(1) Event unterminated_case: The case for value "'F'" is not terminated by a "break" statement. Also see events: [fallthrough]
时间: 2024-03-19 17:41:28 浏览: 16
这是一个编程错误,表示在一个 switch 语句中,当匹配到某个 case 分支时,没有使用 break 语句来终止该分支的执行,导致代码会继续执行下面的分支,这可能会引起意想不到的结果。解决这个问题的方法是在每个 case 分支的最后添加一个 break 语句来终止该分支的执行。如果需要让多个分支共享一些代码,可以使用 fallthrough 关键字来实现。
相关问题
json.decoder.JSONDecodeError: Unterminated string starting at: line 1 column 1822 (char 1821)
.decoder.JSONDecodeError: Unterminated string starting at: line 1 column 1822 (char 1821)是指在解析JSON格式的数据时,发现在第1行第1822个字符处出现了未结束的字符串。这通常是由于JSON数据格式错误导致的。解决此问题的方法是检查JSON数据格式是否正确,特别是检查字符串是否正确结束。
以下是解决此问题的一些方法:
```python
# 读取JSON文件
import json
with open('data.json', 'r') as f:
try:
data = json.load(f)
except json.decoder.JSONDecodeError as e:
print("JSONDecodeError: ", e)
```
```python
# 解析JSON字符串
import json
json_str = '{"name": "John", "age": 30, "city": "New York"}'
try:
data = json.loads(json_str)
except json.decoder.JSONDecodeError as e:
print("JSONDecodeError: ", e)
```
```python
# 检查JSON数据格式是否正确
import json
json_str = '{"name": "John", "age": 30, "city": "New York"'
try:
data = json.loads(json_str)
except json.decoder.JSONDecodeError as e:
print("JSONDecodeError: ", e)
# 输出:JSONDecodeError: Expecting property name enclosed in double quotes: line 1 column 28 (char 27)
```
D:\123\untitled\mainwindow.h:1: error: unterminated #ifndef #ifndef MAINWINDOW_H ^
这个错误是由于您的代码中的 #ifndef 预处理指令没有相应的 #endif 配对引起的。#ifndef 和 #endif 通常是成对出现的,用于条件编译,以防止头文件的重复包含。
要解决这个错误,您需要确保在头文件中添加相应的 #endif,来配对之前的 #ifndef。例如,您的代码应该类似于以下形式:
```cpp
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
// 头文件内容
#endif // MAINWINDOW_H
```
请检查您的代码,确保在头文件的开头有一个 #ifndef 指令,并在文件的结尾处添加相应的 #endif 指令。这样可以解决 "unterminated #ifndef" 错误。
如果问题仍然存在,请提供更多相关的代码和错误信息以便我更好地帮助您解决问题。
相关推荐
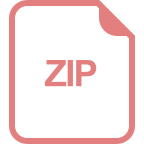
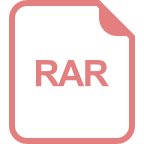
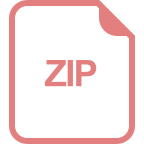












