dcache.c中的d_hash函数和dentry操作中的d_hash之间的调用路径展示一下
时间: 2024-04-29 17:23:56 浏览: 27
1. 在dcache.c中定义了d_hash函数,该函数用于计算给定dentry的哈希值:
```c
unsigned int d_hash(const struct dentry *dentry, const struct qstr *name)
{
return full_name_hash(name->name, name->len);
}
```
2. 在dentry操作中,需要将dentry插入到dentry缓存中,因此需要调用d_hash函数来计算dentry的哈希值。这个过程发生在d_alloc和d_instantiate函数中。
```c
struct dentry *d_alloc(struct dentry *parent, const struct qstr *name)
{
struct dentry *dentry;
unsigned int len = name->len;
unsigned int hash = d_hash(parent, name); // 调用d_hash计算哈希值
// 分配dentry对象并初始化
dentry = __d_alloc(parent->d_sb, len);
dentry->d_name = *name;
dentry->d_parent = parent;
dentry->d_flags |= DCACHE_UNHASHED;
return dentry;
}
void d_instantiate(struct dentry *entry, struct inode *inode)
{
spin_lock(&dcache_lock);
// 设置dentry的inode和哈希值
entry->d_inode = inode;
entry->d_flags &= ~DCACHE_UNHASHED;
entry->d_hash = inode->i_ino; // inode的编号作为哈希值
// 将dentry插入到哈希表中
d_hash_and_lookup(entry, NULL);
spin_unlock(&dcache_lock);
}
```
3. 在d_instantiate函数中,还调用了d_hash_and_lookup函数来将dentry插入到哈希表中。
```c
static inline void d_hash_and_lookup(struct dentry *entry, struct qstr *str)
{
struct hlist_head *head = dentry_hash(entry->d_sb, entry->d_hash);
spin_lock(&dcache_lock);
// 将dentry插入到哈希表中
hlist_add_head(&entry->d_hash, head);
spin_unlock(&dcache_lock);
}
```
4. d_hash_and_lookup函数中调用了dentry_hash函数来获取哈希表中对应哈希值的链表头。
```c
static inline struct hlist_head *dentry_hash(const struct super_block *sb, unsigned long hashval)
{
return &sb->s_dentry_hashtable[hashval & (sb->s_dentry_hashtable_size - 1)];
}
```
5. 在dentry缓存中查找dentry时,也需要调用d_hash函数来计算dentry的哈希值。这个过程发生在d_lookup函数中。
```c
struct dentry *d_lookup(const struct dentry * parent, const struct qstr * name)
{
struct dentry *dentry;
unsigned int len = name->len;
unsigned int hash = d_hash(parent, name); // 调用d_hash计算哈希值
spin_lock(&dcache_lock);
// 在哈希表中查找dentry
dentry = __d_lookup(parent, name, hash);
spin_unlock(&dcache_lock);
return dentry;
}
```
6. 在__d_lookup函数中,也需要调用dentry_hash函数来获取哈希表中对应哈希值的链表头。
```c
static struct dentry *__d_lookup(const struct dentry * parent, const struct qstr * name, unsigned int hash)
{
struct dentry *dentry;
struct hlist_head *head = dentry_hash(parent->d_sb, hash); // 调用dentry_hash获取哈希表中对应的链表头
// 遍历链表查找dentry
hlist_for_each_entry(dentry, head, d_hash)
{
if (dentry->d_parent == parent && dentry->d_name.hash == hash && dentry_cmp(dentry, name) == 0)
return dentry;
}
return NULL;
}
```
相关推荐
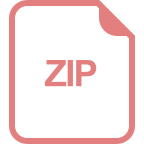














