基于easyexcel的excel工具类
时间: 2023-09-08 07:14:44 浏览: 104
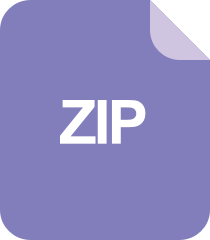
easyExcel 工具类
EasyExcel 是一个基于阿里巴巴开源的工具 EasyPOI,专为解决Java中操作 Excel 的问题而生的。它提供了简单易用的 Java API,可以方便地读写 Excel 文件,并支持大批量数据的导入导出。
以下是一个基于 EasyExcel 的 Excel 工具类示例:
```java
import com.alibaba.excel.EasyExcel;
import com.alibaba.excel.ExcelReader;
import com.alibaba.excel.ExcelWriter;
import com.alibaba.excel.context.AnalysisContext;
import com.alibaba.excel.event.AnalysisEventListener;
import com.alibaba.excel.metadata.Sheet;
import com.alibaba.excel.metadata.Table;
import com.alibaba.excel.support.ExcelTypeEnum;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellType;
import org.apache.poi.ss.usermodel.Row;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
public class ExcelUtil {
/**
* 读取 Excel 文件数据,返回 List 列表
*
* @param inputStream Excel 文件输入流
* @param sheetNo 工作表序号(从 0 开始)
* @param headLineNum 标题行数(默认为 1)
* @return List 列表
*/
public static List<List<String>> readExcel(InputStream inputStream, int sheetNo, int headLineNum) {
List<List<String>> list = new ArrayList<>();
ExcelReader excelReader = EasyExcel.read(inputStream).build();
try {
Sheet sheet = new Sheet(sheetNo, headLineNum);
excelReader.read(sheet);
} finally {
excelReader.finish();
}
return list;
}
/**
* 读取 Excel 文件数据,并通过回调函数处理每行数据
*
* @param inputStream Excel 文件输入流
* @param sheetNo 工作表序号(从 0 开始)
* @param headLineNum 标题行数(默认为 1)
* @param listener 回调函数
*/
public static void readExcel(InputStream inputStream, int sheetNo, int headLineNum,
AnalysisEventListener<Map<Integer, String>> listener) {
ExcelReader excelReader = EasyExcel.read(inputStream, listener).build();
try {
Sheet sheet = new Sheet(sheetNo, headLineNum);
excelReader.read(sheet);
} finally {
excelReader.finish();
}
}
/**
* 写入 Excel 文件数据,返回写入的行数
*
* @param outputStream Excel 文件输出流
* @param sheetNo 工作表序号(从 0 开始)
* @param head 表头数据列表
* @param dataList 数据列表
* @return 写入的行数
*/
public static int writeExcel(OutputStream outputStream, int sheetNo, List<String> head, List<List<Object>> dataList) {
ExcelWriter excelWriter = EasyExcel.write(outputStream).build();
try {
Sheet sheet = new Sheet(sheetNo, 0);
Table table = new Table(0);
List<List<String>> headList = new ArrayList<>();
headList.add(head);
table.setHead(headList);
excelWriter.write1(dataList, sheet, table);
return dataList.size();
} finally {
excelWriter.finish();
}
}
/**
* 获取单元格的值
*
* @param cell 单元格
* @return 单元格的值
*/
public static Object getCellValue(Cell cell) {
if (cell == null) {
return null;
}
CellType cellType = cell.getCellType();
switch (cellType) {
case BLANK:
return null;
case BOOLEAN:
return cell.getBooleanCellValue();
case ERROR:
return cell.getErrorCellValue();
case FORMULA:
return cell.getCellFormula();
case NUMERIC:
return cell.getNumericCellValue();
case STRING:
return cell.getStringCellValue();
default:
return null;
}
}
}
```
该工具类提供了 Excel 文件的读取和写入功能,并支持大批量数据的导入导出。可以通过调用 `readExcel()` 方法读取 Excel 文件数据,并通过回调函数处理每行数据;也可以通过调用 `writeExcel()` 方法将数据写入 Excel 文件中。`getCellValue()` 方法可以获取单元格的值。
阅读全文
相关推荐
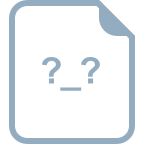
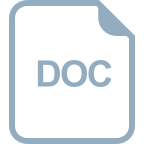














