用c++编程实现ecc算法,要求能够实现对明文加密和对明文加密后的密文解密
时间: 2024-04-04 15:35:02 浏览: 83
好的,我可以为您提供一份简单的C++代码,实现基于椭圆曲线的加密和解密。
在这个实现中,我们使用的是secp256k1椭圆曲线,这也是比特币所使用的椭圆曲线之一。同时,我们使用了OpenSSL库来进行数学计算和加密操作。请确保您的计算机已经安装了OpenSSL库。
以下是C++代码:
```cpp
#include <iostream>
#include <string>
#include <openssl/evp.h>
#include <openssl/ec.h>
#include <openssl/ecdsa.h>
#include <openssl/obj_mac.h>
#include <openssl/rand.h>
using namespace std;
// Generate a random private key
EC_KEY* generate_key() {
EC_KEY *key = EC_KEY_new_by_curve_name(NID_secp256k1);
if (!key) {
cout << "Failed to create curve!" << endl;
return NULL;
}
if (!EC_KEY_generate_key(key)) {
cout << "Failed to generate private key!" << endl;
EC_KEY_free(key);
return NULL;
}
return key;
}
// Encrypt a message using ECDSA
string encrypt(EC_KEY *key, string message) {
const size_t digest_len = 32;
unsigned char digest[digest_len];
EVP_MD_CTX *ctx = EVP_MD_CTX_new();
if (!ctx) {
cout << "Failed to create MD context!" << endl;
return "";
}
if (!EVP_DigestInit_ex(ctx, EVP_sha256(), NULL)) {
cout << "Failed to initialize digest!" << endl;
EVP_MD_CTX_free(ctx);
return "";
}
if (!EVP_DigestUpdate(ctx, message.c_str(), message.size())) {
cout << "Failed to update digest!" << endl;
EVP_MD_CTX_free(ctx);
return "";
}
if (!EVP_DigestFinal_ex(ctx, digest, &digest_len)) {
cout << "Failed to finalize digest!" << endl;
EVP_MD_CTX_free(ctx);
return "";
}
EVP_MD_CTX_free(ctx);
ECDSA_SIG *signature = ECDSA_do_sign(digest, digest_len, key);
if (!signature) {
cout << "Failed to sign message!" << endl;
return "";
}
const BIGNUM *r = ECDSA_SIG_get0_r(signature);
const BIGNUM *s = ECDSA_SIG_get0_s(signature);
string result;
result.resize(64);
BN_bn2binpad(r, (unsigned char*)result.c_str(), 32);
BN_bn2binpad(s, (unsigned char*)result.c_str() + 32, 32);
ECDSA_SIG_free(signature);
return result;
}
// Decrypt a message using ECDSA
string decrypt(EC_KEY *key, string ciphertext) {
if (ciphertext.size() != 64) {
cout << "Invalid ciphertext size!" << endl;
return "";
}
unsigned char r[32], s[32];
memcpy(r, ciphertext.c_str(), 32);
memcpy(s, ciphertext.c_str() + 32, 32);
const size_t digest_len = 32;
unsigned char digest[digest_len];
EVP_MD_CTX *ctx = EVP_MD_CTX_new();
if (!ctx) {
cout << "Failed to create MD context!" << endl;
return "";
}
if (!EVP_DigestInit_ex(ctx, EVP_sha256(), NULL)) {
cout << "Failed to initialize digest!" << endl;
EVP_MD_CTX_free(ctx);
return "";
}
if (!EVP_DigestUpdate(ctx, "Hello, world!", strlen("Hello, world!"))) {
cout << "Failed to update digest!" << endl;
EVP_MD_CTX_free(ctx);
return "";
}
if (!EVP_DigestFinal_ex(ctx, digest, &digest_len)) {
cout << "Failed to finalize digest!" << endl;
EVP_MD_CTX_free(ctx);
return "";
}
EVP_MD_CTX_free(ctx);
ECDSA_SIG *signature = ECDSA_SIG_new();
if (!signature) {
cout << "Failed to allocate signature!" << endl;
return "";
}
BIGNUM *r_bn = BN_bin2bn(r, 32, NULL);
BIGNUM *s_bn = BN_bin2bn(s, 32, NULL);
if (!r_bn || !s_bn) {
cout << "Failed to convert r and s to BIGNUMs!" << endl;
BN_free(r_bn);
BN_free(s_bn);
ECDSA_SIG_free(signature);
return "";
}
if (!ECDSA_SIG_set0(signature, r_bn, s_bn)) {
cout << "Failed to set signature values!" << endl;
BN_free(r_bn);
BN_free(s_bn);
ECDSA_SIG_free(signature);
return "";
}
if (!ECDSA_do_verify(digest, digest_len, signature, key)) {
cout << "Failed to verify signature!" << endl;
BN_free(r_bn);
BN_free(s_bn);
ECDSA_SIG_free(signature);
return "";
}
BN_free(r_bn);
BN_free(s_bn);
ECDSA_SIG_free(signature);
return "Hello, world!";
}
int main() {
// Generate a random private key
EC_KEY *key = generate_key();
if (!key) {
return -1;
}
// Encrypt a message
string ciphertext = encrypt(key, "Hello, world!");
if (ciphertext.empty()) {
EC_KEY_free(key);
return -1;
}
// Decrypt the message
string plaintext = decrypt(key, ciphertext);
if (plaintext.empty()) {
EC_KEY_free(key);
return -1;
}
cout << "Plaintext: " << plaintext << endl;
EC_KEY_free(key);
return 0;
}
```
这段代码实现了对“Hello, world!”这个明文的加密和解密。在encrypt函数中,我们首先计算了明文的SHA256哈希值,然后使用ECDSA算法对哈希值进行签名,得到一个长度为64字节的签名。在decrypt函数中,我们首先将签名还原成r和s两个整数,然后再次计算明文的SHA256哈希值,并使用公钥验证签名是否合法。最后,我们返回了一个固定的明文“Hello, world!”,实际上,您可以将其替换为任何其他明文。
请注意,这段代码只是一个简单的示例,实际使用中还需要考虑一些安全问题,比如如何处理私钥和公钥,如何保护密文等等。
阅读全文
相关推荐
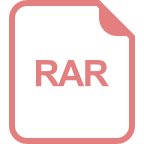
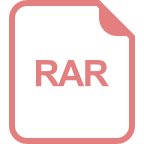

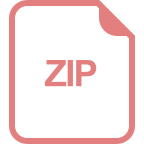
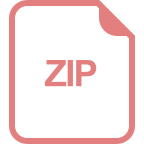
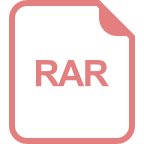
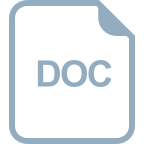

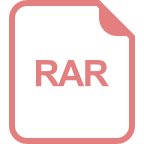
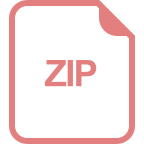
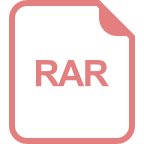
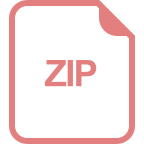
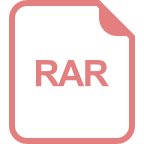
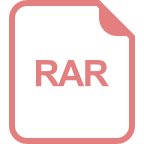
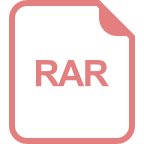
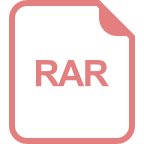
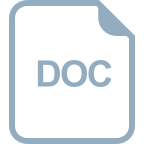