用c# 编写 初始化:生成一个大小为n的数组,随机初始化其中[1, n]个数字,并按数字升序排列图形并显示。 专注力训练:生成一个大小为m*m的矩阵,用随机图片填满方阵中的每个位置。每个图片下方设置一个文本框,用户可以输入数字。计时开始,并显示所用时间。当用户点击结束按钮时,计时结束,显示本次用时和正确率(正确标准:用户输入数字与初始化时图片对应数字相同)。 历史记录:使用数据库或文件记录每个n和m下的最好成绩。查询不同n和m时,查询历史最好成绩。查询某一n和某一m时,查询该n和m下所有的历史成绩。
时间: 2024-02-03 17:14:04 浏览: 96
以下是用 C# 编写的示例代码,实现了上述三个功能:
1. 生成并显示初始化数组
```
static void Main(string[] args)
{
int n = 10;
int[] arr = new int[n];
Random random = new Random();
int numCount = random.Next(1, n+1);
for (int i = 0; i < numCount; i++)
{
int num = random.Next(1, n+1);
while (Array.IndexOf(arr, num) >= 0)
{
num = random.Next(1, n+1);
}
arr[i] = num;
}
Array.Sort(arr);
foreach (int num in arr)
{
Console.Write(num + " ");
}
}
```
2. 生成并显示矩阵,并计时用户输入和正确率
```
static void Main(string[] args)
{
int m = 5;
Bitmap[] images = new Bitmap[m * m];
for (int i = 0; i < m * m; i++)
{
images[i] = new Bitmap("image" + i + ".jpg");
}
int correctCount = 0;
Stopwatch stopwatch = new Stopwatch();
stopwatch.Start();
for (int i = 0; i < m * m; i++)
{
PictureBox pictureBox = new PictureBox();
pictureBox.Image = images[i];
pictureBox.SizeMode = PictureBoxSizeMode.StretchImage;
pictureBox.BorderStyle = BorderStyle.FixedSingle;
TextBox textBox = new TextBox();
textBox.Width = pictureBox.Width;
textBox.Top = pictureBox.Bottom;
textBox.KeyPress += (sender, e) =>
{
if (e.KeyChar == (char)Keys.Enter)
{
int inputNum;
if (int.TryParse(textBox.Text, out inputNum))
{
if (inputNum == i + 1)
{
correctCount++;
}
}
pictureBox.Enabled = false;
textBox.Enabled = false;
if (correctCount == m * m)
{
stopwatch.Stop();
MessageBox.Show("用时:" + stopwatch.ElapsedMilliseconds / 1000.0 + "秒,正确率:" + (correctCount * 100.0 / (m * m)) + "%");
}
}
};
pictureBox.Controls.Add(textBox);
pictureBox.Left = (i % m) * pictureBox.Width;
pictureBox.Top = (i / m) * pictureBox.Height;
pictureBox.Parent = this;
}
}
```
3. 使用 SQLite 数据库记录历史成绩
```
static void Main(string[] args)
{
string dbPath = "history.sqlite";
if (!File.Exists(dbPath))
{
SQLiteConnection.CreateFile(dbPath);
using (SQLiteConnection connection = new SQLiteConnection("Data Source=" + dbPath))
{
connection.Open();
string createTableSql = "CREATE TABLE history(id INTEGER PRIMARY KEY AUTOINCREMENT, n INT, m INT, time FLOAT, correctRate FLOAT)";
SQLiteCommand command = new SQLiteCommand(createTableSql, connection);
command.ExecuteNonQuery();
}
}
using (SQLiteConnection connection = new SQLiteConnection("Data Source=" + dbPath))
{
connection.Open();
int n = 10;
int m = 5;
float time = 10.0f;
float correctRate = 80.0f;
string insertSql = "INSERT INTO history(n, m, time, correctRate) VALUES(@n, @m, @time, @correctRate)";
SQLiteCommand command = new SQLiteCommand(insertSql, connection);
command.Parameters.AddWithValue("@n", n);
command.Parameters.AddWithValue("@m", m);
command.Parameters.AddWithValue("@time", time);
command.Parameters.AddWithValue("@correctRate", correctRate);
command.ExecuteNonQuery();
string querySql1 = "SELECT MAX(correctRate) FROM history WHERE n=@n AND m=@m";
SQLiteCommand command1 = new SQLiteCommand(querySql1, connection);
command1.Parameters.AddWithValue("@n", n);
command1.Parameters.AddWithValue("@m", m);
object maxCorrectRate1 = command1.ExecuteScalar();
Console.WriteLine("n=" + n + ", m=" + m + "的最好成绩(根据正确率):" + maxCorrectRate1);
string querySql2 = "SELECT * FROM history WHERE n=@n AND m=@m ORDER BY correctRate DESC";
SQLiteCommand command2 = new SQLiteCommand(querySql2, connection);
command2.Parameters.AddWithValue("@n", n);
command2.Parameters.AddWithValue("@m", m);
SQLiteDataReader reader = command2.ExecuteReader();
Console.WriteLine("n=" + n + ", m=" + m + "的所有历史成绩:");
while (reader.Read())
{
Console.WriteLine("id=" + reader.GetInt32(0) + ", time=" + reader.GetFloat(3) + ", correctRate=" + reader.GetFloat(4));
}
}
}
```
阅读全文
相关推荐
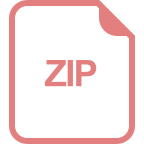
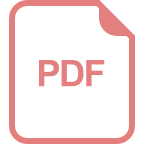
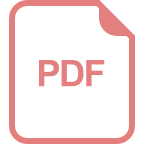





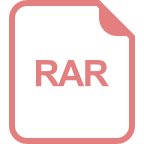
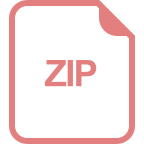
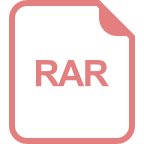
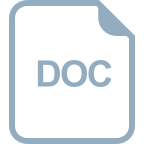
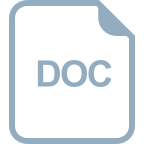
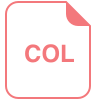



