detectron2mask labelme转coco
时间: 2023-05-25 14:04:10 浏览: 143
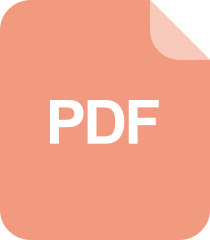
将labelme格式数据转化为标准的coco数据集格式方式

首先使用labelme将图像标注为json文件,然后将这些json文件转换为COCO格式,具体步骤如下:
1. 使用labelme标注并保存图片,得到对应的json标注文件。
2. 安装labelme2coco库,可以使用pip install labelme2coco进行安装。
3. 在Python代码中导入必要的库和函数:
```
import json
import os
import numpy as np
from labelme2coco import labelme2coco
```
4. 定义函数将所有的labelme json文件转换为COCO格式的json文件:
```
def labelme2cocoDir(labelme_dir, coco_path):
# labelme_dir:包含所有labelme json标注文件的目录
# coco_path:生成的COCO格式json文件路径
info = {
"description": "dataset_name",
"url": "https://github.com/me",
"version": "0.1.0",
"year": 2021,
"contributor": "me",
"date_created": "2021/01/01"
}
categories = []
category_index = {}
# 遍历labelme json文件,提取类别信息
for filename in os.listdir(labelme_dir):
if filename.endswith(".json"):
with open(os.path.join(labelme_dir, filename), "r") as f:
labelme_json = json.load(f)
for shape in labelme_json["shapes"]:
label = shape["label"]
if label not in category_index:
category_index[label] = len(categories)
categories.append({"id": category_index[label], "name": label})
# 将labelme json文件转换为COCO格式json文件
coco_dict = labelme2coco(labelme_dir)
coco_dict["info"] = info
coco_dict["categories"] = categories
# 写入COCO格式json文件
with open(coco_path, "w") as f:
json.dump(coco_dict, f)
```
5. 调用以上函数将所有的labelme json文件转换为COCO格式json文件,执行以下代码即可:
```
labelme_dir = "path/to/labelme/json/files"
coco_path = "path/to/coco/json/file"
labelme2cocoDir(labelme_dir, coco_path)
```
一些注意事项:
1. 在labelme中标注的类别名称需要保持一致,否则转换后的COCO格式json文件中会有重复的类别;
2. 如果只有单个labelme json文件,可以使用以下代码将其转换为COCO格式json文件:
```
labelme_json = "path/to/labelme/json/file"
coco_path = "path/to/coco/json/file"
labelme_dict = json.load(open(labelme_json))
coco_dict = labelme2coco(labelme_dict)
with open(coco_path, 'w') as f:
json.dump(coco_dict, f)
```
阅读全文
相关推荐
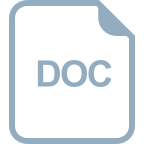
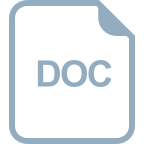
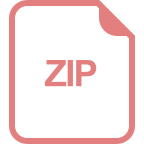
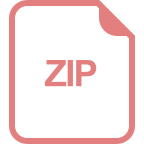
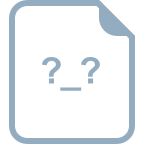
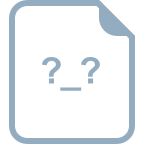


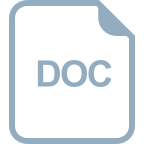
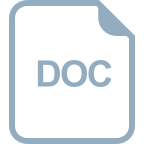
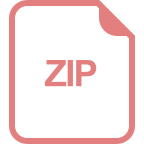