用c语言
时间: 2024-02-16 13:00:22 浏览: 47
以下是一个用C语言实现的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_N 50
typedef struct {
char id[6];
int month;
int day;
} Student;
int cmp(const void *a, const void *b) {
Student *s1 = (Student *) a;
Student *s2 = (Student *) b;
if (s1->month != s2->month) {
return s1->month - s2->month;
}
return s1->day - s2->day;
}
void Count() {
// 读取数据
int n;
scanf("%d", &n);
Student students[MAX_N];
for (int i = 0; i < n; i++) {
scanf("%s %d %d", students[i].id, &students[i].month, &students[i].day);
}
// 统计生日相同的学生信息
qsort(students, n, sizeof(Student), cmp);
int prev_month = -1, prev_day = -1;
for (int i = 0; i < n; i++) {
if (students[i].month != prev_month || students[i].day != prev_day) {
printf("%02d %02d", students[i].month, students[i].day);
prev_month = students[i].month;
prev_day = students[i].day;
}
printf(" %s", students[i].id);
}
printf("\n");
}
int main() {
Count();
return 0;
}
```
此C语言代码中,我们依然是先读取数据,然后使用qsort函数(C语言标准库提供的快速排序函数)按日期排序。最后按日期顺序输出生日相同的学生信息。
阅读全文
相关推荐
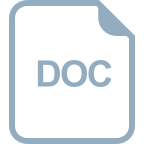
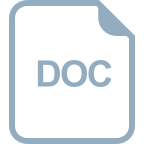
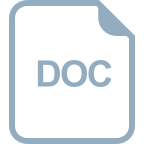
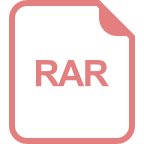
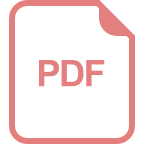