用uniapp的uni.requireNativePlugin('bindingx')插件,写个拖动,缩小,放大页面,用的页面是nvue
时间: 2024-02-06 10:09:01 浏览: 34
好的,下面是一个简单的示例代码:
1. 在 pages.json 中添加插件配置:
```json
{
"pages": [
{
"path": "pages/index/index",
"style": {
"navigationBarTitleText": "首页"
},
"usingComponents": {
"van-button": "/static/vant/button/index"
},
"uni": {
"enablePullDownRefresh": true,
"disableScroll": false,
"disableSwipeBack": false,
"pageOrientation": "portrait",
"backgroundColor": "#f8f8f8",
"pullRefreshOptions": {
"style": "default",
"textColor": "#000000",
"refreshingText": "正在刷新...",
"completeText": "刷新完成",
"failText": "刷新失败"
},
"navigationBarBackgroundColor": "#ffffff",
"navigationBarTextStyle": "black",
"navigationBarTitleText": "uni-app",
"backgroundTextStyle": "dark",
"backgroundColorTop": "#ffffff",
"backgroundColorBottom": "#ffffff",
"usingComponents": {
"van-button": "/static/vant/button/index"
},
"plugins": {
"bindingx": {
"version": "1.0.0",
"provider": "dcloudio.unipluginbindingx"
}
}
}
}
]
}
```
2. 在 index.nvue 中添加一个可拖动的元素:
```html
<template>
<view class="box" :style="{ transform: `translate3d(${x}px, ${y}px, 0) scale(${scale})` }">
<text>我可以拖动哦~~~</text>
</view>
</template>
<style>
.box {
position: absolute;
width: 200px;
height: 200px;
background-color: #f1f1f1;
border: 1px solid #ccc;
font-size: 18px;
text-align: center;
line-height: 200px;
border-radius: 10px;
user-select: none;
-webkit-user-select: none;
-webkit-touch-callout: none;
}
</style>
```
3. 在 index.js 中添加拖动和缩放的逻辑:
```javascript
import Vue from 'vue';
const bindingx = uni.requireNativePlugin('bindingx');
Vue.mixin({
mounted() {
this.bindEvent();
},
methods: {
bindEvent() {
const box = this.$refs.box;
const start = { x: 0, y: 0 };
let lastX = 0;
let lastY = 0;
let lastScale = 1;
let isMove = false;
box.addEventListener('touchstart', (event) => {
const touches = event.touches;
if (touches.length === 1) {
const touch = touches[0];
start.x = touch.pageX;
start.y = touch.pageY;
} else {
isMove = true;
const touch1 = touches[0];
const touch2 = touches[1];
lastX = (touch1.pageX + touch2.pageX) / 2;
lastY = (touch1.pageY + touch2.pageY) / 2;
lastScale = this.scale;
}
});
box.addEventListener('touchmove', (event) => {
const touches = event.touches;
if (touches.length === 1 && !isMove) {
const touch = touches[0];
const moveX = touch.pageX - start.x;
const moveY = touch.pageY - start.y;
box.style.transform = `translate3d(${moveX}px, ${moveY}px, 0) scale(${this.scale})`;
} else if (touches.length === 2) {
isMove = true;
const touch1 = touches[0];
const touch2 = touches[1];
const x = (touch1.pageX + touch2.pageX) / 2;
const y = (touch1.pageY + touch2.pageY) / 2;
const deltaX = x - lastX;
const deltaY = y - lastY;
const scale =
lastScale *
Math.sqrt(
(touch1.pageX - touch2.pageX) *
(touch1.pageX - touch2.pageX) +
(touch1.pageY - touch2.pageY) *
(touch1.pageY - touch2.pageY)
) /
Math.sqrt(
(event.touches[0].pageX - event.touches[1].pageX) *
(event.touches[0].pageX - event.touches[1].pageX) +
(event.touches[0].pageY - event.touches[1].pageY) *
(event.touches[0].pageY - event.touches[1].pageY)
);
this.scale = scale;
box.style.transform = `translate3d(${deltaX}px, ${deltaY}px, 0) scale(${scale})`;
}
});
box.addEventListener('touchend', () => {
if (!isMove) {
bindingx.create({
eventType: 'pan',
selector: '._bindingx_box_',
props: [
{
element: 'transform.translateX',
expression: 'x'
},
{
element: 'transform.translateY',
expression: 'y'
}
]
}).interact(this.$refs.box, {
startX: 0,
startY: 0,
touchAction: 'pan-y',
animation: {
duration: 1000
}
});
}
isMove = false;
});
}
},
data() {
return {
x: 0,
y: 0,
scale: 1
};
}
});
export default {
methods: {
onClick() {
console.log('click');
}
}
};
```
这样,就可以实现一个简单的拖动、缩放页面元素的功能了。注意,这里使用了 `bindingx` 插件来实现拖动动画效果,需要在 `touchend` 事件中检测拖动距离,如果没有拖动,则触发 `bindingx` 动画效果。
相关推荐
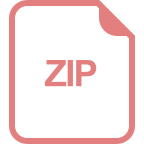
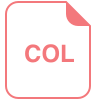
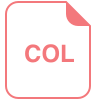
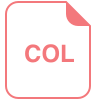
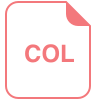
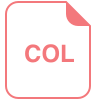









