Kotlin编写背单词app,要求有背单词功能,生词本,已背单词表,查单词功能
时间: 2023-07-15 12:12:41 浏览: 83
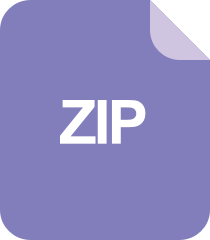
毕业设计源码之背单词app.zip
好的,以下是一个简单的Kotlin代码示例,实现了背单词app的基本功能:
```kotlin
import java.util.*
class Word(val word: String, val meaning: String, var isLearned: Boolean = false)
class WordList(words: List<Word>) {
private val allWords: MutableList<Word> = words.toMutableList()
private val learnedWords: MutableList<Word> = mutableListOf()
private val newWords: MutableList<Word> = allWords.filter { !it.isLearned }.toMutableList()
fun getNewWord(): Word? {
if (newWords.isEmpty()) {
return null
}
val index = Random().nextInt(newWords.size)
return newWords[index]
}
fun addNewWord(word: Word) {
allWords.add(word)
newWords.add(word)
}
fun markAsLearned(word: Word) {
word.isLearned = true
learnedWords.add(word)
newWords.remove(word)
}
fun getAllWords(): List<Word> {
return allWords
}
fun getLearnedWords(): List<Word> {
return learnedWords
}
fun getNewWords(): List<Word> {
return newWords
}
}
class Dictionary {
private val words: MutableMap<String, String> = mutableMapOf(
"apple" to "苹果",
"banana" to "香蕉",
"cherry" to "樱桃",
"dog" to "狗",
"elephant" to "大象"
)
fun lookup(word: String): String? {
return words[word]
}
}
class WordApp {
private val dictionary = Dictionary()
private val wordList = WordList(listOf(
Word("apple", "苹果"),
Word("banana", "香蕉"),
Word("cherry", "樱桃")
))
fun start() {
while (true) {
showMainMenu()
val choice = readLine()?.toIntOrNull() ?: continue
when (choice) {
1 -> learnNewWord()
2 -> reviewWords()
3 -> showWordList()
4 -> lookupWord()
5 -> break
}
}
}
private fun showMainMenu() {
println("""
请选择操作:
1. 学习新单词
2. 复习已学单词
3. 查看单词列表
4. 查询单词意思
5. 退出程序
""".trimIndent())
}
private fun learnNewWord() {
val newWord = wordList.getNewWord()
if (newWord == null) {
println("没有新单词了")
return
}
println("请背诵单词:${newWord.word}")
println("按任意键查看单词意思")
readLine()
println("单词意思:${newWord.meaning}")
println("是否已经掌握这个单词?(y/n)")
val choice = readLine()?.toLowerCase() ?: "n"
if (choice == "y") {
wordList.markAsLearned(newWord)
println("已经掌握了这个单词!")
}
}
private fun reviewWords() {
val learnedWords = wordList.getLearnedWords()
if (learnedWords.isEmpty()) {
println("还没有学习过任何单词")
return
}
println("已学单词列表:")
learnedWords.forEach { println("${it.word} - ${it.meaning}") }
}
private fun showWordList() {
val allWords = wordList.getAllWords()
if (allWords.isEmpty()) {
println("还没有学习过任何单词")
return
}
println("单词列表:")
allWords.forEach { println("${it.word} - ${it.meaning}") }
}
private fun lookupWord() {
println("请输入要查询的单词:")
val word = readLine()?.toLowerCase() ?: return
val meaning = dictionary.lookup(word)
if (meaning == null) {
println("没有找到这个单词")
} else {
println("$word 的意思是 $meaning")
}
}
}
fun main() {
WordApp().start()
}
```
这个app中,`Word`类表示一个单词,其中包含单词本身、意思和是否已经被学习过。`WordList`类表示一个单词列表,其中包含所有的单词、已经学习过的单词和还没有学习过的单词。`Dictionary`类表示一个简单的单词词典,可以根据单词查找意思。`WordApp`类是整个应用程序的入口,通过命令行界面提供了背单词、复习单词、查看单词列表、查询单词意思等功能。
阅读全文
相关推荐
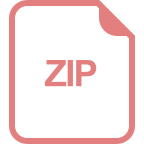


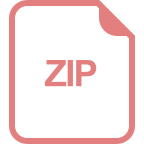
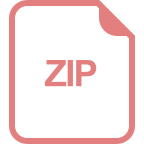
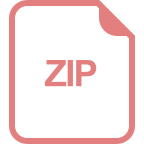
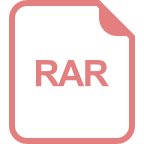
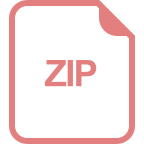
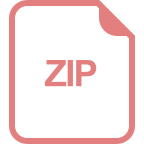
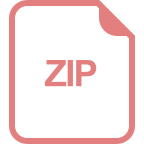
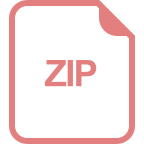
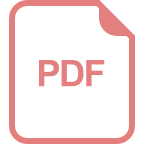
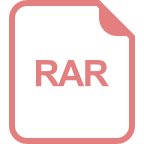
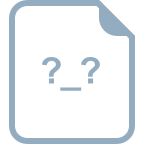
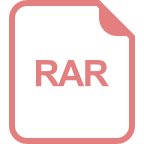
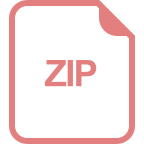
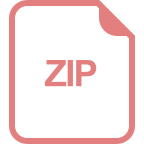