2.掌握字符串的基础操作: (1) 字符串的输入、输出; (2) 统计给定字符串的字符数; (3) 查找指定字符; (4) 插入字符; (5) 删除指定字符; (6) 替换字符; (7) 模式匹配BF算法。
时间: 2023-11-26 07:03:07 浏览: 194
1. 字符串的输入、输出
在C++中,可以使用cin和cout进行字符串的输入输出,也可以使用getline()函数进行输入,使用cout或printf()函数进行输出。
示例:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str;
// 使用cin进行输入
cin >> str;
cout << "使用cin进行输入,输出结果为:" << str << endl;
cin.get(); // 用于清空cin缓存,避免getline()函数受到影响
// 使用getline()函数进行输入
getline(cin, str);
cout << "使用getline()函数进行输入,输出结果为:" << str << endl;
// 使用cout进行输出
cout << "使用cout进行输出,输出结果为:" << str << endl;
// 使用printf()函数进行输出
printf("使用printf()函数进行输出,输出结果为:%s\n", str.c_str());
return 0;
}
```
2. 统计给定字符串的字符数
可以使用string类的size()函数或length()函数获取字符串长度。
示例:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "hello world";
int len = str.size(); // 或者使用 str.length()
cout << "字符串\"" << str << "\"的长度为:" << len << endl;
return 0;
}
```
3. 查找指定字符
可以使用string类的find()函数查找字符串中是否包含指定字符,并返回第一个匹配的位置;如果没有匹配,则返回string::npos。
示例:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "hello world";
char ch = 'l';
int pos = str.find(ch);
if (pos != string::npos) {
cout << "字符串\"" << str << "\"中包含字符'" << ch << "',位置为:" << pos << endl;
} else {
cout << "字符串\"" << str << "\"中不包含字符'" << ch << "'" << endl;
}
return 0;
}
```
4. 插入字符
可以使用string类的insert()函数在指定位置插入一个字符。
示例:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "hello world";
char ch = '!';
int pos = 5;
str.insert(pos, 1, ch);
cout << "在字符串\"" << str << "\"的第" << pos << "个字符位置插入'" << ch << "'后,得到的字符串为:" << str << endl;
return 0;
}
```
5. 删除指定字符
可以使用string类的erase()函数删除字符串中的指定字符。
示例:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "hello world";
char ch = 'l';
int pos = str.find(ch);
if (pos != string::npos) {
str.erase(pos, 1);
cout << "删除字符串\"" << str << "\"中的字符'" << ch << "'后,得到的字符串为:" << str << endl;
} else {
cout << "字符串\"" << str << "\"中不包含字符'" << ch << "'" << endl;
}
return 0;
}
```
6. 替换字符
可以使用string类的replace()函数替换字符串中的指定字符。
示例:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "hello world";
char ch1 = 'l', ch2 = 'L';
int pos = str.find(ch1);
if (pos != string::npos) {
str.replace(pos, 1, 1, ch2);
cout << "替换字符串\"" << str << "\"中的字符'" << ch1 << "'为'" << ch2 << "'后,得到的字符串为:" << str << endl;
} else {
cout << "字符串\"" << str << "\"中不包含字符'" << ch1 << "'" << endl;
}
return 0;
}
```
7. 模式匹配BF算法
BF算法(Brute-Force算法),也称朴素匹配算法,是一种简单的字符串匹配算法,思想是从主串的第一个字符开始,依次和模式串的每个字符比较,如果出现不匹配,则主串指针后移一位,继续匹配下一个字符。
示例:
```c++
#include <iostream>
#include <string>
using namespace std;
int BF(string s, string p) {
int n = s.size(), m = p.size();
for (int i = 0; i <= n - m; i++) {
bool flag = true;
for (int j = 0; j < m; j++) {
if (s[i+j] != p[j]) {
flag = false;
break;
}
}
if (flag) return i;
}
return -1;
}
int main() {
string s = "hello world";
string p = "world";
int pos = BF(s, p);
if (pos != -1) {
cout << "字符串\"" << s << "\"中包含模式串\"" << p << "\",位置为:" << pos << endl;
} else {
cout << "字符串\"" << s << "\"中不包含模式串\"" << p << "\"" << endl;
}
return 0;
}
```
阅读全文
相关推荐
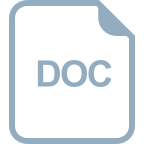
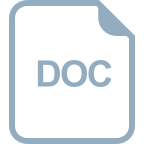
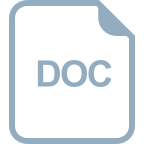
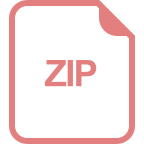
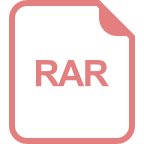
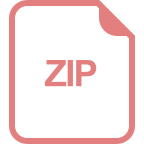
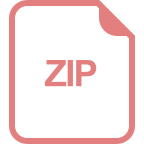
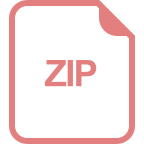
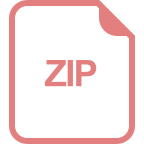
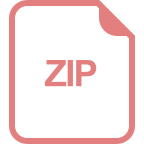
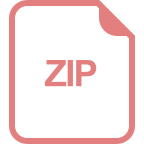
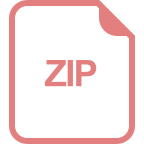
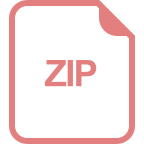
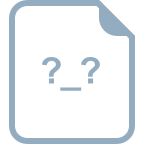
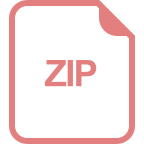
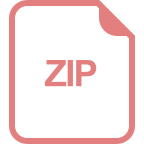
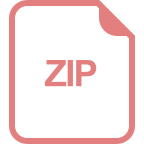