package com.de.debook.utils; import java.io.*; import java.text.SimpleDateFormat; import java.util.Date; import java.util.UUID; public class FileUploadUtils { /** * @param * @description: 生成一个唯一的ID * @return: java.lang.String */ public static String createUUID() { String s = UUID.randomUUID().toString(); String s2 = s.substring(24).replace("-", ""); return s2.toUpperCase(); } /** * @description: 得到一个保证不重复的临时文件名 * @return: java.lang.String */ public static String createTmpFileName(String suffix) { SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd-HHmmss"); String datestr = sdf.format(new Date()); String name = datestr + "-" + createUUID() + "." + suffix; return name; } /** * @param fileName 原始文件名 * @description: 得到文件的后缀名 * @return: java.lang.String */ public static String fileSuffix(String fileName) { int p = fileName.lastIndexOf('.'); if (p >= 0) { return fileName.substring(p + 1).toLowerCase(); } return ""; } public static void deleteDir(String dirPath) { File file = new File(dirPath); if (file.isFile()) { file.delete(); } else { File[] files = file.listFiles(); if (files == null) { file.delete(); } else { for (int i = 0; i < files.length; i++) { deleteDir(files[i].getAbsolutePath()); } file.delete(); } } } public static byte[] getFileByteArray(File file) { long fileSize = file.length(); if (fileSize > Integer.MAX_VALUE) { System.out.println("file too big..."); return null; } byte[] buffer = null; try (FileInputStream fi = new FileInputStream(file)) { buffer = new byte[(int) fileSize]; int offset = 0; int numRead = 0; while (offset < buffer.length && (numRead = fi.read(buffer, offset, buffer.length - offset)) >= 0) { offset += numRead; } // 确保所有数据均被读取 if (offset != buffer.length) { throw new IOException("Could not completely read file" + file.getName()); } } catch (Exception e) { e.printStackTrace(); } return buffer; } public static void writeBytesToFile(byte[] bs, String file) throws IOException { OutputStream out = new FileOutputStream(file); InputStream is = new ByteArrayInputStream(bs); byte[] buff = new byte[1024]; int len = 0; while ((len = is.read(buff)) != -1) { out.write(buff, 0, len); } is.close(); out.close(); } }
时间: 2023-12-25 13:06:25 浏览: 36
这是一个文件上传工具类,包含以下方法:
1. createUUID():用于生成唯一的UUID。
2. createTmpFileName(String suffix):用于生成一个不重复的临时文件名,包括时间戳和UUID。
3. fileSuffix(String fileName):用于获取文件的后缀名。
4. deleteDir(String dirPath):用于删除指定目录下的所有文件和子目录。
5. getFileByteArray(File file):用于将文件转换成byte数组。
6. writeBytesToFile(byte[] bs, String file):用于将byte数组写入指定的文件中。
这些方法可以帮助我们实现文件上传功能。需要注意的是,该工具类中的一些方法可能会抛出IOException异常,需要在调用时进行处理。
相关问题
package com.de.debook.init; import com.de.debook.constant.WebConstant; import org.springframework.boot.CommandLineRunner; import org.springframework.stereotype.Component; import org.springframework.util.ClassUtils; @Component public class InitConfig implements CommandLineRunner { @Override public void run(String... strings) throws Exception { // 项目路径 WebConstant.WEB_FILE_ROOT = ClassUtils.getDefaultClassLoader() .getResource("").getPath().substring(1); // 图片存储目录 WebConstant.FILE_STORAGE_ROOT = WebConstant.WEB_FILE_ROOT + "static/images/"; } }
这是一个Spring Boot项目的初始化配置类,实现了CommandLineRunner接口,用于在Spring Boot应用程序启动时执行一些初始化操作。
具体来说,该配置类的run方法实现了以下两个操作:
1. 获取项目路径:通过ClassUtils.getDefaultClassLoader().getResource("").getPath()方法获取当前类所在的classpath路径,然后通过substring(1)方法去掉开头的“/”字符,得到项目的根路径。
2. 设置图片存储目录:将WebConstant.WEB_FILE_ROOT和"static/images/"拼接起来,得到图片存储目录的绝对路径,保存到WebConstant.FILE_STORAGE_ROOT中。
这里的WebConstant是一个常量类,用于保存项目中的常量值。通过这个配置类,我们可以方便地获取项目路径和设置图片存储目录,从而方便地进行文件操作。
package com.de.debook.controller; import com.de.debook.bo.ResponseBean; import com.de.debook.constant.WebConstant; import com.de.debook.utils.FileUploadUtils; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController; import org.springframework.web.multipart.MultipartFile; import java.io.File; import java.io.IOException; import java.util.Map; @RestController public class UploadFileController { private static final int FILE_SIZE_MAX = 20 * 1024 * 1024; // 上传限制大小 /** * @param multipartFile * @description: 通用文件上传处理器 * @return: java.util.Map<java.lang.String , java.lang.Object> */ @RequestMapping(value = "/uploadFile", produces = "application/json;charset=UTF-8") public Map<String, Object> fileUpload(@RequestParam("file") MultipartFile multipartFile) { ResponseBean responseBean = new ResponseBean(); if (multipartFile != null) { String realName = multipartFile.getOriginalFilename(); // 原始文件名 String suffix = FileUploadUtils.fileSuffix(realName); // 文件名后缀 String tmpFileName = FileUploadUtils.createTmpFileName(suffix); // 生成保证不重复的临时文件名 if (multipartFile.getSize() > FILE_SIZE_MAX) { responseBean.putError("上传失败:文件大小不得超过20MB"); return responseBean.getResponseMap(); } File tmpFile = new File(WebConstant.FILE_STORAGE_ROOT,tmpFileName); try { multipartFile.transferTo(tmpFile); // 写入本地 responseBean.putData("data", "/images/" + tmpFileName); } catch (IOException e) { responseBean.putError("上传失败:" + e.getMessage()); e.printStackTrace(); } } return responseBean.getResponseMap(); } }
这是一个基于Spring框架开发的文件上传处理器,它接收一个MultipartFile类型的参数,即上传的文件,然后进行处理,最终返回一个包含上传结果的ResponseBean对象。其中,文件大小限制为20MB,超过限制则返回上传失败的信息;上传成功后,会将文件写入到本地,并将其访问路径存储到ResponseBean中返回。
相关推荐
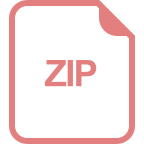













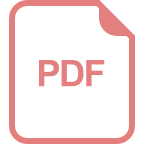