vue实现表格增删改查
时间: 2023-08-31 19:09:30 浏览: 91
Vue可以通过组件化的方式实现表格的增删改查功能,以下是一个简单的示例:
1. 首先,定义一个表格组件 Table.vue:
```html
<template>
<div>
<table>
<thead>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in list" :key="index">
<td>{{ item.name }}</td>
<td>{{ item.age }}</td>
<td>
<button @click="edit(index)">编辑</button>
<button @click="remove(index)">删除</button>
</td>
</tr>
</tbody>
</table>
<button @click="add">新增</button>
<div v-if="showEdit">
<input v-model="editItem.name" placeholder="请输入姓名" />
<input v-model="editItem.age" placeholder="请输入年龄" />
<button @click="save">保存</button>
</div>
</div>
</template>
<script>
export default {
props: {
list: {
type: Array,
default: () => [],
},
},
data() {
return {
showEdit: false,
editItem: {
name: '',
age: '',
},
editIndex: -1,
};
},
methods: {
add() {
this.showEdit = true;
},
edit(index) {
this.showEdit = true;
this.editIndex = index;
this.editItem = { ...this.list[index] };
},
remove(index) {
this.$emit('remove', index);
},
save() {
if (this.editIndex === -1) {
this.$emit('add', this.editItem);
} else {
this.$emit('edit', this.editIndex, this.editItem);
}
this.showEdit = false;
this.editIndex = -1;
this.editItem = { name: '', age: '' };
},
},
};
</script>
```
2. 在父组件中引入 Table 组件,并在 data 中定义一个数组 list 来存储表格数据:
```html
<template>
<div>
<Table :list="list" @add="add" @edit="edit" @remove="remove" />
</div>
</template>
<script>
import Table from './Table.vue';
export default {
components: {
Table,
},
data() {
return {
list: [
{ name: '张三', age: 18 },
{ name: '李四', age: 20 },
{ name: '王五', age: 22 },
],
};
},
methods: {
add(item) {
this.list.push(item);
},
edit(index, item) {
this.list.splice(index, 1, item);
},
remove(index) {
this.list.splice(index, 1);
},
},
};
</script>
```
这样,我们就实现了一个简单的表格增删改查功能。当点击新增或编辑按钮时,会弹出一个对话框,用户可以输入新的数据或修改已有数据,并保存。当点击删除按钮时,会删除对应的数据。
阅读全文
相关推荐
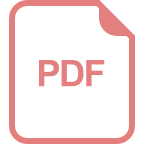
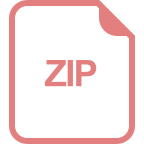
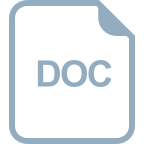
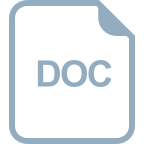
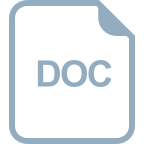
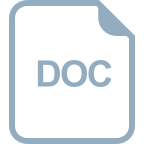
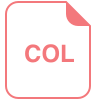






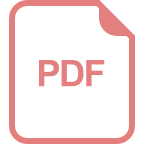