解释这段代码:public static class AudioPlayer { public static void play(String filePath) { try { URL url = AudioPlayer.class.getClassLoader().getResource(filePath); AudioInputStream audioStream = AudioSystem.getAudioInputStream(url); Clip clip = AudioSystem.getClip(); clip.open(audioStream); clip.loop(Clip.LOOP_CONTINUOUSLY); } catch (Exception e) { e.printStackTrace(); } } }
时间: 2024-02-10 09:34:27 浏览: 69
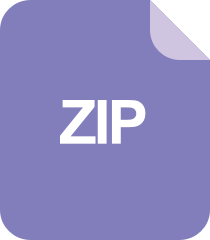
AudioPlayer.js.zip
这段代码定义了一个名为 `AudioPlayer` 的静态内部类,其中包含一个名为 `play` 的静态方法,用于播放音频文件。该方法接受一个字符串类型的参数 `filePath`,表示待播放的音频文件路径。
在方法中,首先通过 `AudioPlayer.class.getClassLoader().getResource(filePath)` 方法获取指定路径下的音频资源文件的 `URL` 对象,然后使用 `AudioSystem.getAudioInputStream(url)` 方法将 `URL` 对象转换为 `AudioInputStream` 对象。
接下来,使用 `AudioSystem.getClip()` 方法创建 `Clip` 对象,然后使用 `clip.open(audioStream)` 方法打开音频流并将其加载到 `Clip` 对象中。最后,使用 `clip.loop(Clip.LOOP_CONTINUOUSLY)` 方法设置循环播放模式,使音频文件可以一直循环播放。
如果在上述过程中发生异常,程序将打印异常堆栈信息。
阅读全文
相关推荐
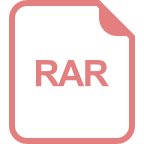
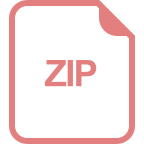















