php编写一个学生类_PHP实现简单的学生信息管理系统(web版)
时间: 2024-01-27 19:05:01 浏览: 64
好的,我可以为您提供一个基本的学生信息管理系统示例,使用 PHP 和 MySQL 来存储和管理数据。以下是基本的代码示例:
首先,我们需要创建一个数据库,并在其中创建一个名为“students”的表。该表应包含以下字段:id(INT,主键),name(VARCHAR),age(INT),gender(VARCHAR)和address(VARCHAR)。
接下来,我们需要创建一个名为“index.php”的文件,该文件将显示所有学生的列表,并包含以下代码:
```php
<!DOCTYPE html>
<html>
<head>
<title>学生信息管理系统</title>
</head>
<body>
<h1>学生信息管理系统</h1>
<a href="add.php">添加学生</a>
<table>
<tr>
<th>ID</th>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
<th>地址</th>
<th>操作</th>
</tr>
<?php
// 连接到数据库
$conn = mysqli_connect("localhost", "root", "", "students");
// 检查连接是否成功
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
// 查询所有学生
$sql = "SELECT * FROM students";
$result = mysqli_query($conn, $sql);
// 输出每个学生的信息
if (mysqli_num_rows($result) > 0) {
while($row = mysqli_fetch_assoc($result)) {
echo "<tr>";
echo "<td>" . $row["id"] . "</td>";
echo "<td>" . $row["name"] . "</td>";
echo "<td>" . $row["age"] . "</td>";
echo "<td>" . $row["gender"] . "</td>";
echo "<td>" . $row["address"] . "</td>";
echo "<td><a href='edit.php?id=" . $row["id"] . "'>编辑</a> <a href='delete.php?id=" . $row["id"] . "'>删除</a></td>";
echo "</tr>";
}
} else {
echo "0 个结果";
}
// 关闭数据库连接
mysqli_close($conn);
?>
</table>
</body>
</html>
```
接下来,我们需要创建一个名为“add.php”的文件,该文件将允许用户添加新的学生,包含以下代码:
```php
<!DOCTYPE html>
<html>
<head>
<title>添加学生</title>
</head>
<body>
<h1>添加学生</h1>
<form method="post" action="save.php">
<label for="name">姓名:</label>
<input type="text" id="name" name="name"><br><br>
<label for="age">年龄:</label>
<input type="text" id="age" name="age"><br><br>
<label for="gender">性别:</label>
<input type="text" id="gender" name="gender"><br><br>
<label for="address">地址:</label>
<input type="text" id="address" name="address"><br><br>
<input type="submit" value="提交">
</form>
</body>
</html>
```
然后,我们需要创建一个名为“save.php”的文件,该文件将处理表单提交并将新学生添加到数据库中,包含以下代码:
```php
<?php
// 连接到数据库
$conn = mysqli_connect("localhost", "root", "", "students");
// 检查连接是否成功
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
// 获取用户提交的数据
$name = $_POST["name"];
$age = $_POST["age"];
$gender = $_POST["gender"];
$address = $_POST["address"];
// 将新学生添加到数据库中
$sql = "INSERT INTO students (name, age, gender, address) VALUES ('$name', '$age', '$gender', '$address')";
if (mysqli_query($conn, $sql)) {
echo "新学生添加成功";
} else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
// 关闭数据库连接
mysqli_close($conn);
?>
```
最后,我们需要创建一个名为“edit.php”的文件,该文件将允许用户编辑现有的学生信息,包含以下代码:
```php
<!DOCTYPE html>
<html>
<head>
<title>编辑学生</title>
</head>
<body>
<h1>编辑学生</h1>
<?php
// 连接到数据库
$conn = mysqli_connect("localhost", "root", "", "students");
// 检查连接是否成功
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
// 获取要编辑的学生ID
$id = $_GET["id"];
// 查询要编辑的学生信息
$sql = "SELECT * FROM students WHERE id='$id'";
$result = mysqli_query($conn, $sql);
// 输出要编辑的学生信息
if (mysqli_num_rows($result) > 0) {
$row = mysqli_fetch_assoc($result);
echo "<form method='post' action='update.php'>";
echo "<input type='hidden' name='id' value='" . $row["id"] . "'>";
echo "<label for='name'>姓名:</label>";
echo "<input type='text' id='name' name='name' value='" . $row["name"] . "'><br><br>";
echo "<label for='age'>年龄:</label>";
echo "<input type='text' id='age' name='age' value='" . $row["age"] . "'><br><br>";
echo "<label for='gender'>性别:</label>";
echo "<input type='text' id='gender' name='gender' value='" . $row["gender"] . "'><br><br>";
echo "<label for='address'>地址:</label>";
echo "<input type='text' id='address' name='address' value='" . $row["address"] . "'><br><br>";
echo "<input type='submit' value='提交'>";
echo "</form>";
} else {
echo "未找到学生信息";
}
// 关闭数据库连接
mysqli_close($conn);
?>
</body>
</html>
```
最后,我们需要创建一个名为“update.php”的文件,该文件将处理表单提交并更新学生信息,包含以下代码:
```php
<?php
// 连接到数据库
$conn = mysqli_connect("localhost", "root", "", "students");
// 检查连接是否成功
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
// 获取用户提交的数据
$id = $_POST["id"];
$name = $_POST["name"];
$age = $_POST["age"];
$gender = $_POST["gender"];
$address = $_POST["address"];
// 更新学生信息
$sql = "UPDATE students SET name='$name', age='$age', gender='$gender', address='$address' WHERE id='$id'";
if (mysqli_query($conn, $sql)) {
echo "学生信息更新成功";
} else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
// 关闭数据库连接
mysqli_close($conn);
?>
```
以上就是一个简单的学生信息管理系统的示例。当然,还有很多改进和优化的空间,例如添加输入验证、使用面向对象编程等。
阅读全文
相关推荐
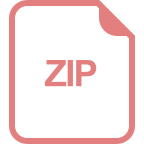
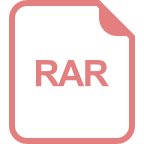
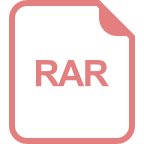
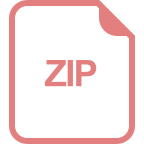
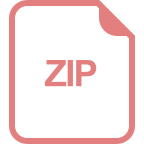
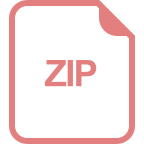
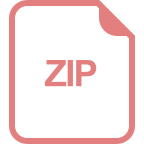
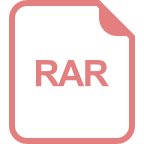
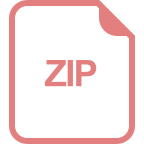
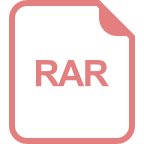
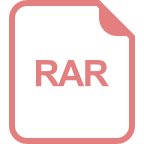
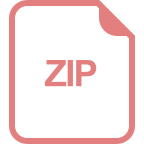
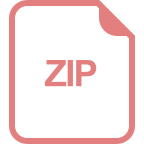
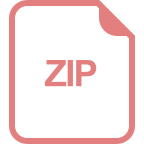
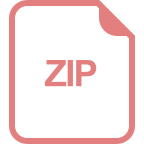
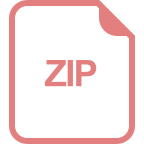
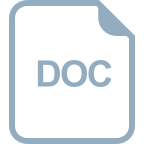
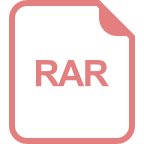