python去掉字符串里的()
时间: 2023-08-19 14:05:44 浏览: 45
可以使用Python的字符串替换功能,将字符串中的括号替换成空字符串。例如:
```python
s = "(Hello) World!"
s = s.replace("(", "").replace(")", "")
print(s) # 输出 "Hello World!"
```
这里使用了 `replace()` 方法两次,第一次将左括号替换成空字符串,第二次将右括号替换成空字符串,从而去掉了字符串中的括号。
相关问题
python 去除字符串末尾
要去除Python字符串末尾的空格,可以使用rstrip()方法。该方法会返回一个去除了字符串末尾空格的新字符串。例如,如果有一个字符串name = "Sheep Core ",可以使用name.rstrip()来去除末尾的空格,结果为'Sheep Core'。\[1\]
#### 引用[.reference_title]
- *1* *2* [python 去掉字符串首尾空格](https://blog.csdn.net/u014651560/article/details/128410404)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
python 去掉字符串
要去掉字符串两端的空格,可以使用字符串的 `strip` 方法。例如:
```python
s = " hello world "
s = s.strip()
print(s) # 输出:hello world
```
如果要只去掉左边或右边的空格,可以使用 `lstrip` 或 `rstrip` 方法。例如:
```python
s = " hello world "
s = s.lstrip()
print(s) # 输出:hello world
s = " hello world "
s = s.rstrip()
print(s) # 输出: hello world
```
如果要去掉字符串中间的空格,可以使用字符串的 `replace` 方法。例如:
```python
s = "hello world"
s = s.replace(" ", "")
print(s) # 输出:helloworld
```
如果要去掉字符串中指定的字符,可以使用字符串的 `replace` 方法或 `translate` 方法。例如:
```python
s = "hello, world!"
s = s.replace(",", "").replace("!", "")
print(s) # 输出:hello world
s = "hello, world!"
translator = str.maketrans("", "", ",!")
s = s.translate(translator)
print(s) # 输出:hello world
```
相关推荐
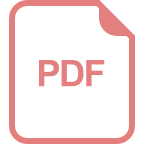
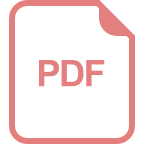
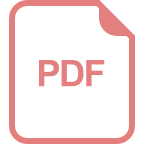
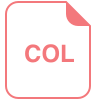
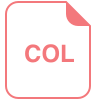
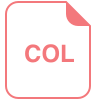
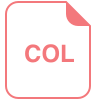
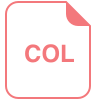







