用Java语言写一个消消乐小游戏
时间: 2023-12-07 20:54:34 浏览: 144
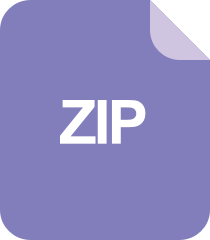
用Java写的一个小游戏
好的,这里提供一个简单的消消乐小游戏代码示例,仅供参考:
```java
import java.util.Random;
import java.util.Scanner;
public class Xiaoxiaole {
private static final int SIZE = 8; // 游戏区域大小
private static final int COLORS = 4; // 可选颜色数
private static final int MINIMUM = 3; // 最小消除数
private static final int SCORE_BASE = 10; // 每个方块基础得分
private int[][] board; // 游戏区域
private int score; // 当前得分
private boolean gameover; // 游戏是否结束
public Xiaoxiaole() {
board = new int[SIZE][SIZE];
score = 0;
gameover = false;
initBoard();
}
// 初始化游戏区域
private void initBoard() {
Random rand = new Random();
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
board[i][j] = rand.nextInt(COLORS) + 1;
}
}
}
// 打印当前游戏状态
public void printBoard() {
System.out.println("Score: " + score);
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
// 判断两个方块是否相邻
private boolean isAdjacent(int x1, int y1, int x2, int y2) {
return Math.abs(x1 - x2) + Math.abs(y1 - y2) == 1;
}
// 判断是否存在可消除的方块
private boolean hasMatch() {
boolean[][] marked = new boolean[SIZE][SIZE]; // 标记已经遍历过的方块
boolean found = false;
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
if (marked[i][j]) {
continue;
}
int color = board[i][j];
int count = 0; // 连续相同颜色的方块数
// 横向查找
for (int k = j; k < SIZE; k++) {
if (board[i][k] == color) {
count++;
} else {
break;
}
}
if (count >= MINIMUM) { // 找到一组可消除的方块
found = true;
for (int k = j; k < j + count; k++) {
marked[i][k] = true;
}
}
count = 0;
// 纵向查找
for (int k = i; k < SIZE; k++) {
if (board[k][j] == color) {
count++;
} else {
break;
}
}
if (count >= MINIMUM) {
found = true;
for (int k = i; k < i + count; k++) {
marked[k][j] = true;
}
}
}
}
return found;
}
// 消除所有被标记的方块
private int removeMatches() {
boolean[][] marked = new boolean[SIZE][SIZE]; // 标记已经遍历过的方块
int count = 0; // 被消除的方块数
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
if (marked[i][j]) {
continue;
}
int color = board[i][j];
int c = 0; // 连续相同颜色的方块数
// 横向查找
for (int k = j; k < SIZE; k++) {
if (board[i][k] == color) {
c++;
} else {
break;
}
}
if (c >= MINIMUM) { // 找到一组可消除的方块
count += c;
for (int k = j; k < j + c; k++) {
marked[i][k] = true;
}
}
c = 0;
// 纵向查找
for (int k = i; k < SIZE; k++) {
if (board[k][j] == color) {
c++;
} else {
break;
}
}
if (c >= MINIMUM) {
count += c;
for (int k = i; k < i + c; k++) {
marked[k][j] = true;
}
}
}
}
// 消除被标记的方块
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
if (marked[i][j]) {
board[i][j] = 0;
}
}
}
// 移动剩余方块
for (int j = 0; j < SIZE; j++) {
int k = SIZE - 1;
for (int i = SIZE - 1; i >= 0; i--) {
if (board[i][j] > 0) {
board[k--][j] = board[i][j];
}
}
while (k >= 0) {
board[k--][j] = 0;
}
}
// 更新得分
score += count * SCORE_BASE;
return count;
}
// 判断游戏是否结束
private boolean isGameOver() {
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
if (board[i][j] == 0) {
continue;
}
// 检查上下左右四个方向是否有相同颜色的方块
if (i > 0 && board[i-1][j] == board[i][j]) {
return false;
}
if (i < SIZE-1 && board[i+1][j] == board[i][j]) {
return false;
}
if (j > 0 && board[i][j-1] == board[i][j]) {
return false;
}
if (j < SIZE-1 && board[i][j+1] == board[i][j]) {
return false;
}
}
}
return true;
}
// 处理玩家输入
private void handleInput() {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter row and column (e.g. 1 2): ");
int row1 = scanner.nextInt();
int col1 = scanner.nextInt();
System.out.print("Enter row and column (e.g. 3 4): ");
int row2 = scanner.nextInt();
int col2 = scanner.nextInt();
if (!isAdjacent(row1, col1, row2, col2)) {
System.out.println("Error: These two blocks are not adjacent.");
return;
}
int temp = board[row1-1][col1-1];
board[row1-1][col1-1] = board[row2-1][col2-1];
board[row2-1][col2-1] = temp;
if (!hasMatch()) { // 没有可消除的方块,恢复交换的方块
temp = board[row1-1][col1-1];
board[row1-1][col1-1] = board[row2-1][col2-1];
board[row2-1][col2-1] = temp;
System.out.println("Error: There is no match after swapping these two blocks.");
}
}
// 游戏主循环
public void run() {
while (!gameover) {
printBoard();
if (!hasMatch()) { // 没有可消除的方块,游戏结束
gameover = true;
System.out.println("Game over!");
break;
}
int count = removeMatches();
if (count > 0) { // 有方块被消除,继续游戏
System.out.println("You got " + count + " points.");
} else { // 没有方块被消除,等待玩家输入
handleInput();
}
}
}
public static void main(String[] args) {
Xiaoxiaole game = new Xiaoxiaole();
game.run();
}
}
```
这是一个控制台程序,运行后会生成一个 8x8 的消消乐游戏区域,玩家可以交换相邻的两个方块,如果有三个或以上颜色相同的方块连成一条线,则会被消除,得到基础得分。游戏结束的条件是没有可消除的方块或者玩家主动退出游戏。
阅读全文
相关推荐

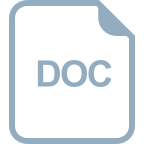
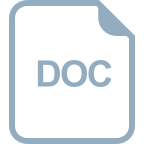
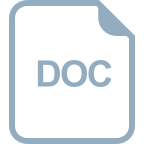
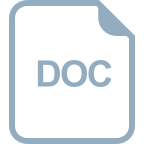

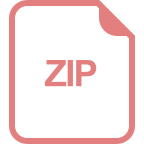
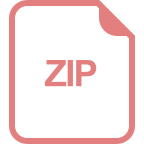
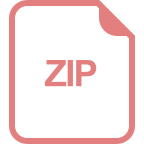
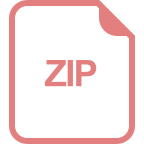
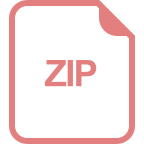


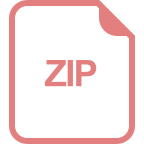
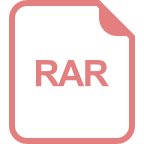