NFC Android studio
时间: 2024-01-21 20:44:42 浏览: 133
NFC(Near Field Communication)是一种短距离无线通信技术,它允许设备在非接触模式下进行通信。在Android中,可以使用NFC API来读取和写入NFC标签,以及与其他NFC设备进行通信。
以下是在Android Studio中使用NFC API的基本步骤:
1. 在AndroidManifest.xml文件中添加以下权限:
```
<uses-permission android:name="android.permission.NFC" />
```
2. 在布局文件中添加NFC相关组件,例如NFC标签扫描区域:
```
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Scan NFC tag" />
<LinearLayout
android:id="@+id/nfc_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:visibility="gone">
<TextView
android:id="@+id/tag_content"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Tag Content" />
<TextView
android:id="@+id/tag_tech_list"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Tag Technology List" />
</LinearLayout>
```
3. 在Activity中初始化NFC相关组件,并注册NFC事件:
```
public class MainActivity extends AppCompatActivity implements NfcAdapter.ReaderCallback {
private NfcAdapter nfcAdapter;
private TextView textView;
private LinearLayout nfcLayout;
private TextView tagContent;
private TextView tagTechList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView = findViewById(R.id.textView);
nfcLayout = findViewById(R.id.nfc_layout);
tagContent = findViewById(R.id.tag_content);
tagTechList = findViewById(R.id.tag_tech_list);
nfcAdapter = NfcAdapter.getDefaultAdapter(this);
if (nfcAdapter == null) {
textView.setText("NFC is not available on this device.");
}
}
@Override
protected void onResume() {
super.onResume();
if (nfcAdapter != null) {
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, new Intent(this, getClass()).addFlags(Intent.FLAG_ACTIVITY_SINGLE_TOP), 0);
nfcAdapter.enableForegroundDispatch(this, pendingIntent, null, null);
}
}
@Override
protected void onPause() {
super.onPause();
if (nfcAdapter != null) {
nfcAdapter.disableForegroundDispatch(this);
}
}
@Override
public void onNewIntent(Intent intent) {
super.onNewIntent(intent);
if (NfcAdapter.ACTION_TAG_DISCOVERED.equals(intent.getAction())) {
Tag tag = intent.getParcelableExtra(NfcAdapter.EXTRA_TAG);
byte[] tagId = tag.getId();
// Read tag content and technology list
String tagContentText = "";
String tagTechListText = "";
for (String tech : tag.getTechList()) {
tagTechListText += tech + "\n";
if (tech.equals(Ndef.class.getName())) {
Ndef ndef = Ndef.get(tag);
try {
ndef.connect();
NdefMessage ndefMessage = ndef.getNdefMessage();
if (ndefMessage != null) {
tagContentText += new String(ndefMessage.getRecords()[0].getPayload());
}
} catch (IOException | FormatException e) {
e.printStackTrace();
} finally {
try {
ndef.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
// Update UI with tag content and technology list
tagContent.setText(tagContentText);
tagTechList.setText(tagTechListText);
nfcLayout.setVisibility(View.VISIBLE);
textView.setVisibility(View.GONE);
}
}
@Override
public void onTagDiscovered(Tag tag) {
// This method is deprecated and should not be used
}
}
```
在onResume()方法和onPause()方法中启用和禁用前台调度,以便在应用程序处于前台时处理NFC事件。在onNewIntent()方法中处理NFC标签扫描事件,并在UI中显示标签内容和技术列表。
4. 运行应用程序,并将NFC标签放在设备的NFC扫描区域上。如果一切正常,应该会显示标签内容和技术列表。
这是一个简单的NFC应用程序示例,您可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
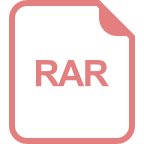
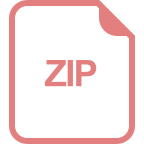
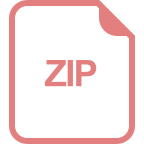
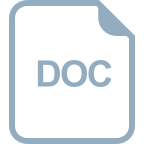










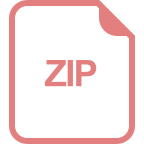
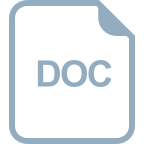