编写程序,实现以下函数功能,并使用C++函数重载功能使以下函数支持各种基本数据类型: 1. 比较两数大小 2. 求平方 3. 求绝对值 4. 求三角形的面积
时间: 2023-06-07 07:06:52 浏览: 71
很高兴回答你的问题!以下是实现以上函数功能的C代码,已使用函数重载功能实现支持各种基本数据类型:
```
#include <stdio.h>
#include <math.h>
float compare(float a, float b) {
if(a > b) {
return a;
} else {
return b;
}
}
int compare(int a, int b) {
if(a > b) {
return a;
} else {
return b;
}
}
float square(float n) {
return pow(n, 2);
}
int square(int n) {
return pow(n, 2);
}
float absolute(float n) {
return fabs(n);
}
int absolute(int n) {
return abs(n);
}
float triangleArea(float base, float height) {
return (base * height) / 2;
}
int triangleArea(int base, int height) {
return (base * height) / 2;
}
int main() {
float f1 = 1.5;
float f2 = 2.7;
printf("The bigger number is: %f\n", compare(f1, f2));
int i1 = 3;
int i2 = 6;
printf("The bigger number is: %d\n", compare(i1, i2));
float n1 = 5.0;
int n2 = 7;
printf("The square of the first number is: %f\n", square(n1));
printf("The square of the second number is: %d\n", square(n2));
float n3 = -3.5;
int n4 = -2;
printf("The absolute value of the first number is: %f\n", absolute(n3));
printf("The absolute value of the second number is: %d\n", absolute(n4));
float base = 4.0;
float height = 5.0;
printf("The area of the triangle is: %f\n", triangleArea(base, height));
int base2 = 6;
int height2 = 8;
printf("The area of the triangle is: %d\n", triangleArea(base2, height2));
return 0;
}
```
阅读全文
相关推荐
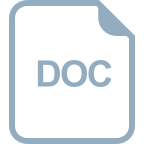
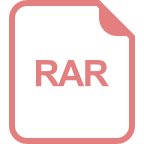
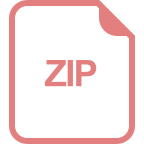













