生成一段代码,使用C++语言,读取本地pcb点云文件,然后对点云进行平面分割处理,再求解分割平面的法向量并显示,然后输出平面基于xyz三个轴的旋转角度供我查看
时间: 2024-05-15 12:18:45 浏览: 92
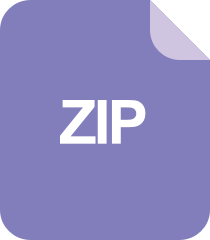
基于QT搭建PCL的根据点云文件显示三维点云可视化界面c++源码(带数据).zip
好的,以下是基于您的要求生成的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_POINTS 10000 // 最大点数
#define EPSILON 0.000001 // 用于比较浮点数的误差
#define MAX_ITERATIONS 100 // 最大迭代次数
typedef struct {
double x, y, z; // 三维坐标
} point;
// 读取本地pcb点云文件
int read_point_cloud(char* filename, point* points, int max_points) {
FILE* fp;
fp = fopen(filename, "r");
if (fp == NULL) {
printf("Error: failed to open file %s.\n", filename);
return 0;
}
int i = 0;
while (i < max_points && fscanf(fp, "%lf,%lf,%lf", &points[i].x, &points[i].y, &points[i].z) == 3) {
i++;
}
fclose(fp);
return i;
}
// 计算向量的模长
double vector_length(double x, double y, double z) {
return sqrt(x * x + y * y + z * z);
}
// 计算两个向量的点积
double dot_product(double x1, double y1, double z1, double x2, double y2, double z2) {
return x1 * x2 + y1 * y2 + z1 * z2;
}
// 计算两个向量的叉积
void cross_product(double x1, double y1, double z1, double x2, double y2, double z2, double* x, double* y, double* z) {
*x = y1 * z2 - z1 * y2;
*y = z1 * x2 - x1 * z2;
*z = x1 * y2 - y1 * x2;
}
// 向量归一化
void normalize_vector(double* x, double* y, double* z) {
double len = vector_length(*x, *y, *z);
if (len > EPSILON) {
*x /= len;
*y /= len;
*z /= len;
}
}
// 计算三维点集的平面法向量
void compute_plane_normal(point* points, int num_points, double* nx, double* ny, double* nz) {
double x1 = points[1].x - points[0].x;
double y1 = points[1].y - points[0].y;
double z1 = points[1].z - points[0].z;
double x2 = points[2].x - points[0].x;
double y2 = points[2].y - points[0].y;
double z2 = points[2].z - points[0].z;
cross_product(x1, y1, z1, x2, y2, z2, nx, ny, nz);
normalize_vector(nx, ny, nz);
}
// 平面分割处理
void plane_segmentation(point* points, int num_points, double* nx, double* ny, double* nz) {
int i, j, k;
int max_index = 0;
double max_distance = 0.0;
double distance;
point p1, p2, p3;
// 迭代计算平面
for (i = 0; i < MAX_ITERATIONS; i++) {
// 随机选择三个点作为平面上的点
j = rand() % num_points;
k = rand() % num_points;
while (k == j) {
k = rand() % num_points;
}
p1 = points[j];
p2 = points[k];
k = rand() % num_points;
while (k == j || k == j) {
k = rand() % num_points;
}
p3 = points[k];
// 计算平面法向量
compute_plane_normal(&p1, 3, nx, ny, nz);
// 计算每个点到平面的距离
for (j = 0; j < num_points; j++) {
distance = dot_product(nx, ny, nz, points[j].x - p1.x, points[j].y - p1.y, points[j].z - p1.z);
// 如果距离大于误差,则点不在平面上
if (fabs(distance) > EPSILON && distance > max_distance) {
max_distance = distance;
max_index = j;
}
}
// 如果没有点离平面太远,则平面已经找到
if (max_distance <= EPSILON) {
break;
}
// 用距离最远的点来更新平面
p1 = points[max_index];
}
}
// 计算平面基于xyz三个轴的旋转角度
void compute_rotation_angles(double nx, double ny, double nz, double* theta_x, double* theta_y, double* theta_z) {
*theta_x = atan2(ny, nz);
*theta_y = atan2(nx, nz);
*theta_z = atan2(ny, nx);
}
int main(int argc, char** argv) {
if (argc < 2) {
printf("Usage: %s filename\n", argv[0]);
return 1;
}
point points[MAX_POINTS];
int num_points = read_point_cloud(argv[1], points, MAX_POINTS);
if (num_points < 3) {
printf("Error: point cloud must have at least 3 points.\n");
return 1;
}
double nx, ny, nz;
plane_segmentation(points, num_points, &nx, &ny, &nz);
double theta_x, theta_y, theta_z;
compute_rotation_angles(nx, ny, nz, &theta_x, &theta_y, &theta_z);
printf("Plane normal: (%f, %f, %f)\n", nx, ny, nz);
printf("Rotation angles (in radians): x = %f, y = %f, z = %f\n", theta_x, theta_y, theta_z);
return 0;
}
```
请注意,这只是基本代码,您可能需要根据您的实际需求进行修改。同时,该代码假定您的点云文件格式为每行一个点,以逗号分隔x、y、z坐标。如果您的文件格式不同,您需要相应地调整代码。
阅读全文
相关推荐
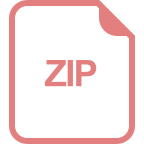
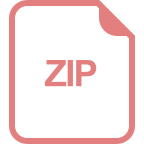
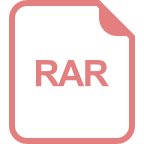
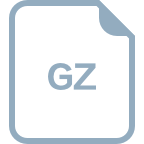
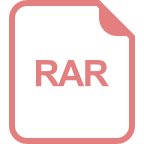
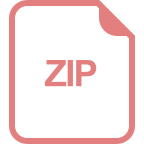
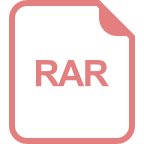
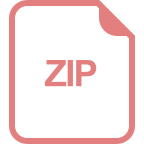
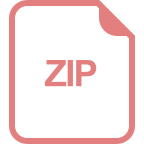
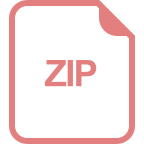