#include "myenroll.h" myenroll::myenroll(QWidget *parent) : QWidget(parent) { init_ui(); connect(this->bnt1,&QPushButton::clicked,this,&myenroll::send_msg); } void myenroll::init_ui() { this->setFixedSize(QSize(600,350)); this->setWindowTitle(tr("注册帐号")); this->setWindowIcon(QIcon(":/src/1.png")); name = new QLineEdit; //用于写入名字 pass = new QLineEdit; //用于写入密码 lb1 = new QLabel; lb2 = new QLabel; bnt1 = new QPushButton; hb1 = new QHBoxLayout; hb2 = new QHBoxLayout; hb3 = new QHBoxLayout; vb1 = new QVBoxLayout; this->lb1->setText(tr("请输入账号:")); this->lb2->setText(tr("请输入密码:")); this->bnt1->setText(tr("确认")); this->hb1->addWidget(lb1); this->hb1->addWidget(name); this->hb2->addWidget(lb2); this->hb2->addWidget(pass); this->hb3->addWidget(bnt1); this->vb1->addLayout(hb1); this->vb1->addLayout(hb2); this->vb1->addLayout(hb3); this->setLayout(vb1); } void myenroll::send_msg() { emit sig_enroll_info(name->text(),pass->text()); }解释并注释每行代码的语法并说明各
时间: 2024-03-18 13:41:52 浏览: 18
行代码的作用和功能。
```
#include "myenroll.h" // 引入 myenroll 类的头文件
myenroll::myenroll(QWidget *parent) : QWidget(parent) { // myenroll 构造函数的实现,需要传入一个 QWidget 类型的父窗口对象指针作为参数,使用初始化列表对成员变量初始化
init_ui(); // 初始化 UI 界面
connect(this->bnt1,&QPushButton::clicked,this,&myenroll::send_msg); // 将按钮 bnt1 的 clicked 信号连接到 send_msg() 槽函数上
}
void myenroll::init_ui() { // 初始化 UI 界面的函数
this->setFixedSize(QSize(600,350)); // 设置窗口大小为 600x350
this->setWindowTitle(tr("注册帐号")); // 设置窗口标题为 “注册帐号”
this->setWindowIcon(QIcon(":/src/1.png")); // 设置窗口图标
name = new QLineEdit; // 创建 QLineEdit 对象 name,用于输入用户名
pass = new QLineEdit; // 创建 QLineEdit 对象 pass,用于输入密码
lb1 = new QLabel; // 创建 QLabel 对象 lb1,用于显示 “请输入账号:” 文本
lb2 = new QLabel; // 创建 QLabel 对象 lb2,用于显示 “请输入密码:” 文本
bnt1 = new QPushButton; // 创建 QPushButton 对象 bnt1,用于触发注册操作
hb1 = new QHBoxLayout; // 创建 QHBoxLayout 对象 hb1,用于布局 label1 和 name
hb2 = new QHBoxLayout; // 创建 QHBoxLayout 对象 hb2,用于布局 label2 和 pass
hb3 = new QHBoxLayout; // 创建 QHBoxLayout 对象 hb3,用于布局 bnt1
vb1 = new QVBoxLayout; // 创建 QVBoxLayout 对象 vb1,用于布局 hb1、hb2 和 hb3
this->lb1->setText(tr("请输入账号:")); // 设置 lb1 的文本为 “请输入账号:”
this->lb2->setText(tr("请输入密码:")); // 设置 lb2 的文本为 “请输入密码:”
this->bnt1->setText(tr("确认")); // 设置 bnt1 的文本为 “确认”
this->hb1->addWidget(lb1); // 将 lb1 添加到 hb1 布局中
this->hb1->addWidget(name); // 将 name 添加到 hb1 布局中
this->hb2->addWidget(lb2); // 将 lb2 添加到 hb2 布局中
this->hb2->addWidget(pass); // 将 pass 添加到 hb2 布局中
this->hb3->addWidget(bnt1); // 将 bnt1 添加到 hb3 布局中
this->vb1->addLayout(hb1); // 将 hb1 添加到 vb1 布局中
this->vb1->addLayout(hb2); // 将 hb2 添加到 vb1 布局中
this->vb1->addLayout(hb3); // 将 hb3 添加到 vb1 布局中
this->setLayout(vb1); // 设置当前窗口的布局为 vb1
}
void myenroll::send_msg() { // 发送注册信息的函数
emit sig_enroll_info(name->text(),pass->text()); // 发送 sig_enroll_info 信号,将当前输入的用户名和密码作为参数传递出去
}
```
该代码实现了一个简单的注册界面,包括输入用户名和密码以及注册按钮。通过点击注册按钮,可以将输入的用户名和密码发送出去,用于注册账号。其中,使用了 Qt 框架提供的 QWidget、QLineEdit、QLabel、QPushButton、QHBoxLayout 和 QVBoxLayout 等类来实现界面布局和功能实现。同时,也使用了 Qt 框架提供的信号与槽机制来实现不同组件之间的交互。
相关推荐
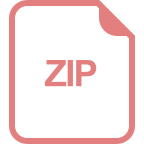




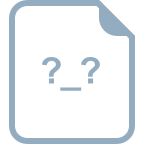
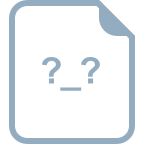