BeautifulSoup中previous_sibling
时间: 2024-04-05 22:09:02 浏览: 118
BeautifulSoup中的previous_sibling是指在HTML文档中,当前标签的上一个同级标签(即与当前标签具有相同父级标签的标签)。如果当前标签没有上一个同级标签,则返回None。
例如,假设我们有以下HTML代码:
```
<html>
<body>
<p>第一个段落</p>
<p>第二个段落</p>
<p>第三个段落</p>
</body>
</html>
```
我们可以使用BeautifulSoup来找到第二个段落标签(即<p>第二个段落</p>)的上一个同级标签,代码如下:
```python
from bs4 import BeautifulSoup
html = """
<html>
<body>
<p>第一个段落</p>
<p>第二个段落</p>
<p>第三个段落</p>
</body>
</html>
"""
soup = BeautifulSoup(html, 'html.parser')
second_p = soup.find_all('p')[1]
previous_p = second_p.previous_sibling
print(previous_p)
```
输出结果为:
```html
<p>第一个段落</p>
```
因为第二个段落标签的上一个同级标签是第一个段落标签。注意,上一个同级标签并不包括任何文本或空格。如果希望获取上一个同级标签的文本内容,可以使用previous_sibling.next_sibling.string。
相关问题
import os import re from bs4 import BeautifulSoup # 设置html文件路径 folder_path = r'C:\Users\test\Desktop\DIDItest' # 提取html文件内所需要数据 def extract_html_info(file_path): with open(file_path, 'r', encoding='utf-8') as file: # 读取HTML源代码 html = file.read() soup = BeautifulSoup(html, 'html.parser') # 提取所有的<p>标签 p_tags = soup.find_all('p') for p_tag in p_tags: # 提取ID talk_id = p_tag.find_previous(string=lambda text: isinstance(text, str) and '[talkid:' in text) talk_id = talk_id.strip('[talkid:]') # 提取时间 timestamp = p_tag.find_previous('body').find_previous('head').find('meta', {'http-equiv': 'Content=-Type'})[ 'content'] # 提取发送号码 send_number = p_tag.find('span', {'class': 'hint-success'}).text # 提取接收号码 receive_number = p_tag.find_all('span', {'class': 'hint-success'})[1].text # 提取信息内容 message = p_tag.previous_sibling.strip() # 遍历文件夹及子文件夹,提取HTML文件信息 for root, dirs, files in os.walk(folder_path): for file in files: if file.endswith('.html'): file_path = os.path.join(root, file) extract_html_info(file_path) # 判断是否是音频 if '音频' in message: file_url = p_tag.find('a')['href'] print( f"ID: {talk_id}, 时间: {timestamp}, 发送号码: {send_number}, 接收号码: {receive_number}, 音频文件地址: {file_url}") else: print( f"ID: {talk_id}, 时间: {timestamp}, 发送号码: {send_number}, 接收号码: {receive_number}, 信息内容: {message}")
你的代码有一些缩进错误,导致无法正确运行。以下是修正后的代码:
```python
import os
import re
from bs4 import BeautifulSoup
# 设置html文件路径
folder_path = r'C:\Users\test\Desktop\DIDItest'
# 提取html文件内所需要数据
def extract_html_info(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
# 读取HTML源代码
html = file.read()
soup = BeautifulSoup(html, 'html.parser')
# 提取所有的<p>标签
p_tags = soup.find_all('p')
for p_tag in p_tags:
# 提取ID
talk_id = p_tag.find_previous(string=lambda text: isinstance(text, str) and '[talkid:' in text)
talk_id = talk_id.strip('[talkid:]')
# 提取时间
timestamp = p_tag.find_previous('body').find_previous('head').find('meta', {'http-equiv': 'Content=-Type'})['content']
# 提取发送号码
send_number = p_tag.find('span', {'class': 'hint-success'}).text
# 提取接收号码
receive_number = p_tag.find_all('span', {'class': 'hint-success'})[1].text
# 提取信息内容
message = p_tag.previous_sibling.strip()
# 判断是否是音频
if '音频' in message:
file_url = p_tag.find('a')['href']
print(f"ID: {talk_id}, 时间: {timestamp}, 发送号码: {send_number}, 接收号码: {receive_number}, 音频文件地址: {file_url}")
else:
print(f"ID: {talk_id}, 时间: {timestamp}, 发送号码: {send_number}, 接收号码: {receive_number}, 信息内容: {message}")
# 遍历文件夹及子文件夹,提取HTML文件信息
for root, dirs, files in os.walk(folder_path):
for file in files:
if file.endswith('.html'):
file_path = os.path.join(root, file)
extract_html_info(file_path)
```
在这个修正后的代码中,我将提取HTML文件信息的部分封装到了一个函数`extract_html_info`中。然后,我使用`os.walk`函数遍历了指定文件夹及其子文件夹,找到所有的HTML文件,并调用`extract_html_info`函数来提取信息。
希望这次能够顺利运行!如果你还有其他问题,请随时提问。
beautifulsoup实例
### BeautifulSoup 使用示例
#### 解析 HTML 文件并提取数据
当使用 `BeautifulSoup` 处理网页内容时,通常会先读取HTML文件或字符串,并创建一个 `BeautifulSoup` 对象。此对象允许通过多种方法来导航和搜索文档中的标签。
```python
import bs4
from bs4 import BeautifulSoup
import requests
response = requests.get('http://example.com')
exampleSoup = bs4.BeautifulSoup(response.text, 'html.parser') # 创建BeautifulSoup对象[^1]
# 查找所有的<a>标签
elems = exampleSoup.select('a')
for elem in elems:
url = elem.get('href') # 获取链接地址
text = elem.getText() # 获取链接文字
print(f'URL: {url}, Text: {text}')
```
这段代码展示了如何获取页面上的所有超链接及其对应的显示文本[^2]。
#### 修改元素属性
除了简单的检索外,还可以轻松地更改现有标记内的特定属性:
```python
soup = BeautifulSoup("<p class='product'>Old Product Name</p>", "html.parser")
tag = soup.p
print(tag['class']) # 输出当前类名列表 ['product']
tag['class'] = 'new-product-name'
print(tag) # <p class="new-product-name">Old Product Name</p>
```
这里说明了怎样改变某个HTML元素的CSS类名称。
#### 遍历树形结构
对于更复杂的操作,比如访问父节点、兄弟节点或是子节点,可以借助于所提供的遍历函数来进行:
```python
html_doc = """
<html><head><title>The Dormouse's story</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
first_a_tag = soup.a
next_sibling = first_a_tag.find_next_sibling()
previous_sibling = next_sibling.find_previous_sibling()
print(first_a_tag.string) # Elsie
print(next_sibling.string) # Lacie
print(previous_sibling.string) # The Dormouse's story
```
上述实例演示了如何在一个HTML片段内定位相邻的手足关系以及前后顺序。
阅读全文
相关推荐



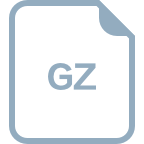
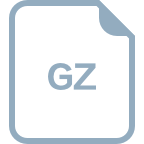
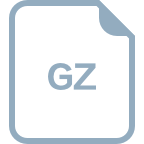










