用tensorflow写一个逻辑回归的代码
时间: 2024-05-09 12:18:59 浏览: 7
以下是使用 TensorFlow 实现逻辑回归的示例代码:
```
import tensorflow as tf
import numpy as np
# 定义训练数据
x_train = np.array([[1.0, 2.0], [2.0, 3.0], [3.0, 4.0], [4.0, 5.0], [5.0, 6.0]])
y_train = np.array([[0.0], [0.0], [1.0], [1.0], [1.0]])
# 定义模型参数
W = tf.Variable(tf.zeros([2, 1]))
b = tf.Variable(tf.zeros([1]))
# 定义模型
def logistic_regression(x):
return tf.sigmoid(tf.matmul(x, W) + b)
# 定义损失函数
def loss(x, y):
y_pred = logistic_regression(x)
return tf.reduce_mean(tf.nn.sigmoid_cross_entropy_with_logits(labels=y, logits=y_pred))
# 定义优化器
optimizer = tf.train.GradientDescentOptimizer(learning_rate=0.01)
# 定义训练步骤
def train_step(x, y):
with tf.GradientTape() as tape:
cost = loss(x, y)
gradients = tape.gradient(cost, [W, b])
optimizer.apply_gradients(zip(gradients, [W, b]))
# 训练模型
for epoch in range(1000):
train_step(x_train, y_train)
# 预测结果
x_test = np.array([[2.0, 1.0], [3.0, 2.0], [4.0, 3.0]])
y_test = np.array([[0.0], [0.0], [1.0]])
y_pred = logistic_regression(x_test)
correct_prediction = tf.equal(tf.round(y_pred), y_test)
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
print("Accuracy:", accuracy.numpy())
```
相关推荐
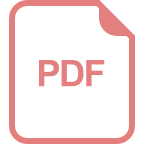
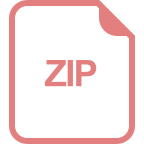
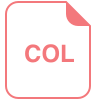
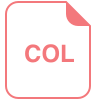
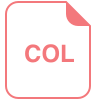
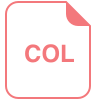
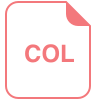









