C++实现商用RSA密码
时间: 2023-10-20 10:24:20 浏览: 99
实现商用RSA密码需要注意以下几点:
1. 生成密钥对:RSA加密算法需要公钥和私钥两个密钥,可以使用C++的大数库生成大素数、计算欧拉函数、选择公钥和私钥等操作。
2. 加密数据:使用公钥对明文进行加密,可以使用C++的大数库进行快速幂运算。
3. 解密数据:使用私钥对密文进行解密,同样可以使用C++的大数库进行快速幂运算。
4. 数据转换:在加密和解密过程中,需要将字符串或文件内容转换为大整数,或将大整数转换为字符串或文件内容。
以下是一个简单的C++实现商用RSA密码的示例代码:
```cpp
#include <iostream>
#include <string>
#include "RSA.h" // 自己实现的RSA类
using namespace std;
int main() {
// 生成密钥对
RSA rsa;
rsa.generateKeyPair(1024); // 生成1024位的密钥对
// 显示公钥和私钥
cout << "Public key: " << rsa.getPublicKey() << endl;
cout << "Private key: " << rsa.getPrivateKey() << endl;
// 加密数据
string plaintext = "Hello, world!";
RSA::BigInteger ciphertext = rsa.encrypt(plaintext);
// 显示密文
cout << "Ciphertext: " << ciphertext.toString() << endl;
// 解密数据
string decryptedtext = rsa.decrypt(ciphertext);
// 显示明文
cout << "Decrypted text: " << decryptedtext << endl;
return 0;
}
```
注意:RSA加密算法是一种公钥密码体制,因此公钥可以公开,但私钥必须保密。在实际应用中,需要使用加密模式、填充方式等技术来增强RSA算法的安全性。
阅读全文
相关推荐
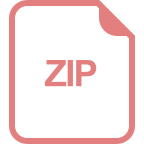
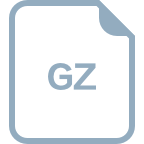
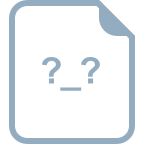
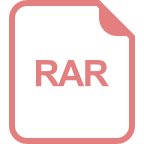
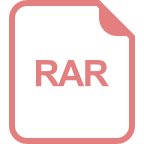
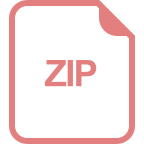
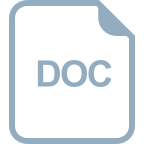
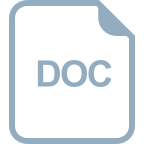
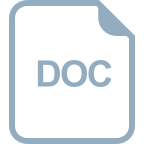
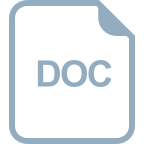
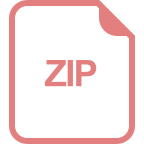
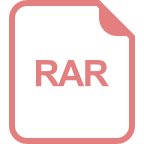
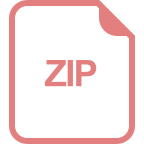