JDBC连接mysql数据库获取数据并生成Txt输出
时间: 2023-08-02 15:41:26 浏览: 52
好的,我可以为您提供Java代码示例,实现JDBC连接MySQL数据库,获取数据并生成Txt输出。
首先,您需要确保已经安装了MySQL数据库并创建了一张表,用于存储您需要获取的数据。假设您的表名为`user`,包含两个字段`id`和`name`。
接下来,您需要在Java项目中导入MySQL JDBC驱动程序的jar包,以便连接MySQL数据库。
示例代码如下:
```java
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.sql.*;
public class JDBCExample {
// JDBC连接的URL,其中`test`为数据库名,`root`为用户名,`password`为密码,根据实际情况修改
private static final String URL = "jdbc:mysql://localhost:3306/test?useSSL=false&serverTimezone=UTC";
private static final String USER = "root";
private static final String PASSWORD = "123456";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 注册JDBC驱动程序
Class.forName("com.mysql.cj.jdbc.Driver");
// 打开连接
conn = DriverManager.getConnection(URL, USER, PASSWORD);
// 执行查询
stmt = conn.createStatement();
rs = stmt.executeQuery("SELECT * FROM user");
// 写入数据
BufferedWriter writer = new BufferedWriter(new FileWriter("data.txt"));
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
writer.write("id: " + id + ", name: " + name + "\n");
}
writer.close();
System.out.println("数据写入成功!");
} catch (ClassNotFoundException | SQLException | IOException e) {
e.printStackTrace();
} finally {
// 关闭连接
try {
if (rs != null) rs.close();
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
在上面的代码中,我们首先注册了MySQL JDBC驱动程序,然后打开了一个连接。接着,我们执行了一个查询语句,将结果集写入到`data.txt`文件中,并在控制台输出成功信息。最后,我们关闭了连接。
您需要根据实际情况修改`URL`、`USER`和`PASSWORD`参数,以及查询语句和写入数据的方式。
相关推荐
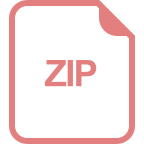
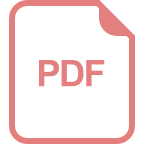
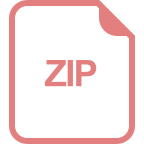














