android开发一个记事本代码
时间: 2024-02-16 12:00:25 浏览: 129
以下是一个简单的 Android 记事本应用的代码,包含保存、读取、清空、撤销、重做、搜索和分享功能:
MainActivity.java
```java
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.text.Editable;
import android.text.TextWatcher;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.EditText;
import android.widget.Toast;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.util.Stack;
public class MainActivity extends AppCompatActivity {
private EditText editText;
private Stack<String> undoStack = new Stack<>();
private Stack<String> redoStack = new Stack<>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.editText);
// 添加输入框的监听器,当输入框中的内容发生变化时自动保存
editText.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
// 将当前内容保存到撤销栈中
undoStack.push(s.toString());
}
@Override
public void afterTextChanged(Editable s) {
}
});
// 读取保存的内容
String text = readText();
editText.setText(text);
editText.setSelection(text.length());
}
// 创建菜单
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
// 处理菜单点击事件
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId();
switch (id) {
case R.id.action_save:
// 保存输入框中的内容
saveText();
return true;
case R.id.action_load:
// 读取保存的内容
String text = readText();
editText.setText(text);
editText.setSelection(text.length());
return true;
case R.id.action_clear:
// 清空输入框中的内容
editText.setText("");
return true;
case R.id.action_undo:
// 撤销操作
if (!undoStack.isEmpty()) {
String text1 = undoStack.pop();
redoStack.push(text1);
if (!undoStack.isEmpty()) {
String text2 = undoStack.peek();
editText.setText(text2);
editText.setSelection(text2.length());
} else {
editText.setText("");
}
}
return true;
case R.id.action_redo:
// 重做操作
if (!redoStack.isEmpty()) {
String text1 = redoStack.pop();
undoStack.push(text1);
editText.setText(text1);
editText.setSelection(text1.length());
}
return true;
case R.id.action_search:
// 搜索输入框中的内容
Intent intent = new Intent(Intent.ACTION_SEND);
intent.setType("text/plain");
intent.putExtra(Intent.EXTRA_TEXT, editText.getText().toString());
startActivity(Intent.createChooser(intent, "分享到"));
return true;
default:
return super.onOptionsItemSelected(item);
}
}
// 保存输入框中的内容到文件中
private void saveText() {
try {
File file = new File(getFilesDir(), "note.txt");
FileOutputStream fos = new FileOutputStream(file);
OutputStreamWriter osw = new OutputStreamWriter(fos);
osw.write(editText.getText().toString());
osw.close();
Toast.makeText(MainActivity.this, "保存成功", Toast.LENGTH_SHORT).show();
} catch (Exception e) {
e.printStackTrace();
Toast.makeText(MainActivity.this, "保存失败", Toast.LENGTH_SHORT).show();
}
}
// 从文件中读取保存的内容
private String readText() {
StringBuilder sb = new StringBuilder();
try {
File file = new File(getFilesDir(), "note.txt");
FileInputStream fis = new FileInputStream(file);
InputStreamReader isr = new InputStreamReader(fis);
BufferedReader br = new BufferedReader(isr);
String line;
while ((line = br.readLine()) != null) {
sb.append(line).append('\n');
}
br.close();
} catch (Exception e) {
e.printStackTrace();
}
return sb.toString();
}
}
```
activity_main.xml
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scrollbars="vertical" />
</RelativeLayout>
```
menu_main.xml
```xml
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<item
android:id="@+id/action_save"
android:title="保存"
app:showAsAction="always" />
<item
android:id="@+id/action_load"
android:title="读取"
app:showAsAction="always" />
<item
android:id="@+id/action_clear"
android:title="清空"
app:showAsAction="always" />
<item
android:id="@+id/action_undo"
android:title="撤销"
app:showAsAction="ifRoom" />
<item
android:id="@+id/action_redo"
android:title="重做"
app:showAsAction="ifRoom" />
<item
android:id="@+id/action_search"
android:title="分享"
app:showAsAction="never" />
</menu>
```
注意:上述代码仅供参考,实际应用需要根据具体需求进行修改和扩展。
阅读全文
相关推荐
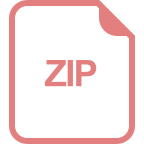

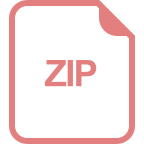
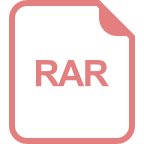
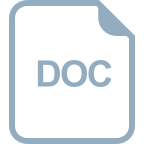
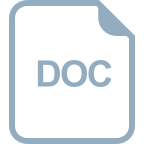
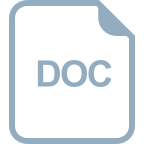









