Vue axios 写一个table 用到的组件越多越好
时间: 2024-02-18 09:02:56 浏览: 64
好,以下是一个使用 Vue 和 Axios 实现的 table 组件,包含了一些常用的组件和功能。
首先,需要安装 Vue 和 Axios。可以使用以下命令进行安装:
```
npm install vue axios
```
接下来,创建一个名为 `Table.vue` 的 Vue 组件文件,并添加以下代码:
```vue
<template>
<div>
<table>
<thead>
<tr>
<th v-for="(column, index) in columns" :key="index">{{ column.title }}</th>
</tr>
</thead>
<tbody>
<tr v-for="(row, rowIndex) in rows" :key="rowIndex">
<td v-for="(column, columnIndex) in columns" :key="columnIndex">
{{ row[column.key] }}
</td>
</tr>
</tbody>
</table>
<div v-if="loading">Loading...</div>
<div v-if="error">{{ error }}</div>
</div>
</template>
<script>
import axios from "axios";
export default {
name: "Table",
props: {
url: String,
columns: Array,
},
data() {
return {
rows: [],
loading: false,
error: null,
};
},
created() {
this.fetchData();
},
methods: {
async fetchData() {
this.loading = true;
try {
const response = await axios.get(this.url);
this.rows = response.data;
this.loading = false;
} catch (error) {
this.error = error.message;
this.loading = false;
}
},
},
};
</script>
```
以上代码定义了一个名为 `Table` 的 Vue 组件,并接收两个 props:`url` 和 `columns`。`url` 表示数据的来源,`columns` 表示表格的列信息。组件中使用了 Axios 库来从指定的 URL 获取数据,并将数据显示在表格中。当获取数据时,会显示一个 loading 提示;如果获取数据失败,会显示一个错误信息。
在以上示例中,使用了 Vue 的 v-for 指令来遍历数据,动态生成表格和表头。使用了 Axios 库来进行数据请求。使用了组件的 created 钩子函数来在组件创建时调用 fetchData 方法。fetchData 方法使用了 async/await 语法来处理异步请求,以及 try/catch 语法来处理错误。
你可以根据以上示例,进一步学习和使用 Vue 和 Axios,添加更多的组件和功能,实现更复杂的表格。
阅读全文
相关推荐
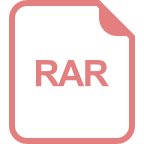
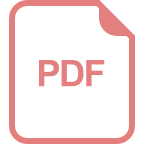
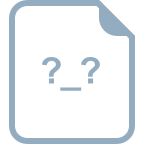
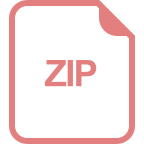
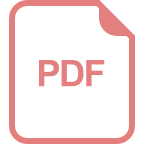
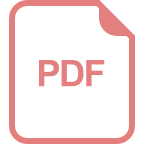
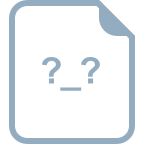
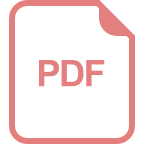
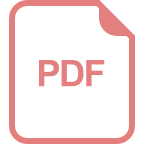
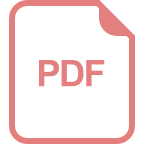
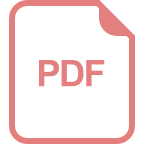
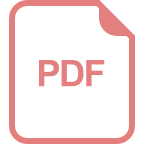
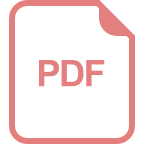
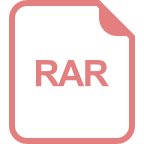
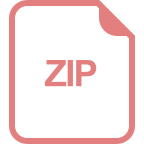