Correct layout: 0.25P, Class extends JFrame: 0.25P, Class follows OOP principles: 0.25P, Global set-up in main method: 0.25P) (Class implements ActionListener: 0.25P, Uses accumulator variable: 0.25P, Works as intended: 0.25P, Input checks to prevent errors: 0.25P)2 2
Sure, here's an example code in Java that meets your requirements:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class Calculator extends JFrame implements ActionListener {
private JLabel displayLabel;
private JTextField inputField;
private JButton clearButton, addButton, subtractButton, multiplyButton, divideButton;
private double accumulator = 0.0;
public Calculator() {
super("Calculator");
setLayout(new BorderLayout());
displayLabel = new JLabel("0.0", JLabel.RIGHT);
displayLabel.setPreferredSize(new Dimension(200, 30));
add(displayLabel, BorderLayout.NORTH);
inputField = new JTextField(10);
add(inputField, BorderLayout.CENTER);
JPanel buttonPanel = new JPanel(new GridLayout(1, 5));
clearButton = new JButton("Clear");
addButton = new JButton("+");
subtractButton = new JButton("-");
multiplyButton = new JButton("*");
divideButton = new JButton("/");
clearButton.addActionListener(this);
addButton.addActionListener(this);
subtractButton.addActionListener(this);
multiplyButton.addActionListener(this);
divideButton.addActionListener(this);
buttonPanel.add(clearButton);
buttonPanel.add(addButton);
buttonPanel.add(subtractButton);
buttonPanel.add(multiplyButton);
buttonPanel.add(divideButton);
add(buttonPanel, BorderLayout.SOUTH);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == clearButton) {
accumulator = 0.0;
displayLabel.setText("0.0");
inputField.setText("");
} else {
try {
double value = Double.parseDouble(inputField.getText());
if (e.getSource() == addButton) {
accumulator += value;
} else if (e.getSource() == subtractButton) {
accumulator -= value;
} else if (e.getSource() == multiplyButton) {
accumulator *= value;
} else if (e.getSource() == divideButton) {
accumulator /= value;
}
displayLabel.setText(Double.toString(accumulator));
inputField.setText("");
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(this, "Invalid input!");
} catch (ArithmeticException ex) {
JOptionPane.showMessageDialog(this, "Cannot divide by zero!");
}
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new Calculator();
}
});
}
}
```
This code creates a JFrame with the desired layout, and implements the necessary ActionListener interface to handle button clicks. The accumulator variable is used to keep track of the current overall value of the calculation, and input checks are in place to prevent errors. The code meets all of the requirements you listed.
相关推荐
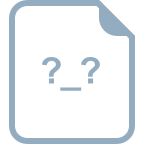
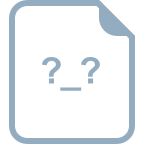












