用c语言解决5乘5迷宫问题 的最短路径1为可通行的地方0为障碍
时间: 2023-11-27 07:49:02 浏览: 81
要解决这个问题,我们可以使用广度优先搜索算法(BFS)来找到从起点到终点的最短路径。下面是一个简单的C语言程序,它使用BFS算法来解决迷宫问题:
```c
#include <stdio.h>
#include <stdlib.h>
#define ROWS 5
#define COLS 5
int maze[ROWS][COLS] = { {1, 0, 1, 1, 1},
{1, 0, 1, 0, 1},
{1, 0, 1, 0, 1},
{1, 0, 1, 0, 1},
{1, 1, 1, 0, 1} };
typedef struct {
int x;
int y;
int distance;
} Node;
Node queue[ROWS*COLS];
int front = 0, rear = 0;
void enqueue(Node n) {
queue[rear++] = n;
}
Node dequeue() {
return queue[front++];
}
int is_empty() {
return front == rear;
}
int is_valid(int x, int y) {
if (x < 0 || y < 0 || x >= ROWS || y >= COLS) {
return 0;
}
if (maze[x][y] == 0) {
return 0;
}
return 1;
}
int bfs(int sx, int sy, int tx, int ty) {
Node start = {sx, sy, 0};
enqueue(start);
maze[sx][sy] = 0;
while (!is_empty()) {
Node current = dequeue();
if (current.x == tx && current.y == ty) {
return current.distance;
}
Node next = {current.x + 1, current.y, current.distance + 1};
if (is_valid(next.x, next.y)) {
enqueue(next);
maze[next.x][next.y] = 0;
}
next.x = current.x - 1;
next.y = current.y;
if (is_valid(next.x, next.y)) {
enqueue(next);
maze[next.x][next.y] = 0;
}
next.x = current.x;
next.y = current.y + 1;
if (is_valid(next.x, next.y)) {
enqueue(next);
maze[next.x][next.y] = 0;
}
next.x = current.x;
next.y = current.y - 1;
if (is_valid(next.x, next.y)) {
enqueue(next);
maze[next.x][next.y] = 0;
}
}
return -1; // no path found
}
int main() {
int sx = 0, sy = 0;
int tx = 4, ty = 4;
int distance = bfs(sx, sy, tx, ty);
if (distance != -1) {
printf("Shortest path from (%d,%d) to (%d,%d) is %d\n", sx, sy, tx, ty, distance);
} else {
printf("No path found from (%d,%d) to (%d,%d)\n", sx, sy, tx, ty);
}
return 0;
}
```
这个程序首先定义了一个5x5的迷宫(用0表示障碍,1表示可通行的地方),然后使用BFS算法来找到从起点(0,0)到终点(4,4)的最短路径。在BFS算法中,我们将起点添加到队列中,并且将起点的值设置为0,表示已经访问过。然后我们循环处理队列中的节点,直到队列为空或者找到了终点。
在每个节点中,我们生成四个相邻节点,并将它们添加到队列中。如果一个节点是有效的(即不越界且不是障碍),我们将它的值设置为0,表示已经访问过。
最后,如果我们成功找到了终点,我们返回从起点到终点的距离。如果没有找到路径,我们返回-1。
阅读全文
相关推荐


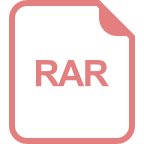



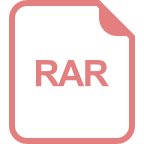







