现有系统参考代码
时间: 2023-08-04 18:03:21 浏览: 110
当然可以,以下是一个简单的图书管理系统的Python代码示例,供您参考:
```python
import sqlite3
from tkinter import *
from tkinter import messagebox
# 连接数据库
conn = sqlite3.connect('book.db')
c = conn.cursor()
# 创建表
c.execute('''CREATE TABLE IF NOT EXISTS book
(id INTEGER PRIMARY KEY AUTOINCREMENT,
title TEXT NOT NULL,
author TEXT NOT NULL,
isbn TEXT NOT NULL,
publisher TEXT NOT NULL,
price REAL NOT NULL)''')
c.execute('''CREATE TABLE IF NOT EXISTS borrow
(id INTEGER PRIMARY KEY AUTOINCREMENT,
book_id INTEGER NOT NULL,
borrower TEXT NOT NULL,
borrow_date TEXT NOT NULL,
return_date TEXT)''')
# 添加图书
def add_book():
title = title_entry.get()
author = author_entry.get()
isbn = isbn_entry.get()
publisher = publisher_entry.get()
price = price_entry.get()
c.execute("INSERT INTO book (title, author, isbn, publisher, price) VALUES (?, ?, ?, ?, ?)", (title, author, isbn, publisher, price))
conn.commit()
messagebox.showinfo('Success', 'Book added successfully')
# 查询图书
def search_book():
keyword = search_entry.get()
c.execute("SELECT * FROM book WHERE title LIKE '%{}%' OR author LIKE '%{}%' OR isbn LIKE '%{}%'".format(keyword, keyword, keyword))
books = c.fetchall()
for book in books:
result_text.insert(END, 'Title: {}\nAuthor: {}\nISBN: {}\nPublisher: {}\nPrice: {}\n\n'.format(book[1], book[2], book[3], book[4], book[5]))
# 借阅图书
def borrow_book():
book_id = book_id_entry.get()
borrower = borrower_entry.get()
borrow_date = borrow_date_entry.get()
c.execute("INSERT INTO borrow (book_id, borrower, borrow_date) VALUES (?, ?, ?)", (book_id, borrower, borrow_date))
conn.commit()
messagebox.showinfo('Success', 'Book borrowed successfully')
# 归还图书
def return_book():
borrow_id = borrow_id_entry.get()
return_date = return_date_entry.get()
c.execute("UPDATE borrow SET return_date = ? WHERE id = ?", (return_date, borrow_id))
conn.commit()
messagebox.showinfo('Success', 'Book returned successfully')
# GUI界面
root = Tk()
root.title('图书管理系统')
# 添加图书
title_label = Label(root, text='Title:')
title_label.grid(row=0, sticky=W)
title_entry = Entry(root)
title_entry.grid(row=0, column=1)
author_label = Label(root, text='Author:')
author_label.grid(row=1, sticky=W)
author_entry = Entry(root)
author_entry.grid(row=1, column=1)
isbn_label = Label(root, text='ISBN:')
isbn_label.grid(row=2, sticky=W)
isbn_entry = Entry(root)
isbn_entry.grid(row=2, column=1)
publisher_label = Label(root, text='Publisher:')
publisher_label.grid(row=3, sticky=W)
publisher_entry = Entry(root)
publisher_entry.grid(row=3, column=1)
price_label = Label(root, text='Price:')
price_label.grid(row=4, sticky=W)
price_entry = Entry(root)
price_entry.grid(row=4, column=1)
add_button = Button(root, text='Add Book', command=add_book)
add_button.grid(row=5, column=1)
# 查询图书
search_label = Label(root, text='Search:')
search_label.grid(row=6, sticky=W)
search_entry = Entry(root)
search_entry.grid(row=6, column=1)
search_button = Button(root, text='Search', command=search_book)
search_button.grid(row=6, column=2)
result_text = Text(root, width=50, height=10)
result_text.grid(row=7, columnspan=3)
# 借阅图书
book_id_label = Label(root, text='Book ID:')
book_id_label.grid(row=8, sticky=W)
book_id_entry = Entry(root)
book_id_entry.grid(row=8, column=1)
borrower_label = Label(root, text='Borrower:')
borrower_label.grid(row=9, sticky=W)
borrower_entry = Entry(root)
borrower_entry.grid(row=9, column=1)
borrow_date_label = Label(root, text='Borrow Date:')
borrow_date_label.grid(row=10, sticky=W)
borrow_date_entry = Entry(root)
borrow_date_entry.grid(row=10, column=1)
borrow_button = Button(root, text='Borrow', command=borrow_book)
borrow_button.grid(row=11, column=1)
# 归还图书
borrow_id_label = Label(root, text='Borrow ID:')
borrow_id_label.grid(row=12, sticky=W)
borrow_id_entry = Entry(root)
borrow_id_entry.grid(row=12, column=1)
return_date_label = Label(root, text='Return Date:')
return_date_label.grid(row=13, sticky=W)
return_date_entry = Entry(root)
return_date_entry.grid(row=13, column=1)
return_button = Button(root, text='Return', command=return_book)
return_button.grid(row=14, column=1)
root.mainloop()
```
这段代码实现了基本的图书添加、查询、借阅归还等功能,您可以根据您的实际需求进行修改和扩展。
阅读全文
相关推荐
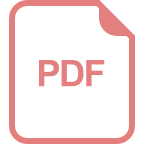
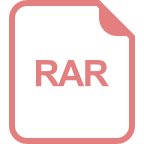
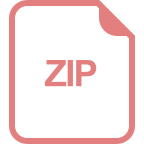
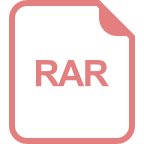
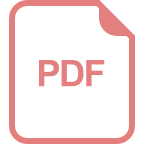
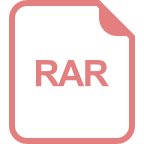
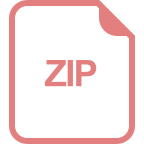
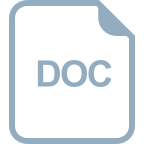
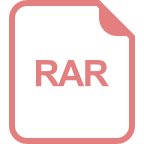
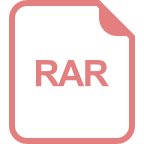
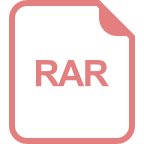
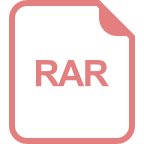
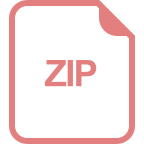
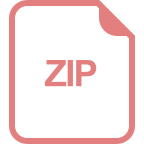
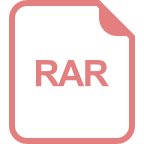
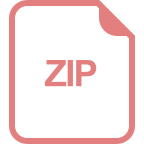