int C[]={11,1,89}从小到大排序
时间: 2023-12-17 22:02:26 浏览: 25
好的,这里提供一个简单的选择排序算法示例,可以对整型数组进行从小到大排序:
```
void selectionSort(int arr[], int len) {
int i, j, minIndex, tmp;
for (i = 0; i < len - 1; i++) {
minIndex = i;
for (j = i + 1; j < len; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
if (minIndex != i) {
tmp = arr[i];
arr[i] = arr[minIndex];
arr[minIndex] = tmp;
}
}
}
```
使用方法:
```
int main() {
int C[] = {11, 1, 89};
int len = sizeof(C) / sizeof(int);
selectionSort(C, len);
for (int i = 0; i < len; i++) {
printf("%d ", C[i]);
}
return 0;
}
```
这段代码会输出排好序的数组,即 `1 11 89`。
相关问题
int C[]={11,1,89,7,36,}从小到大排序
好的,这里提供一个简单的冒泡排序算法示例,可以对整型数组进行从小到大排序:
```
void bubbleSort(int arr[], int len) {
int i, j, tmp;
for (i = 0; i < len - 1; i++) {
for (j = 0; j < len - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
tmp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = tmp;
}
}
}
}
```
使用方法:
```
int main() {
int C[] = {11, 1, 89, 7, 36};
int len = sizeof(C) / sizeof(int);
bubbleSort(C, len);
for (int i = 0; i < len; i++) {
printf("%d ", C[i]);
}
return 0;
}
```
这段代码会输出排好序的数组,即 `1 7 11 36 89`。
int型从小到大排序 c/c++语言
在 C 或 C++ 中,可以使用 qsort 函数对数组中的元素进行排序。qsort 函数需要提供一个比较函数,用于指定如何比较两个元素的大小。
以下是一个示例,展示了如何使用 qsort 函数对 int 型数组进行从小到大排序:
```
#include <stdio.h>
#include <stdlib.h>
int compare(const void *a, const void *b) {
// 将指针转换回原类型,并比较它们的值
int x = *(int *)a;
int y = *(int *)b;
if (x < y) {
return -1;
} else if (x > y) {
return 1;
} else {
return 0;
}
}
int main() {
int arr[] = {3, 1, 4, 1, 5, 9, 2, 6, 5, 3};
int n = sizeof(arr) / sizeof(int);
// 对数组进行排序
qsort(arr, n, sizeof(int), compare);
// 输出排序后的数组
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
```
在上面的示例中,compare 函数是一个比较函数,它接受两个指针参数,指向待比较的元素。在 compare 函数内部,我们将指针转换回原类型,然后比较两个元素的值。如果第一个元素小于第二个元素,则返回 -1;如果第一个元素大于第二个元素,则返回 1;如果两个元素相等,则返回 0。
相关推荐
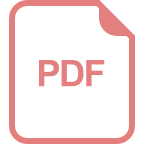
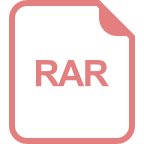












