调用函数fun((),其功能是用选择法对10个整数按降序进行排序。
时间: 2024-05-13 18:16:22 浏览: 35
以下是用选择法对10个整数按降序进行排序的示例代码:
```python
def fun(nums):
n = len(nums)
for i in range(n):
max_idx = i
for j in range(i+1, n):
if nums[j] > nums[max_idx]:
max_idx = j
nums[i], nums[max_idx] = nums[max_idx], nums[i]
return nums
nums = [3, 7, 1, 9, 2, 8, 10, 5, 4, 6]
sorted_nums = fun(nums)
print(sorted_nums)
```
运行结果为:
```
[10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
```
其中,`fun`函数的参数为待排序的整数列表 `nums`,函数内部使用选择法对 `nums` 进行排序,并返回排序后的结果。在 `for` 循环中,首先定义 `max_idx` 为当前循环的起始位置 `i`,然后从 `i+1` 开始遍历整个列表,找到最大值所在的位置,将该位置的元素与起始位置上的元素进行交换。这样,每一次循环都能将当前未排序部分的最大值放到已排序部分的最前面,最终得到降序排列的整数列表。
相关问题
c语言 调用函数fun 其功能是用选择法对10个整数按降序进行排序
可以像这样编写一个函数fun来实现选择排序:
```c
void fun(int arr[], int n) {
int i, j, max_idx;
for (i = 0; i < n-1; i++) {
max_idx = i;
for (j = i+1; j < n; j++) {
if (arr[j] > arr[max_idx]) {
max_idx = j;
}
}
// 交换 arr[i] 和 arr[max_idx]
int temp = arr[i];
arr[i] = arr[max_idx];
arr[max_idx] = temp;
}
}
```
其中,`arr` 是包含 10 个整数的数组,`n` 是数组的长度。调用 `fun(arr, 10)` 就可以按降序对数组进行排序了。
c语言调用函数fun其功能是用选择法对10个整数按降序排序
以下是一个使用选择排序算法对10个整数按降序排序的C语言函数fun的实现:
```c
void fun(int arr[], int n) {
int i, j, temp, max_index;
for (i = 0; i < n - 1; i++) {
max_index = i;
for (j = i + 1; j < n; j++) {
if (arr[j] > arr[max_index]) {
max_index = j;
}
}
if (max_index != i) {
temp = arr[i];
arr[i] = arr[max_index];
arr[max_index] = temp;
}
}
}
```
该函数的参数包括一个包含10个整数的数组和数组的长度n。在函数内部,我们使用选择排序算法对数组进行降序排序。首先,我们从数组的第一个元素开始,找到数组中最大的元素,并将其与第一个元素交换。然后,我们从第二个元素开始,找到剩余元素中最大的元素,并将其与第二个元素交换。重复此过程,直到整个数组被排序。
相关推荐
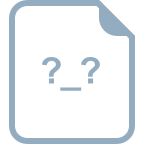
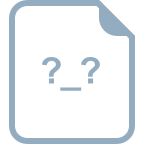
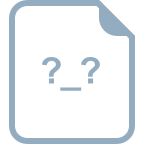












