private static void addStudent() { Student student = new Student(); System.out.print("请输入姓名:"); student.name = input.next(); System.out.print("请输入学号:"); int id = input.nextInt(); boolean noCheck = true; while (noCheck) { if (studentNoList.contains(id)) {//已存在的学号 System.out.print("学号已存在,请重新输入:"); id = input.nextInt(); } else { student.id = id; studentNoList.add(id); noCheck = false; } } System.out.print("请输入年龄:"); student.age = input.nextInt(); student.setScoreList(new ArrayList<>()); studentList.add(student); System.out.println("---------------------添加学生成功---------------------"); menu(); }这段代码是什么意思
时间: 2023-02-15 14:50:09 浏览: 81
这段代码是 Java 语言中的一段程序,它定义了一个名为 "addStudent" 的方法,用于在程序中添加学生信息。
具体来说,它会提示用户输入学生的姓名、学号、年龄,然后创建一个 Student 类的实例,将用户输入的信息存储到该实例中。然后,程序会检查输入的学号是否已经存在,如果已经存在,则提示用户重新输入;如果不存在,则将学号添加到 studentNoList 列表中,并将 Student 实例添加到 studentList 列表中。最后,程序会显示 "添加学生成功" 的提示信息,并调用 menu() 方法,继续执行程序的其他部分。
相关问题
public static void searchStudentByCollege(Map<String,College>colleges) { Scanner scanner= new Scanner(System.in); System.out.print("请输入要查询的学院名称:"); String collegeName = scanner.next(); if (colleges.containsKey(collegeName)) { College college = colleges.get(collegeName); List<ClassInfo> classInCollege = college.getAllClasses(); System.out.println("------ 学生信息 ------"); for (ClassInfo classInfo : classInCollege) { System.out.println(classInfo); List<Student> studentsInCollege = college.getStudentsByCollege(); for (Student student : studentsInCollege) { System.out.println(student); } } System.out.println("----------------------"); } else { System.out.println("未找到该学院的信息!"); } 帮我写出相关的类和构造方法 }
以下是相关的类和构造方法:
1. College类:
public class College {
private String name;
private List<ClassInfo> classes;
private Map<String, Student> students;
public College(String name, List<ClassInfo> classes, Map<String, Student> students) {
this.name = name;
this.classes = classes;
this.students = students;
}
public String getName() {
return name;
}
public List<ClassInfo> getAllClasses() {
return classes;
}
public List<Student> getStudentsByCollege() {
return new ArrayList<>(students.values());
}
public void addStudent(Student student) {
this.students.put(student.getId(), student);
}
}
2. ClassInfo类:
public class ClassInfo {
private String name;
private List<Student> students;
public ClassInfo(String name, List<Student> students) {
this.name = name;
this.students = students;
}
public String getName() {
return name;
}
public List<Student> getStudents() {
return students;
}
}
3. Student类:
public class Student {
private String id;
private String name;
private int age;
public Student(String id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
在调用该方法之前,需要先创建相应的College、ClassInfo和Student对象,并将它们组织成相应的数据结构,然后将这些对象存储在一个Map中。调用该方法时,将这个Map作为参数传入即可。
根据以下这段话写出相对应的代码文件夹中有3个类文件(.java文件),Student.java StudentUtils.java StudentManager.java 学生管理系统 1.学生实体用Student类,包含学生学号,姓名,家庭住址,电话号码 2.学生操作用StudentUtils类,包含学生信息的新增,删除,修改,查询,保存 -每次新增学生时,要将学生信息保存到文件中 -每次删除学生时,要将相应学生信息从文件中删除 -每次修改学生时,要将相应学生信息在文件中修改 -学生信息保存在StudentInfo.txt中 3.学生管理用StudentManager类 当程序运行后,出现菜单供使用者选择 新增学生信息, 删除学生信息, 修改学生信息, 查询学生信息。
以下是三个类的代码实现:
Student.java
```java
public class Student {
private String id;
private String name;
private String address;
private String phone;
public Student(String id, String name, String address, String phone) {
this.id = id;
this.name = name;
this.address = address;
this.phone = phone;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public String getAddress() {
return address;
}
public String getPhone() {
return phone;
}
public void setId(String id) {
this.id = id;
}
public void setName(String name) {
this.name = name;
}
public void setAddress(String address) {
this.address = address;
}
public void setPhone(String phone) {
this.phone = phone;
}
}
```
StudentUtils.java
```java
import java.io.*;
import java.util.ArrayList;
public class StudentUtils {
private static String fileName = "StudentInfo.txt";
public static void addStudent(Student student) {
try {
BufferedWriter writer = new BufferedWriter(new FileWriter(fileName, true));
writer.write(student.getId() + "," + student.getName() + "," + student.getAddress() + "," + student.getPhone());
writer.newLine();
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void deleteStudent(String id) {
try {
BufferedReader reader = new BufferedReader(new FileReader(fileName));
ArrayList<String> lines = new ArrayList<>();
String line;
while ((line = reader.readLine()) != null) {
if (!line.startsWith(id + ",")) {
lines.add(line);
}
}
reader.close();
BufferedWriter writer = new BufferedWriter(new FileWriter(fileName));
for (String l : lines) {
writer.write(l);
writer.newLine();
}
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void modifyStudent(String id, Student newStudent) {
try {
BufferedReader reader = new BufferedReader(new FileReader(fileName));
ArrayList<String> lines = new ArrayList<>();
String line;
while ((line = reader.readLine()) != null) {
if (line.startsWith(id + ",")) {
line = newStudent.getId() + "," + newStudent.getName() + "," + newStudent.getAddress() + "," + newStudent.getPhone();
}
lines.add(line);
}
reader.close();
BufferedWriter writer = new BufferedWriter(new FileWriter(fileName));
for (String l : lines) {
writer.write(l);
writer.newLine();
}
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public static Student queryStudent(String id) {
try {
BufferedReader reader = new BufferedReader(new FileReader(fileName));
String line;
while ((line = reader.readLine()) != null) {
if (line.startsWith(id + ",")) {
String[] fields = line.split(",");
return new Student(fields[0], fields[1], fields[2], fields[3]);
}
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
}
```
StudentManager.java
```java
import java.util.Scanner;
public class StudentManager {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("请选择操作:");
System.out.println("1.新增学生信息");
System.out.println("2.删除学生信息");
System.out.println("3.修改学生信息");
System.out.println("4.查询学生信息");
System.out.println("0.退出程序");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
System.out.print("请输入学生学号:");
String id = scanner.nextLine();
System.out.print("请输入学生姓名:");
String name = scanner.nextLine();
System.out.print("请输入学生家庭住址:");
String address = scanner.nextLine();
System.out.print("请输入学生电话号码:");
String phone = scanner.nextLine();
Student newStudent = new Student(id, name, address, phone);
StudentUtils.addStudent(newStudent);
System.out.println("新增学生信息成功!");
break;
case 2:
System.out.print("请输入要删除的学生学号:");
id = scanner.nextLine();
StudentUtils.deleteStudent(id);
System.out.println("删除学生信息成功!");
break;
case 3:
System.out.print("请输入要修改的学生学号:");
id = scanner.nextLine();
Student student = StudentUtils.queryStudent(id);
if (student != null) {
System.out.print("请输入新的学生学号:");
String newId = scanner.nextLine();
System.out.print("请输入新的学生姓名:");
name = scanner.nextLine();
System.out.print("请输入新的学生家庭住址:");
address = scanner.nextLine();
System.out.print("请输入新的学生电话号码:");
phone = scanner.nextLine();
newStudent = new Student(newId, name, address, phone);
StudentUtils.modifyStudent(id, newStudent);
System.out.println("修改学生信息成功!");
} else {
System.out.println("学生信息不存在!");
}
break;
case 4:
System.out.print("请输入要查询的学生学号:");
id = scanner.nextLine();
student = StudentUtils.queryStudent(id);
if (student != null) {
System.out.println("学号:" + student.getId());
System.out.println("姓名:" + student.getName());
System.out.println("家庭住址:" + student.getAddress());
System.out.println("电话号码:" + student.getPhone());
} else {
System.out.println("学生信息不存在!");
}
break;
case 0:
System.out.println("程序已退出。");
System.exit(0);
break;
default:
System.out.println("请输入正确的操作编号!");
break;
}
}
}
}
```
阅读全文
相关推荐
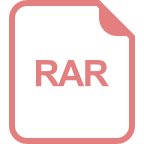
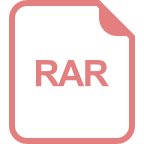
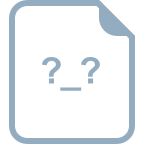













